2020-06-26 22:38:02 +02:00
|
|
|
|
// Copyright (c) Microsoft Corporation.
|
|
|
|
|
// Licensed under the MIT license.
|
|
|
|
|
|
|
|
|
|
#include "pch.h"
|
|
|
|
|
#include "Command.h"
|
|
|
|
|
#include "Command.g.cpp"
|
|
|
|
|
|
|
|
|
|
#include "ActionAndArgs.h"
|
2020-07-17 03:31:09 +02:00
|
|
|
|
#include "JsonUtils.h"
|
2020-06-26 22:38:02 +02:00
|
|
|
|
#include <LibraryResources.h>
|
2020-08-19 19:33:19 +02:00
|
|
|
|
#include "TerminalSettingsSerializationHelpers.h"
|
2020-06-26 22:38:02 +02:00
|
|
|
|
|
2020-10-06 18:56:59 +02:00
|
|
|
|
using namespace winrt::Microsoft::Terminal::Settings::Model;
|
|
|
|
|
using namespace winrt::Windows::Foundation::Collections;
|
|
|
|
|
using namespace ::Microsoft::Terminal::Settings::Model;
|
2020-06-26 22:38:02 +02:00
|
|
|
|
|
2020-08-21 20:08:02 +02:00
|
|
|
|
namespace winrt
|
|
|
|
|
{
|
|
|
|
|
namespace MUX = Microsoft::UI::Xaml;
|
|
|
|
|
namespace WUX = Windows::UI::Xaml;
|
|
|
|
|
}
|
|
|
|
|
|
2020-06-26 22:38:02 +02:00
|
|
|
|
static constexpr std::string_view NameKey{ "name" };
|
2020-08-21 20:08:02 +02:00
|
|
|
|
static constexpr std::string_view IconKey{ "icon" };
|
2020-06-26 22:38:02 +02:00
|
|
|
|
static constexpr std::string_view ActionKey{ "command" };
|
|
|
|
|
static constexpr std::string_view ArgsKey{ "args" };
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
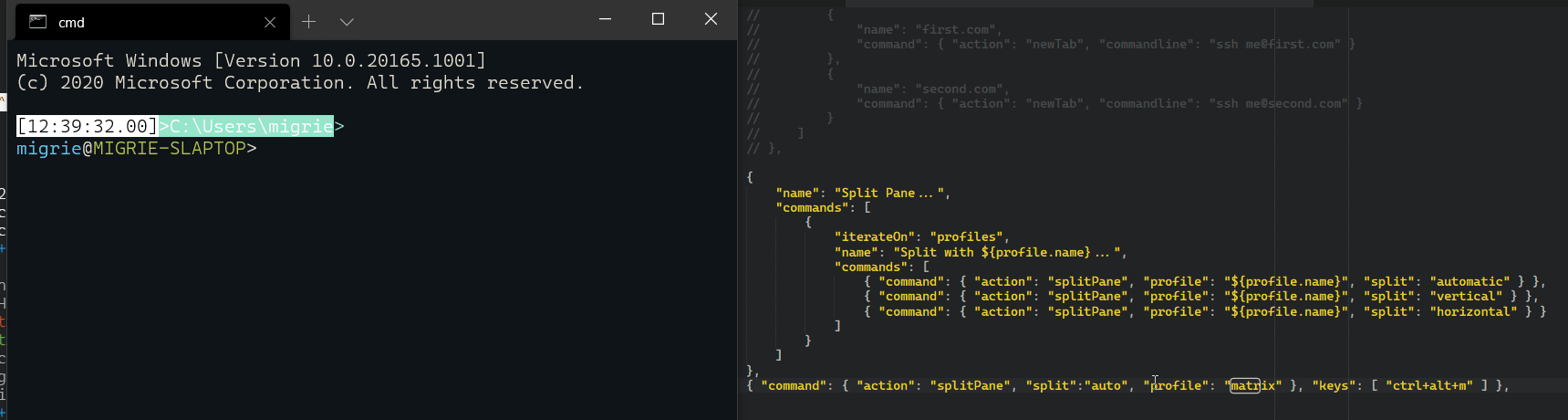
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
static constexpr std::string_view IterateOnKey{ "iterateOn" };
|
|
|
|
|
static constexpr std::string_view CommandsKey{ "commands" };
|
|
|
|
|
|
2020-08-19 19:33:19 +02:00
|
|
|
|
static constexpr std::string_view ProfileNameToken{ "${profile.name}" };
|
2020-08-21 20:08:02 +02:00
|
|
|
|
static constexpr std::string_view ProfileIconToken{ "${profile.icon}" };
|
2020-08-19 19:33:19 +02:00
|
|
|
|
static constexpr std::string_view SchemeNameToken{ "${scheme.name}" };
|
2020-06-26 22:38:02 +02:00
|
|
|
|
|
2020-10-06 18:56:59 +02:00
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Model::implementation
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
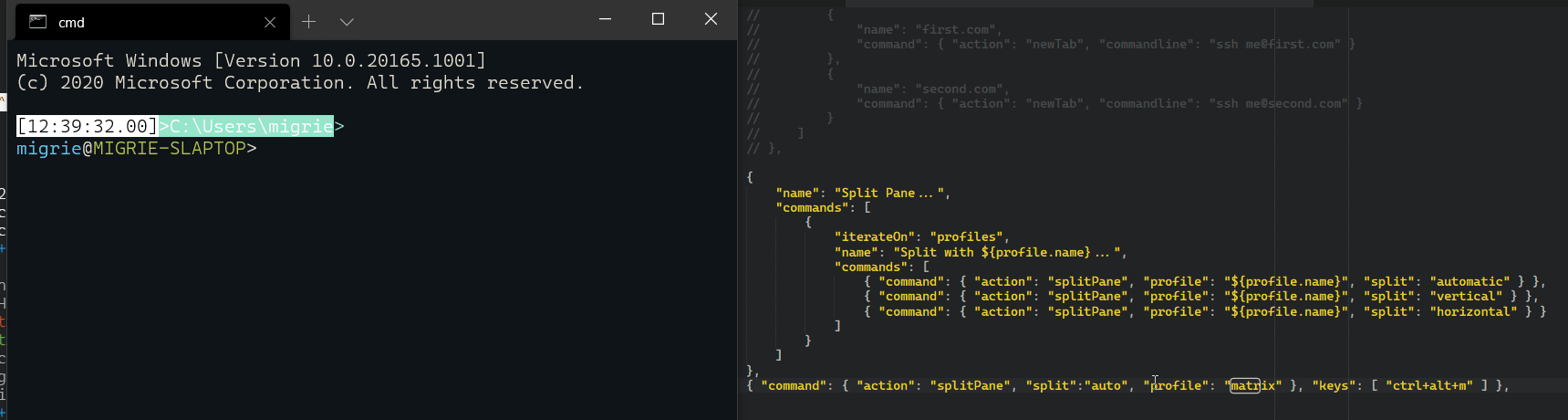
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
Command::Command()
|
|
|
|
|
{
|
|
|
|
|
_setAction(nullptr);
|
|
|
|
|
}
|
|
|
|
|
|
2020-10-17 00:14:11 +02:00
|
|
|
|
com_ptr<Command> Command::Copy() const
|
|
|
|
|
{
|
|
|
|
|
auto command{ winrt::make_self<Command>() };
|
|
|
|
|
command->_Name = _Name;
|
|
|
|
|
command->_Action = _Action;
|
|
|
|
|
command->_KeyChordText = _KeyChordText;
|
2021-02-24 00:37:23 +01:00
|
|
|
|
command->_IconPath = _IconPath;
|
2020-10-17 00:14:11 +02:00
|
|
|
|
command->_IterateOn = _IterateOn;
|
|
|
|
|
|
|
|
|
|
command->_originalJson = _originalJson;
|
|
|
|
|
if (HasNestedCommands())
|
|
|
|
|
{
|
|
|
|
|
command->_subcommands = winrt::single_threaded_map<winrt::hstring, Model::Command>();
|
|
|
|
|
