mirror of
https://codeberg.org/forgejo/forgejo.git
synced 2024-11-01 15:19:09 +01:00
1. Fix multiple error display for math and mermaid: 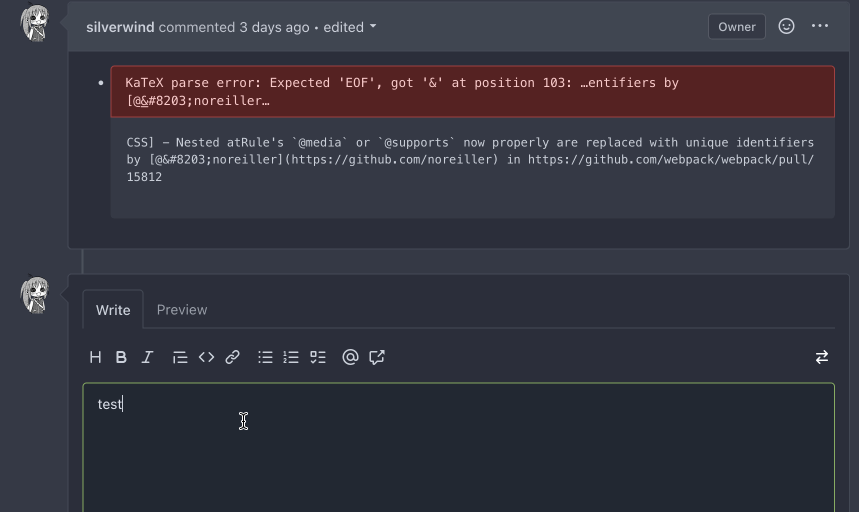 2. Fix height calculation of certain mermaid diagrams by reading the iframe inner height from it's document instead of parsing it from SVG: Before: <img width="866" alt="Screenshot 2023-04-11 at 11 56 27" src="https://user-images.githubusercontent.com/115237/231126480-b194e02b-ea8c-4ddf-8c79-50c525815d92.png"> After: <img width="855" alt="Screenshot 2023-04-11 at 11 56 35" src="https://user-images.githubusercontent.com/115237/231126494-5fe86a48-8d21-455a-8b95-79b6ee27a16f.png"> 3. Refactor error handling to a common function 4. Rename to `renderAsciicast` for consistency 5. Improve mermaid loading sequence Note: I did try `securityLevel: 'sandbox'` to make mermaid output a iframe directly, but that showed a bug in mermaid where the iframe style height was set incorrectly. Opened https://github.com/mermaid-js/mermaid/issues/4289 for this. --------- Co-authored-by: Giteabot <teabot@gitea.io>
72 lines
2.4 KiB
JavaScript
72 lines
2.4 KiB
JavaScript
import {isDarkTheme} from '../utils.js';
|
|
import {makeCodeCopyButton} from './codecopy.js';
|
|
import {displayError} from './common.js';
|
|
|
|
const {mermaidMaxSourceCharacters} = window.config;
|
|
|
|
const iframeCss = `:root {color-scheme: normal}
|
|
body {margin: 0; padding: 0; overflow: hidden}
|
|
#mermaid {display: block; margin: 0 auto}`;
|
|
|
|
export async function renderMermaid() {
|
|
const els = document.querySelectorAll('.markup code.language-mermaid');
|
|
if (!els.length) return;
|
|
|
|
const {default: mermaid} = await import(/* webpackChunkName: "mermaid" */'mermaid');
|
|
|
|
mermaid.initialize({
|
|
startOnLoad: false,
|
|
theme: isDarkTheme() ? 'dark' : 'neutral',
|
|
securityLevel: 'strict',
|
|
});
|
|
|
|
for (const el of els) {
|
|
const pre = el.closest('pre');
|
|
if (pre.hasAttribute('data-render-done')) continue;
|
|
|
|
const source = el.textContent;
|
|
if (mermaidMaxSourceCharacters >= 0 && source.length > mermaidMaxSourceCharacters) {
|
|
displayError(pre, new Error(`Mermaid source of ${source.length} characters exceeds the maximum allowed length of ${mermaidMaxSourceCharacters}.`));
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
await mermaid.parse(source);
|
|
} catch (err) {
|
|
displayError(pre, err);
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
// can't use bindFunctions here because we can't cross the iframe boundary. This
|
|
// means js-based interactions won't work but they aren't intended to work either
|
|
const {svg} = await mermaid.render('mermaid', source);
|
|
|
|
const iframe = document.createElement('iframe');
|
|
iframe.classList.add('markup-render', 'gt-invisible');
|
|
iframe.srcdoc = `<html><head><style>${iframeCss}</style></head><body>${svg}</body></html>`;
|
|
|
|
const mermaidBlock = document.createElement('div');
|
|
mermaidBlock.classList.add('mermaid-block', 'is-loading', 'gt-hidden');
|
|
mermaidBlock.append(iframe);
|
|
|
|
const btn = makeCodeCopyButton();
|
|
btn.setAttribute('data-clipboard-text', source);
|
|
mermaidBlock.append(btn);
|
|
|
|
iframe.addEventListener('load', () => {
|
|
pre.replaceWith(mermaidBlock);
|
|
mermaidBlock.classList.remove('gt-hidden');
|
|
iframe.style.height = `${iframe.contentWindow.document.body.clientHeight}px`;
|
|
setTimeout(() => { // avoid flash of iframe background
|
|
mermaidBlock.classList.remove('is-loading');
|
|
iframe.classList.remove('gt-invisible');
|
|
}, 0);
|
|
});
|
|
|
|
document.body.append(mermaidBlock);
|
|
} catch (err) {
|
|
displayError(pre, err);
|
|
}
|
|
}
|
|
}
|