[SIEM] Fixes "include building block button" to operate (#73900)
## Summary Blocker fixes "include building block button" to operate when there is no data on the table. Before if you had nothing on the table then the button would not operate as it would not cause a re-render: 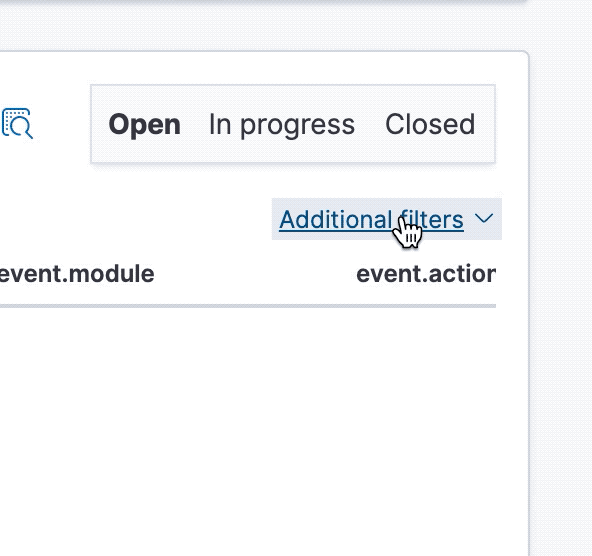 After where the button now works: 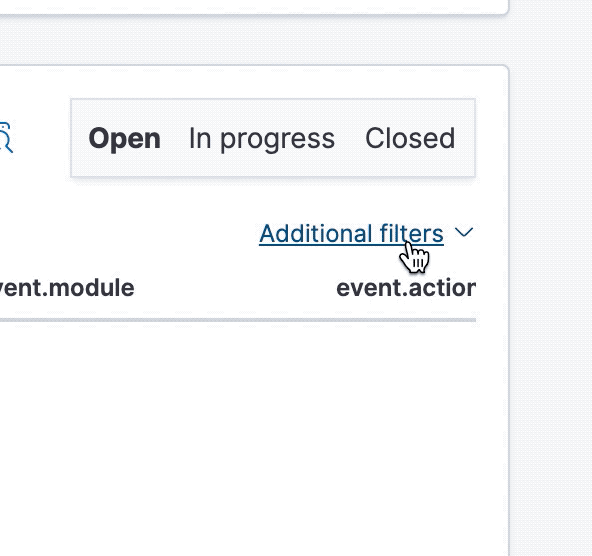 This wasn't caught because most people have something already on the table which makes the rendering render and just work. Simple one line low level fix. ### Checklist Delete any items that are not applicable to this PR. - [ ] [Unit or functional tests](https://github.com/elastic/kibana/blob/master/CONTRIBUTING.md#cross-browser-compatibility) were updated or added to match the most common scenarios No tests for this file at the moment and we need this as a fast backport to make the release cut off.
This commit is contained in:
parent
aa668db796
commit
0a5427842b
|
@ -5,14 +5,15 @@
|
|||
*/
|
||||
|
||||
import React from 'react';
|
||||
import { shallow } from 'enzyme';
|
||||
import { shallow, mount } from 'enzyme';
|
||||
|
||||
import { AlertsUtilityBar } from './index';
|
||||
import { AlertsUtilityBar, AlertsUtilityBarProps } from './index';
|
||||
import { TestProviders } from '../../../../common/mock/test_providers';
|
||||
|
||||
jest.mock('../../../../common/lib/kibana');
|
||||
|
||||
describe('AlertsUtilityBar', () => {
|
||||
it('renders correctly', () => {
|
||||
test('renders correctly', () => {
|
||||
const wrapper = shallow(
|
||||
<AlertsUtilityBar
|
||||
canUserCRUD={true}
|
||||
|
@ -32,4 +33,185 @@ describe('AlertsUtilityBar', () => {
|
|||
|
||||
expect(wrapper.find('[dataTestSubj="alertActionPopover"]')).toBeTruthy();
|
||||
});
|
||||
|
||||
describe('UtilityBarAdditionalFiltersContent', () => {
|
||||
test('does not show the showBuildingBlockAlerts checked if the showBuildingBlockAlerts is false', () => {
|
||||
const onShowBuildingBlockAlertsChanged = jest.fn();
|
||||
const wrapper = mount(
|
||||
<TestProviders>
|
||||
<AlertsUtilityBar
|
||||
canUserCRUD={true}
|
||||
hasIndexWrite={true}
|
||||
areEventsLoading={false}
|
||||
clearSelection={jest.fn()}
|
||||
totalCount={100}
|
||||
selectedEventIds={{}}
|
||||
currentFilter="closed"
|
||||
selectAll={jest.fn()}
|
||||
showClearSelection={true}
|
||||
showBuildingBlockAlerts={false} // Does not show showBuildingBlockAlerts checked if this is false
|
||||
onShowBuildingBlockAlertsChanged={onShowBuildingBlockAlertsChanged}
|
||||
updateAlertsStatus={jest.fn()}
|
||||
/>
|
||||
</TestProviders>
|
||||
);
|
||||
// click the filters button to popup the checkbox to make it visible
|
||||
wrapper
|
||||
.find('[data-test-subj="additionalFilters"] button')
|
||||
.first()
|
||||
.simulate('click')
|
||||
.update();
|
||||
|
||||
// The check box should be false
|
||||
expect(
|
||||
wrapper