for (auto kv : NestedCommands())
|
|
|
|
|
{
|
|
|
|
|
const auto subCmd{ winrt::get_self<Command>(kv.Value()) };
|
|
|
|
|
command->_subcommands.Insert(kv.Key(), *subCmd->Copy());
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
return command;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
IMapView<winrt::hstring, Model::Command> Command::NestedCommands() const
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
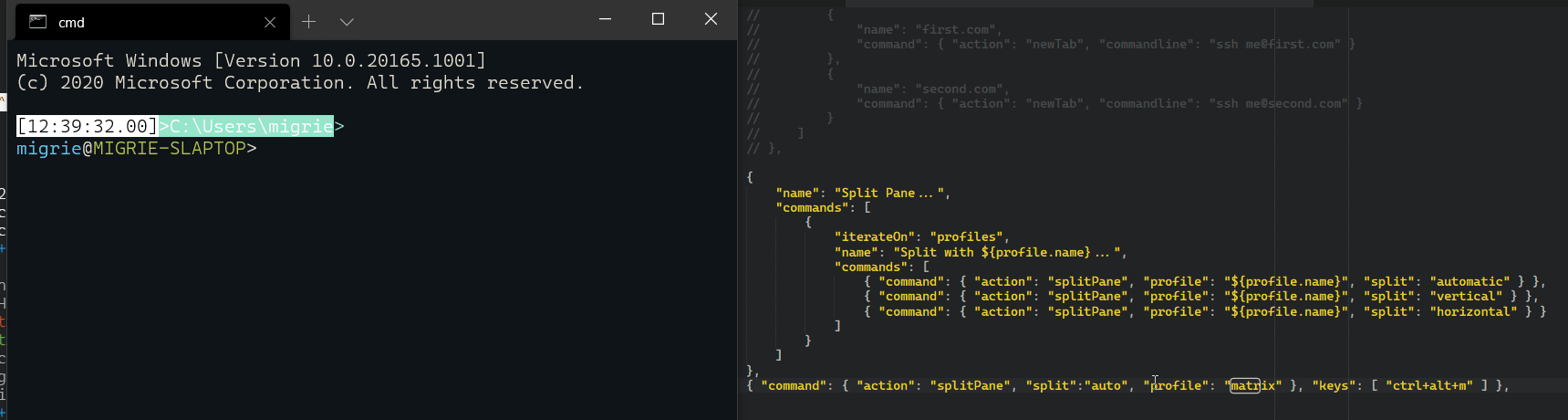
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
{
|
|
|
|
|
return _subcommands ? _subcommands.GetView() : nullptr;
|
|
|
|
|
}
|
|
|
|
|
|
2020-10-17 00:14:11 +02:00
|
|
|
|
bool Command::HasNestedCommands() const
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
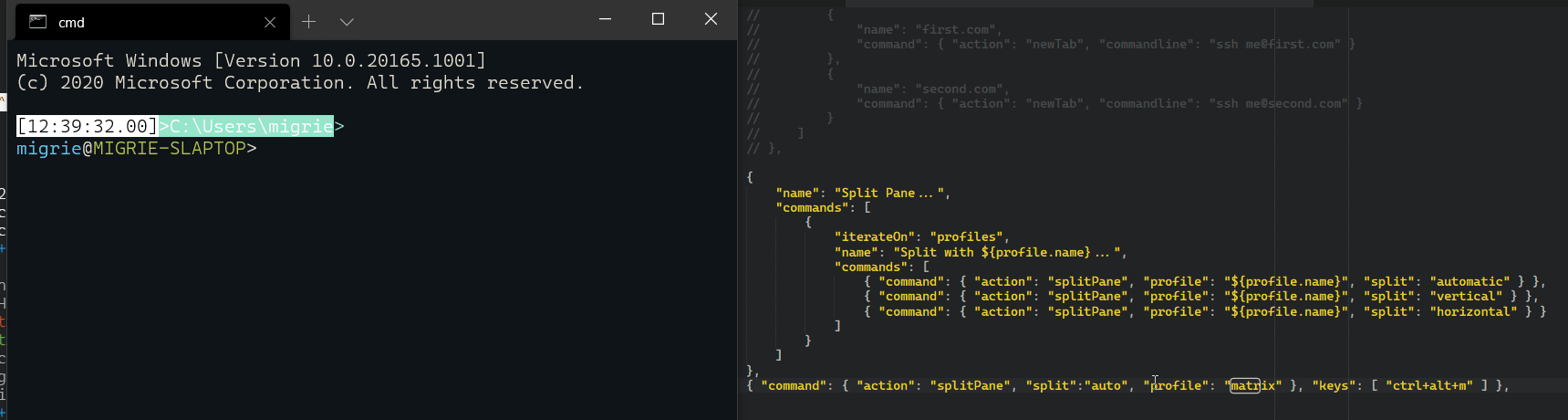
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
{
|
|
|
|
|
return _subcommands ? _subcommands.Size() > 0 : false;
|
|
|
|
|
}
|
2020-06-26 22:38:02 +02:00
|
|
|
|
// Function Description:
|
|
|
|
|
// - attempt to get the name of this command from the provided json object.
|
|
|
|
|
// * If the "name" property is a string, return that value.
|
|
|
|
|
// * If the "name" property is an object, attempt to lookup the string
|
|
|
|
|
// resource specified by the "key" property, to support localizable
|
|
|
|
|
// command names.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - json: The Json::Value representing the command object we should get the name for.
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - the empty string if we couldn't find a name, otherwise the command's name.
|
|
|
|
|
static winrt::hstring _nameFromJson(const Json::Value& json)
|
|
|
|
|
{
|
|
|
|
|
if (const auto name{ json[JsonKey(NameKey)] })
|
|
|
|
|
{
|
|
|
|
|
if (name.isObject())
|
|
|
|
|
{
|
2020-07-17 03:31:09 +02:00
|
|
|
|
if (const auto resourceKey{ JsonUtils::GetValueForKey<std::optional<std::wstring>>(name, "key") })
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
2020-07-17 03:31:09 +02:00
|
|
|
|
if (HasLibraryResourceWithName(*resourceKey))
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
2020-07-17 03:31:09 +02:00
|
|
|
|
return GetLibraryResourceString(*resourceKey);
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
else if (name.isString())
|
|
|
|
|
{
|
2020-07-17 03:31:09 +02:00
|
|
|
|
return JsonUtils::GetValue<winrt::hstring>(name);
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
return L"";
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
|
// - Get the name for the command specified in `json`. If there is no "name"
|
|
|
|
|
// property in the provided json object, then instead generate a name for
|
|
|
|
|
// the provided ActionAndArgs.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - json: json for the command to generate a name for.
|
|
|
|
|
// - actionAndArgs: An ActionAndArgs object to use to generate a name for,
|
|
|
|
|
// if the json object doesn't contain a "name".
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - The "name" from the json, or the generated name from ActionAndArgs::GenerateName
|
|
|
|
|
static winrt::hstring _nameFromJsonOrAction(const Json::Value& json,
|
|
|
|
|
winrt::com_ptr<ActionAndArgs> actionAndArgs)
|
|
|
|
|
{
|
|
|
|
|
auto manualName = _nameFromJson(json);
|
|
|
|
|
if (!manualName.empty())
|
|
|
|
|
{
|
|
|
|
|
return manualName;
|
|
|
|
|
}
|
|
|
|
|
if (!actionAndArgs)
|
|
|
|
|
{
|
|
|
|
|
return L"";
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
return actionAndArgs->GenerateName();
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
|
// - Deserialize a Command from the `json` object. The json object should
|
|
|
|
|
// contain a "name" and "action", and optionally an "icon".
|
|
|
|
|
// * "name": string|object - the name of the command to display in the
|
|
|
|
|
// command palette. If this is an object, look for the "key" property,
|
|
|
|
|
// and try to load the string from our resources instead.
|
|
|
|
|
// * "action": string|object - A ShortcutAction, either as a name or as an
|
|
|
|
|
// ActionAndArgs serialization. See ActionAndArgs::FromJson for details.
|
|
|
|
|
// If this is null, we'll remove this command from the list of commands.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - json: the Json::Value to deserialize into a Command
|
|
|
|
|
// - warnings: If there were any warnings during parsing, they'll be
|
|
|
|
|
// appended to this vector.
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - the newly constructed Command object.
|
|
|
|
|
winrt::com_ptr<Command> Command::FromJson(const Json::Value& json,
|
2020-09-11 02:57:02 +02:00
|
|
|
|
std::vector<SettingsLoadWarnings>& warnings)
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
|
|
|
|
auto result = winrt::make_self<Command>();
|
|
|
|
|
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
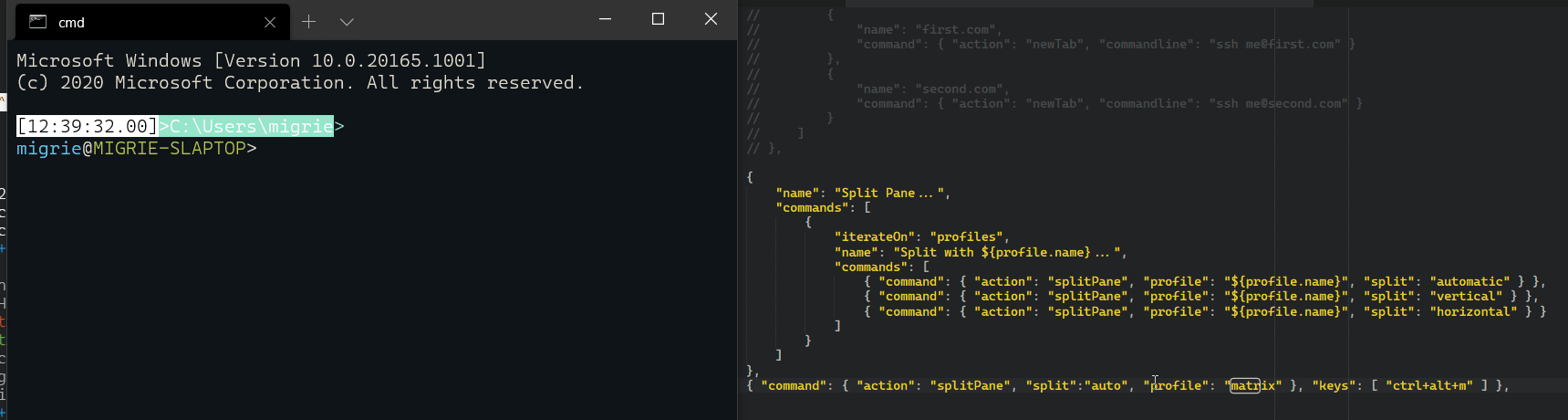
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
bool nested = false;
|
2020-08-19 19:33:19 +02:00
|
|
|
|
JsonUtils::GetValueForKey(json, IterateOnKey, result->_IterateOn);
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
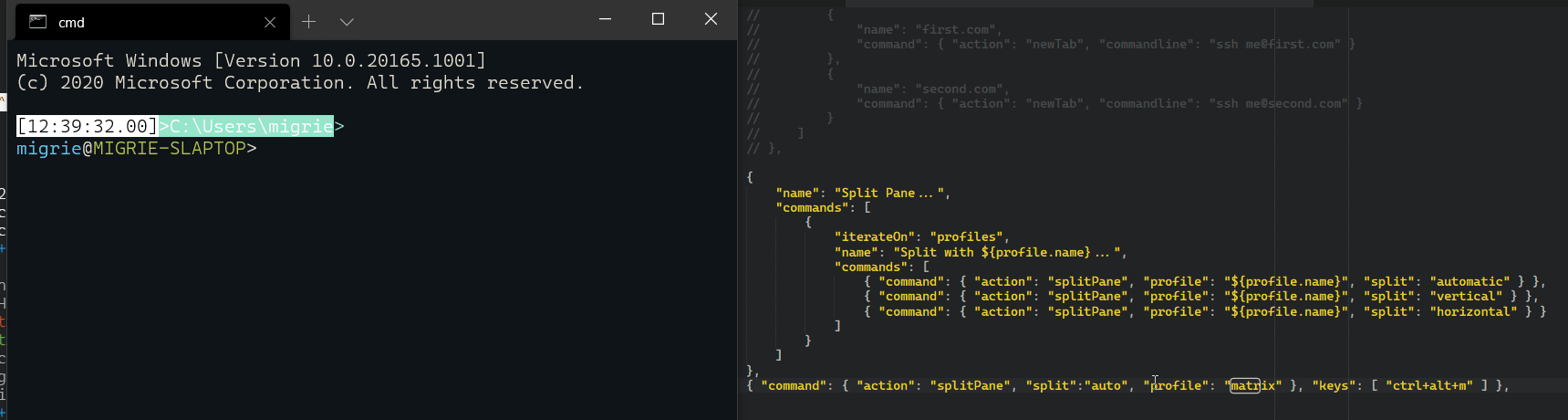
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
|
|
|
|
|
// For iterable commands, we'll make another pass at parsing them once
|
|
|
|
|
// the json is patched. So ignore parsing sub-commands for now. Commands
|
|
|
|
|
// will only be marked iterable on the first pass.
|
|
|
|
|
if (const auto nestedCommandsJson{ json[JsonKey(CommandsKey)] })
|
|
|
|
|
{
|
|
|
|
|
// Initialize our list of subcommands.