|
||||
.find('[data-test-subj="showBuildingBlockAlertsCheckbox"] input')
|
||||
.first()
|
||||
.prop('checked')
|
||||
).toEqual(false);
|
||||
});
|
||||
|
||||
test('does show the showBuildingBlockAlerts checked if the showBuildingBlockAlerts is true', () => {
|
||||
const onShowBuildingBlockAlertsChanged = jest.fn();
|
||||
const wrapper = mount(
|
||||
<TestProviders>
|
||||
<AlertsUtilityBar
|
||||
canUserCRUD={true}
|
||||
hasIndexWrite={true}
|
||||
areEventsLoading={false}
|
||||
clearSelection={jest.fn()}
|
||||
totalCount={100}
|
||||
selectedEventIds={{}}
|
||||
currentFilter="closed"
|
||||
selectAll={jest.fn()}
|
||||
showClearSelection={true}
|
||||
showBuildingBlockAlerts={true} // Does show showBuildingBlockAlerts checked if this is true
|
||||
onShowBuildingBlockAlertsChanged={onShowBuildingBlockAlertsChanged}
|
||||
updateAlertsStatus={jest.fn()}
|
||||
/>
|
||||
</TestProviders>
|
||||
);
|
||||
// click the filters button to popup the checkbox to make it visible
|
||||
wrapper
|
||||
.find('[data-test-subj="additionalFilters"] button')
|
||||
.first()
|
||||
.simulate('click')
|
||||
.update();
|
||||
|
||||
// The check box should be true
|
||||
expect(
|
||||
wrapper
|
||||
.find('[data-test-subj="showBuildingBlockAlertsCheckbox"] input')
|
||||
.first()
|
||||
.prop('checked')
|
||||
).toEqual(true);
|
||||
});
|
||||
|
||||
test('calls the onShowBuildingBlockAlertsChanged when the check box is clicked', () => {
|
||||
const onShowBuildingBlockAlertsChanged = jest.fn();
|
||||
const wrapper = mount(
|
||||
<TestProviders>
|
||||
<AlertsUtilityBar
|
||||
canUserCRUD={true}
|
||||
hasIndexWrite={true}
|
||||
areEventsLoading={false}
|
||||
clearSelection={jest.fn()}
|
||||
totalCount={100}
|
||||
selectedEventIds={{}}
|
||||
currentFilter="closed"
|
||||
selectAll={jest.fn()}
|
||||
showClearSelection={true}
|
||||
showBuildingBlockAlerts={false}
|
||||
onShowBuildingBlockAlertsChanged={onShowBuildingBlockAlertsChanged}
|
||||
updateAlertsStatus={jest.fn()}
|
||||
/>
|
||||
</TestProviders>
|
||||
);
|
||||
// click the filters button to popup the checkbox to make it visible
|
||||
wrapper
|
||||
.find('[data-test-subj="additionalFilters"] button')
|
||||
.first()
|
||||
.simulate('click')
|
||||
.update();
|
||||
|
||||
// check the box
|
||||
wrapper
|
||||
.find('[data-test-subj="showBuildingBlockAlertsCheckbox"] input')
|
||||
.first()
|
||||
.simulate('change', { target: { checked: true } });
|
||||
|
||||
// Make sure our callback is called
|
||||
expect(onShowBuildingBlockAlertsChanged).toHaveBeenCalled();
|
||||
});
|
||||
|
||||
test('can update showBuildingBlockAlerts from false to true', () => {
|
||||
const Proxy = (props: AlertsUtilityBarProps) => (
|
||||
<TestProviders>
|
||||
<AlertsUtilityBar
|
||||
canUserCRUD={true}
|
||||
hasIndexWrite={true}
|
||||
areEventsLoading={false}
|
||||
clearSelection={jest.fn()}