|
2020-10-06 18:56:59 +02:00
|
|
|
|
result->_subcommands = winrt::single_threaded_map<winrt::hstring, Model::Command>();
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
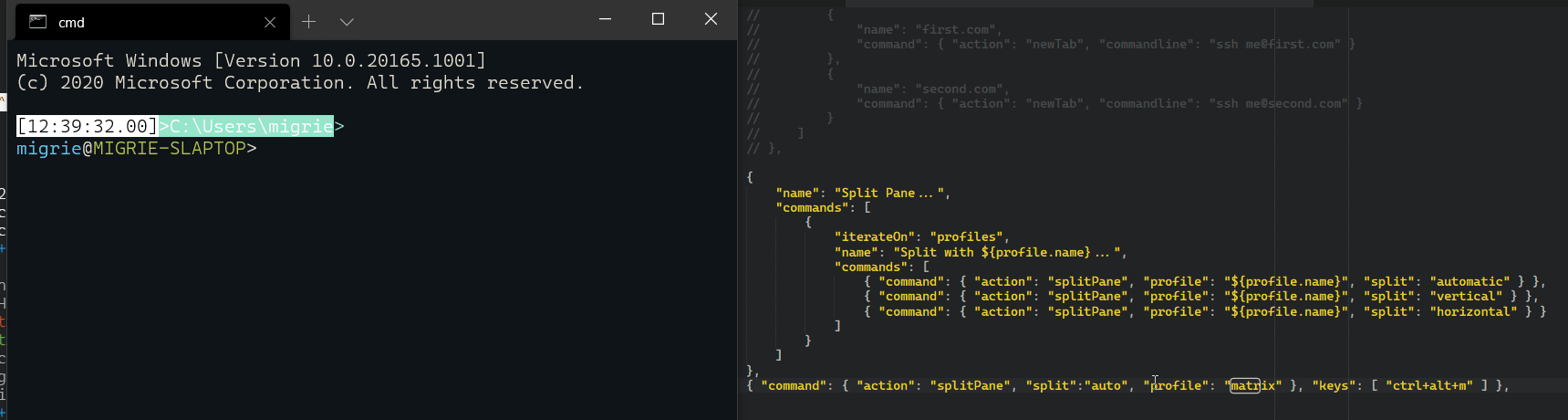
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
auto nestedWarnings = Command::LayerJson(result->_subcommands, nestedCommandsJson);
|
|
|
|
|
// It's possible that the nested commands have some warnings
|
|
|
|
|
warnings.insert(warnings.end(), nestedWarnings.begin(), nestedWarnings.end());
|
|
|
|
|
|
|
|
|
|
nested = true;
|
|
|
|
|
}
|
|
|
|
|
else if (json.isMember(JsonKey(CommandsKey)))
|
|
|
|
|
{
|
|
|
|
|
// { "name": "foo", "commands": null } will land in this case, which
|
|
|
|
|
// should also be used for unbinding.
|
|
|
|
|
return nullptr;
|
|
|
|
|
}
|
|
|
|
|
|
2021-02-24 00:37:23 +01:00
|
|
|
|
JsonUtils::GetValueForKey(json, IconKey, result->_IconPath);
|
2020-06-26 22:38:02 +02:00
|
|
|
|
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
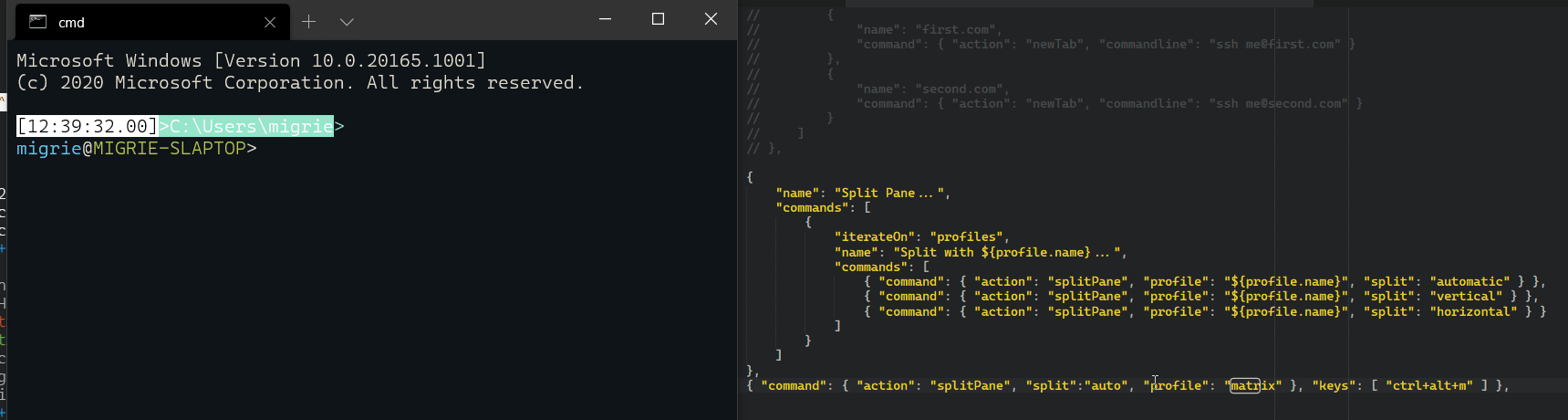
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
// If we're a nested command, we can ignore the current action.
|
|
|
|
|
if (!nested)
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
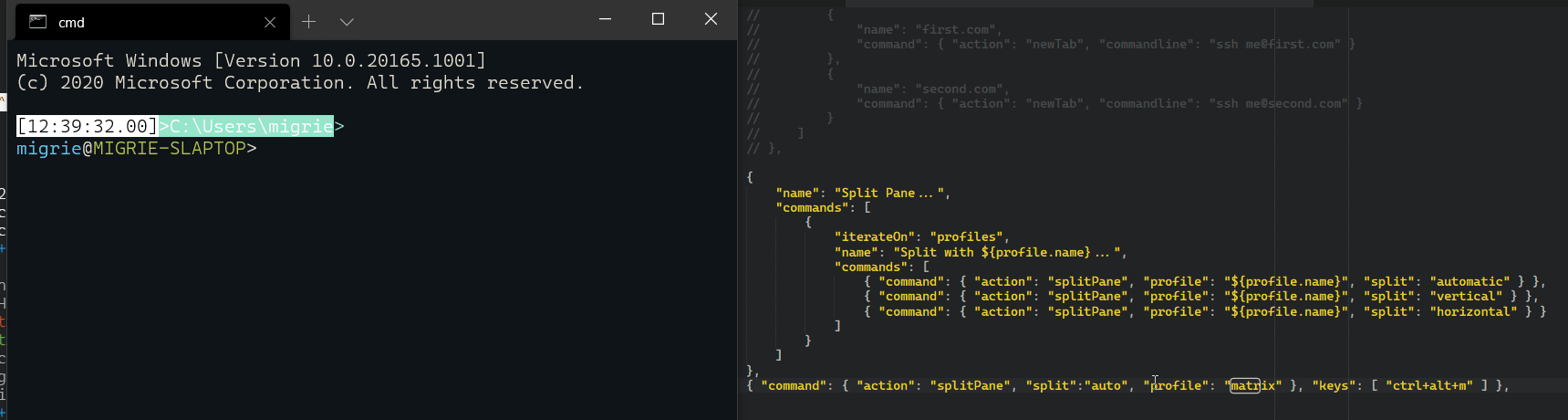
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
if (const auto actionJson{ json[JsonKey(ActionKey)] })
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
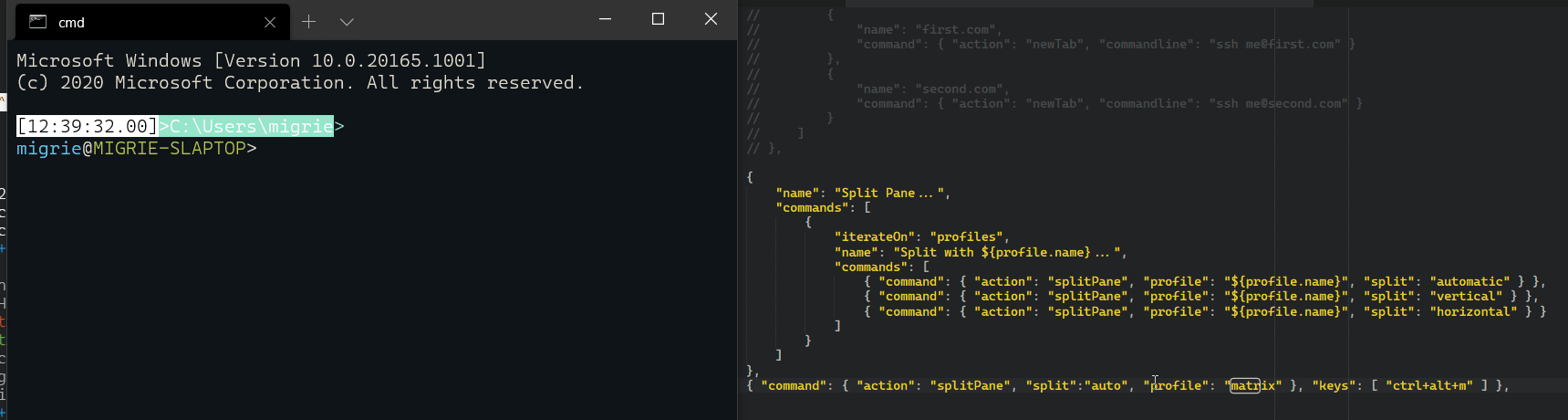
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
auto actionAndArgs = ActionAndArgs::FromJson(actionJson, warnings);
|
|
|
|
|
|
|
|
|
|
if (actionAndArgs)
|
|
|
|
|
{
|
|
|
|
|
result->_setAction(*actionAndArgs);
|
|
|
|
|
}
|
|
|
|
|
else
|
|
|
|
|
{
|
|
|
|
|
// Something like
|
|
|
|
|
// { name: "foo", action: "unbound" }
|
|
|
|
|
// will _remove_ the "foo" command, by returning null here.
|
|
|
|
|
return nullptr;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// If an iterable command doesn't have a name set, we'll still just
|
|
|
|
|
// try and generate a fake name for the command give the string we
|
|
|
|
|
// currently have. It'll probably generate something like "New tab,
|
|
|
|
|
// profile: ${profile.name}". This string will only be temporarily
|
|
|
|
|
// used internally, so there's no problem.
|
|
|
|
|
result->_setName(_nameFromJsonOrAction(json, actionAndArgs));
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|
|
|
|
|
else
|
|
|
|
|
{
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
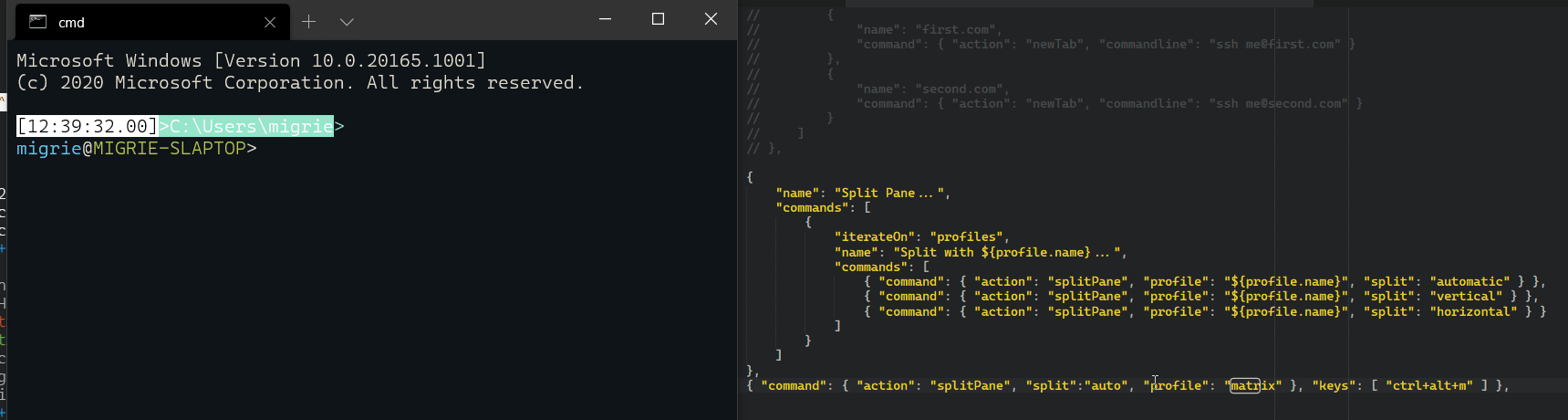
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
// { name: "foo", action: null } will land in this case, which
|
|
|
|
|
// should also be used for unbinding.
|
2020-06-26 22:38:02 +02:00
|
|
|
|
return nullptr;
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
else
|
|
|
|
|
{
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
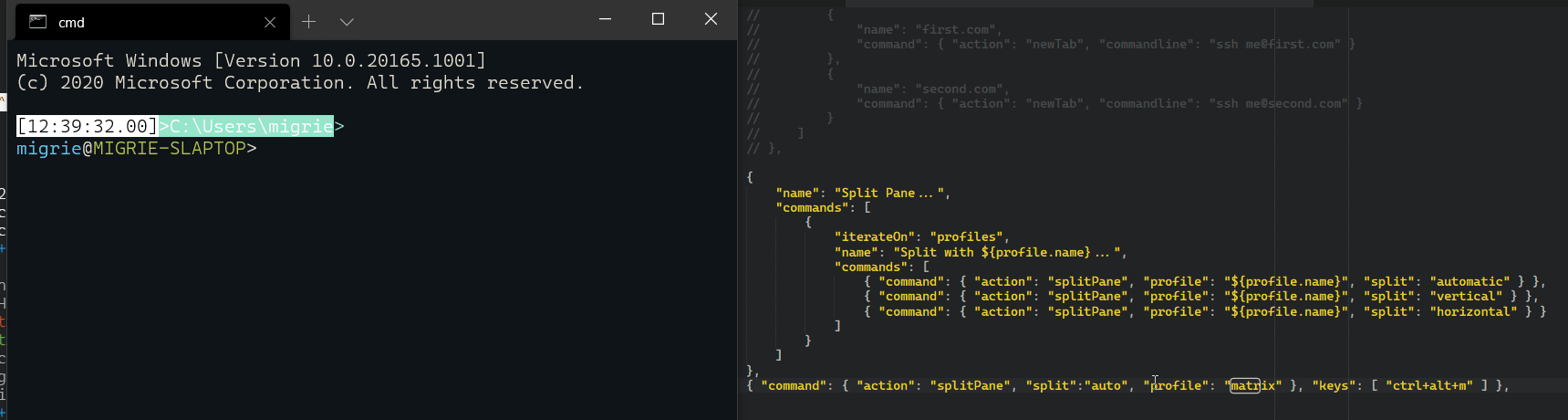
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
result->_setName(_nameFromJson(json));
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|
|
|
|
|
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
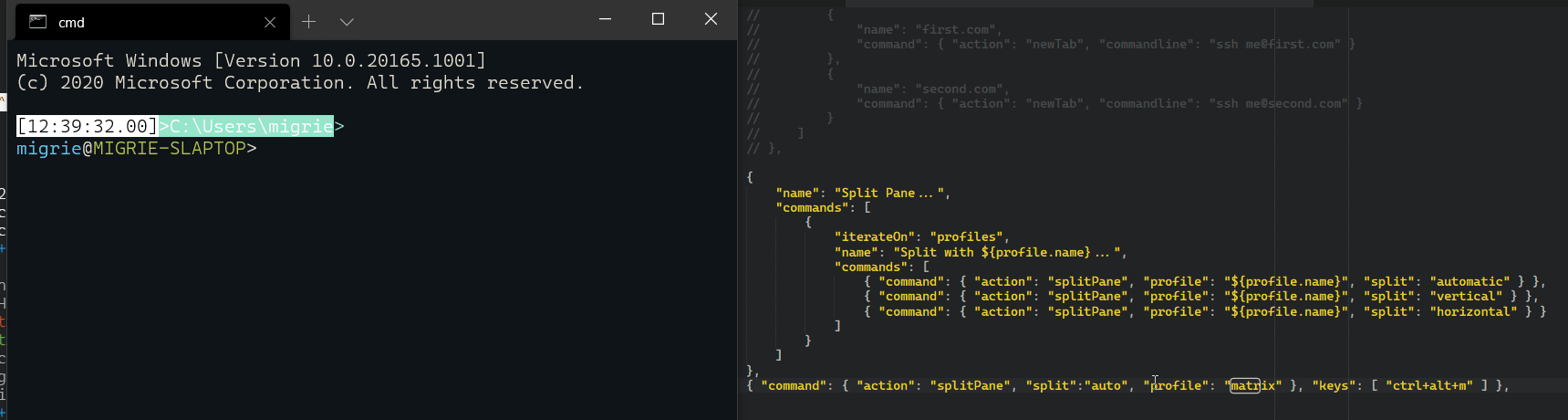
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
// Stash the original json value in this object. If the command is
|
|
|
|
|
// iterable, we'll need to re-parse it later, once we know what all the
|
|
|
|
|
// values we can iterate on are.