|
||||
totalCount={100}
|
||||
selectedEventIds={{}}
|
||||
currentFilter="closed"
|
||||
selectAll={jest.fn()}
|
||||
showClearSelection={true}
|
||||
showBuildingBlockAlerts={props.showBuildingBlockAlerts}
|
||||
onShowBuildingBlockAlertsChanged={jest.fn()}
|
||||
updateAlertsStatus={jest.fn()}
|
||||
/>
|
||||
</TestProviders>
|
||||
);
|
||||
|
||||
const wrapper = mount(
|
||||
<Proxy
|
||||
canUserCRUD={true}
|
||||
hasIndexWrite={true}
|
||||
areEventsLoading={false}
|
||||
clearSelection={jest.fn()}
|
||||
totalCount={100}
|
||||
selectedEventIds={{}}
|
||||
currentFilter="closed"
|
||||
selectAll={jest.fn()}
|
||||
showClearSelection={true}
|
||||
showBuildingBlockAlerts={false}
|
||||
onShowBuildingBlockAlertsChanged={jest.fn()}
|
||||
updateAlertsStatus={jest.fn()}
|
||||
/>
|
||||
);
|
||||
// click the filters button to popup the checkbox to make it visible
|
||||
wrapper
|
||||
.find('[data-test-subj="additionalFilters"] button')
|
||||
.first()
|
||||
.simulate('click')
|
||||
.update();
|
||||
|
||||
// The check box should false now since we initially set the showBuildingBlockAlerts to false
|
||||
expect(
|
||||
wrapper
|
||||
.find('[data-test-subj="showBuildingBlockAlertsCheckbox"] input')
|
||||
.first()
|
||||
.prop('checked')
|
||||
).toEqual(false);
|
||||
|
||||
wrapper.setProps({ showBuildingBlockAlerts: true });
|
||||
wrapper.update();
|
||||
|
||||
// click the filters button to popup the checkbox to make it visible
|
||||
wrapper
|
||||
.find('[data-test-subj="additionalFilters"] button')
|
||||
.first()
|
||||
.simulate('click')
|
||||
.update();
|
||||
|
||||
// The check box should be true now since we changed the showBuildingBlockAlerts from false to true
|
||||
expect(
|
||||
wrapper
|
||||
.find('[data-test-subj="showBuildingBlockAlertsCheckbox"] input')
|
||||
.first()
|
||||
.prop('checked')
|
||||
).toEqual(true);
|
||||
});
|
||||
});
|
||||
});
|
||||
|
|
|
@ -28,7 +28,7 @@ import { TimelineNonEcsData } from '../../../../graphql/types';
|
|||
import { UpdateAlertsStatus } from '../types';
|
||||
import { FILTER_CLOSED, FILTER_IN_PROGRESS, FILTER_OPEN } from '../alerts_filter_group';
|
||||
|
||||
interface AlertsUtilityBarProps {
|
||||
export interface AlertsUtilityBarProps {
|
||||
canUserCRUD: boolean;
|
||||
hasIndexWrite: boolean;
|
||||
areEventsLoading: boolean;
|
||||
|
@ -223,5 +223,6 @@ export const AlertsUtilityBar = React.memo(
|
|||
prevProps.areEventsLoading === nextProps.areEventsLoading &&
|
||||
prevProps.selectedEventIds === nextProps.selectedEventIds &&
|
||||
prevProps.totalCount === nextProps.totalCount &&
|
||||
prevProps.showClearSelection === nextProps.showClearSelection
|
||||
prevProps.showClearSelection === nextProps.showClearSelection &&
|
||||
prevProps.showBuildingBlockAlerts === nextProps.showBuildingBlockAlerts
|
||||
);
|
||||
|
|
Loading…
Reference in a new issue