|
|
|
|
|
result->_originalJson = json;
|
|
|
|
|
|
2020-06-26 22:38:02 +02:00
|
|
|
|
if (result->_Name.empty())
|
|
|
|
|
{
|
|
|
|
|
return nullptr;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
return result;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Function Description:
|
|
|
|
|
// - Attempt to parse all the json objects in `json` into new Command
|
|
|
|
|
// objects, and add them to the map of commands.
|
|
|
|
|
// - If any parsed command has
|
|
|
|
|
// the same Name as an existing command in commands, the new one will
|
|
|
|
|
// layer on top of the existing one.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - commands: a map of Name->Command which new commands should be layered upon.
|
|
|
|
|
// - json: A Json::Value containing an array of serialized commands
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - A vector containing any warnings detected while parsing
|
2020-10-06 18:56:59 +02:00
|
|
|
|
std::vector<SettingsLoadWarnings> Command::LayerJson(IMap<winrt::hstring, Model::Command>& commands,
|
2020-09-11 02:57:02 +02:00
|
|
|
|
const Json::Value& json)
|
2020-06-26 22:38:02 +02:00
|
|
|
|
{
|
2020-09-11 02:57:02 +02:00
|
|
|
|
std::vector<SettingsLoadWarnings> warnings;
|
2020-06-26 22:38:02 +02:00
|
|
|
|
|
|
|
|
|
for (const auto& value : json)
|
|
|
|
|
{
|
|
|
|
|
if (value.isObject())
|
|
|
|
|
{
|
|
|
|
|
try
|
|
|
|
|
{
|
|
|
|
|
auto result = Command::FromJson(value, warnings);
|
|
|
|
|
if (result)
|
|
|
|
|
{
|
|
|
|
|
// Override commands with the same name
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
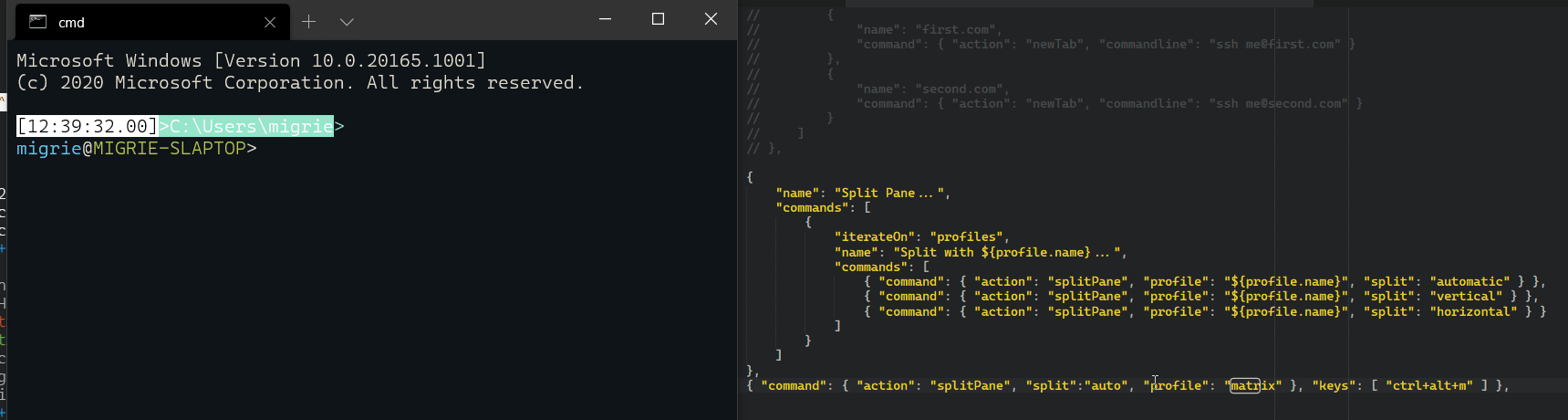
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
commands.Insert(result->Name(), *result);
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|
|
|
|
|
else
|
|
|
|
|
{
|
|
|
|
|
// If there wasn't a parsed command, then try to get the
|
|
|
|
|
// name from the json blob. If that name currently
|
|
|
|
|
// exists in our list of commands, we should remove it.
|
|
|
|
|
const auto name = _nameFromJson(value);
|
|
|
|
|
if (!name.empty())
|
|
|
|
|
{
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
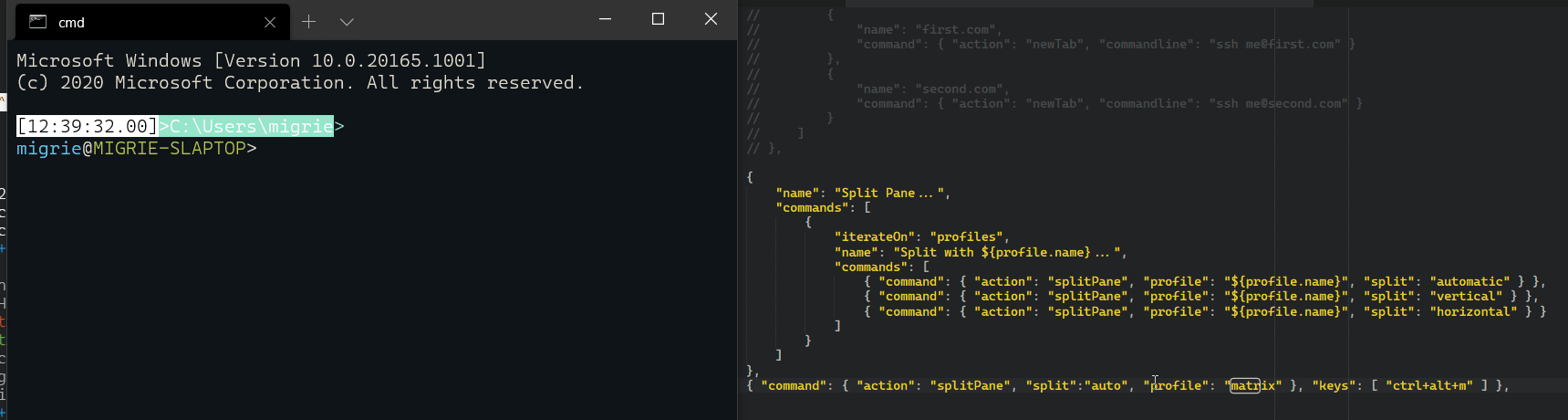
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
commands.Remove(name);
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
CATCH_LOG();
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
return warnings;
|
|
|
|
|
}
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
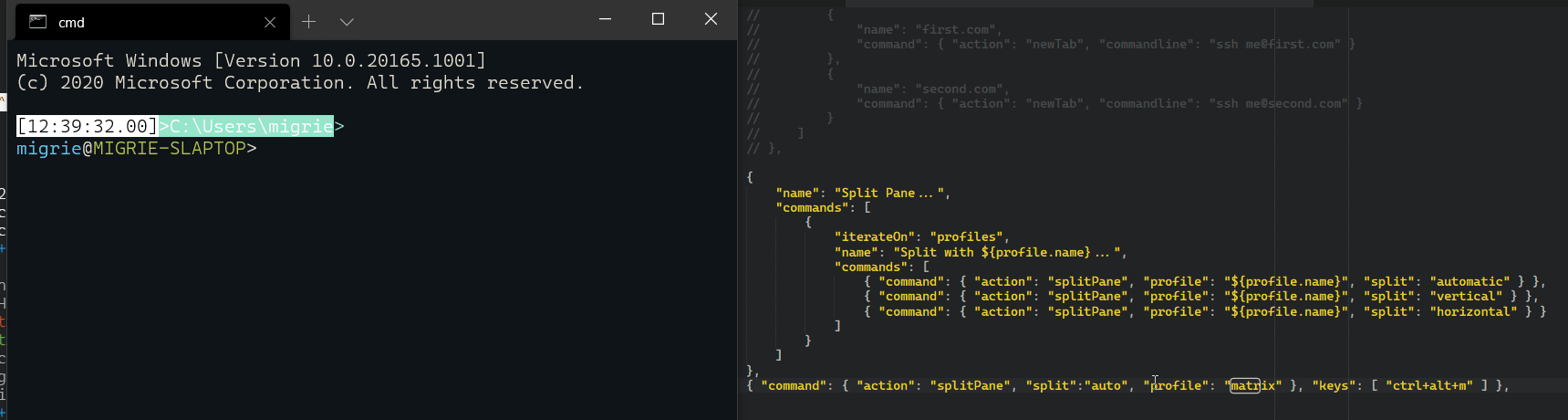
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
|
|
|
|
|
// Function Description:
|
|
|
|
|
// - Helper to escape a string as a json string. This function will also
|
|
|
|
|
// trim off the leading and trailing double-quotes, so the output string
|
|
|
|
|
// can be inserted directly into another json blob.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - input: the string to JSON escape.
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - the input string escaped properly to be inserted into another json blob.
|
|
|
|
|
std::string _escapeForJson(const std::string& input)
|
|
|
|
|
{
|
|
|
|
|
Json::Value inJson{ input };
|
|
|
|
|
Json::StreamWriterBuilder builder;
|
|
|
|
|
builder.settings_["indentation"] = "";
|
|
|
|
|
std::string out{ Json::writeString(builder, inJson) };
|
|
|
|
|
if (out.size() >= 2)
|
|
|
|
|
{
|
|
|
|
|
// trim off the leading/trailing '"'s
|
|
|
|
|
auto ss{ out.substr(1, out.size() - 2) };
|
|
|
|
|
return ss;
|
|
|
|
|
}
|
|
|
|
|
return out;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
|
// - Iterate over all the provided commands, and recursively expand any
|
|
|
|
|
// commands with `iterateOn` set. If we successfully generated expanded
|
|
|
|
|
// commands for them, then we'll remove the original command, and add all
|
|
|
|
|
// the newly generated commands.
|
|
|
|
|
// - For more specific implementation details, see _expandCommand.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - commands: a map of commands to expand. Newly created commands will be
|
|
|
|
|
// inserted into the map to replace the expandable commands.
|
|
|
|
|
// - profiles: A list of all the profiles that this command should be expanded on.
|
|
|
|
|
// - warnings: If there were any warnings during parsing, they'll be
|
|
|
|
|
// appended to this vector.
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - <none>
|
2020-10-06 18:56:59 +02:00
|
|
|
|
void Command::ExpandCommands(IMap<winrt::hstring, Model::Command> commands,
|
|
|
|
|
IVectorView<Model::Profile> profiles,
|
|
|
|
|
IVectorView<Model::ColorScheme> schemes,
|
|
|
|
|
IVector<SettingsLoadWarnings> warnings)
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
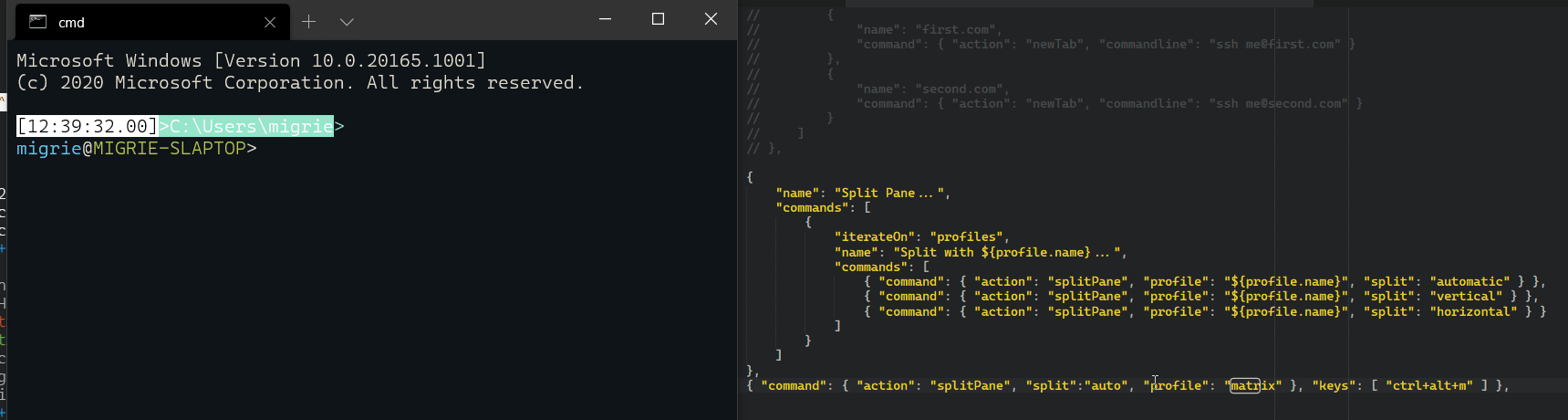
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
{
|
|
|
|
|
std::vector<winrt::hstring> commandsToRemove;
|
2020-10-06 18:56:59 +02:00
|
|
|
|
std::vector<Model::Command> commandsToAdd;
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
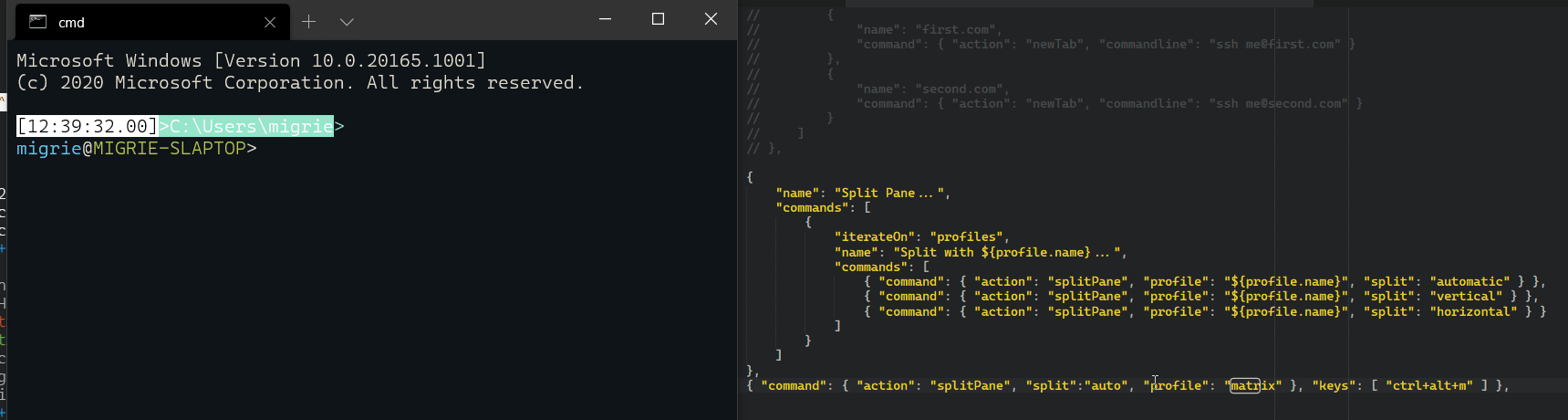
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
|
|
|
|
|
// First, collect up all the commands that need replacing.
|
|
|
|
|
for (const auto& nameAndCmd : commands)
|
|
|
|
|
{
|
|
|
|
|
auto cmd{ get_self<implementation::Command>(nameAndCmd.Value()) };
|
|
|
|
|
|
2020-08-19 19:33:19 +02:00
|
|
|
|
auto newCommands = _expandCommand(cmd, profiles, schemes, warnings);
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
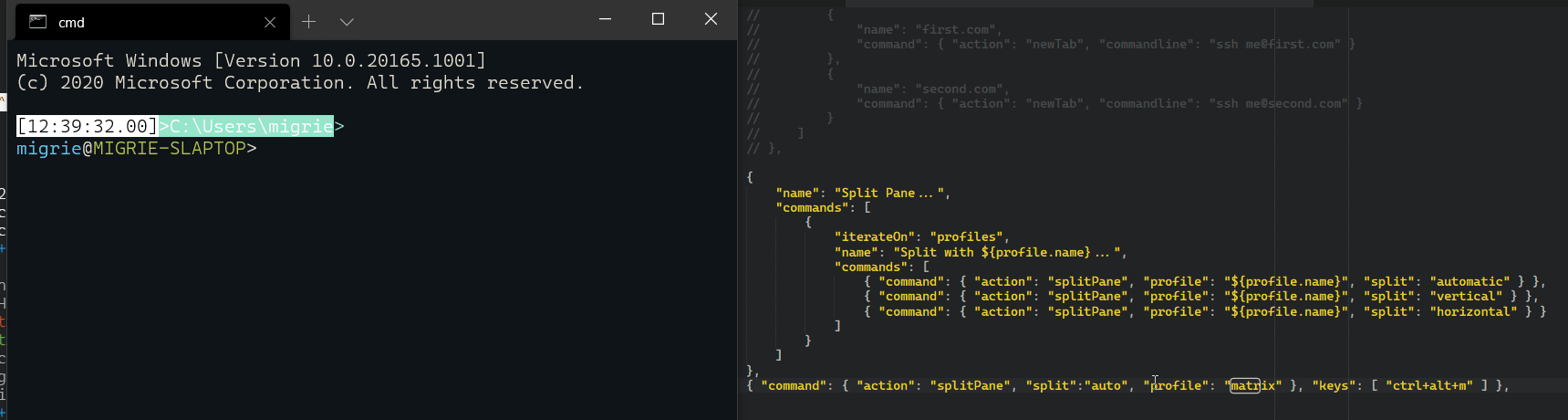
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
if (newCommands.size() > 0)
|
|
|
|
|
{
|
|
|
|
|
commandsToRemove.push_back(nameAndCmd.Key());
|
|
|
|
|
commandsToAdd.insert(commandsToAdd.end(), newCommands.begin(), newCommands.end());
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Second, remove all the commands that need to be removed.
|
|
|
|
|
for (auto& name : commandsToRemove)
|
|
|
|
|
{
|
|
|
|
|
commands.Remove(name);
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Finally, add all the new commands.
|
|
|
|
|
for (auto& cmd : commandsToAdd)
|
|
|
|
|
{
|
|
|
|
|
commands.Insert(cmd.Name(), cmd);
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Function Description:
|
|
|
|
|
// - Attempts to expand the given command into many commands, if the command
|
|
|
|
|
// has `"iterateOn": "profiles"` set.
|
|
|
|
|
// - If it doesn't, this function will do
|
|
|
|
|
// nothing and return an empty vector.
|
|
|
|
|
// - If it does, we're going to attempt to build a new set of commands using
|
|
|
|
|
// the given command as a prototype. We'll attempt to create a new command
|
|
|
|
|
// for each and every profile, to replace the original command.
|
|
|
|
|
// * For the new commands, we'll replace any instance of "${profile.name}"
|
|
|
|
|
// in the original json used to create this action with the name of the
|
|
|
|
|
// given profile.
|
|
|
|
|
// - If we encounter any errors while re-parsing the json with the replaced
|
|
|
|
|
// name, we'll just return immediately.
|
|
|
|
|
// - At the end, we'll return all the new commands we've build for the given command.
|
|
|
|
|
// Arguments:
|
|
|
|
|
// - expandable: the Command to potentially turn into more commands
|
|
|
|
|
// - profiles: A list of all the profiles that this command should be expanded on.
|
|
|
|
|
// - warnings: If there were any warnings during parsing, they'll be
|
|
|
|
|
// appended to this vector.
|
|
|
|
|
// Return Value:
|
|
|
|
|
// - and empty vector if the command wasn't expandable, otherwise a list of
|
|
|
|
|
// the newly-created commands.
|
2020-10-06 18:56:59 +02:00
|
|
|
|
std::vector<Model::Command> Command::_expandCommand(Command* const expandable,
|
|
|
|
|
IVectorView<Model::Profile> profiles,
|
|
|
|
|
IVectorView<Model::ColorScheme> schemes,
|
|
|
|
|
IVector<SettingsLoadWarnings>& warnings)
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
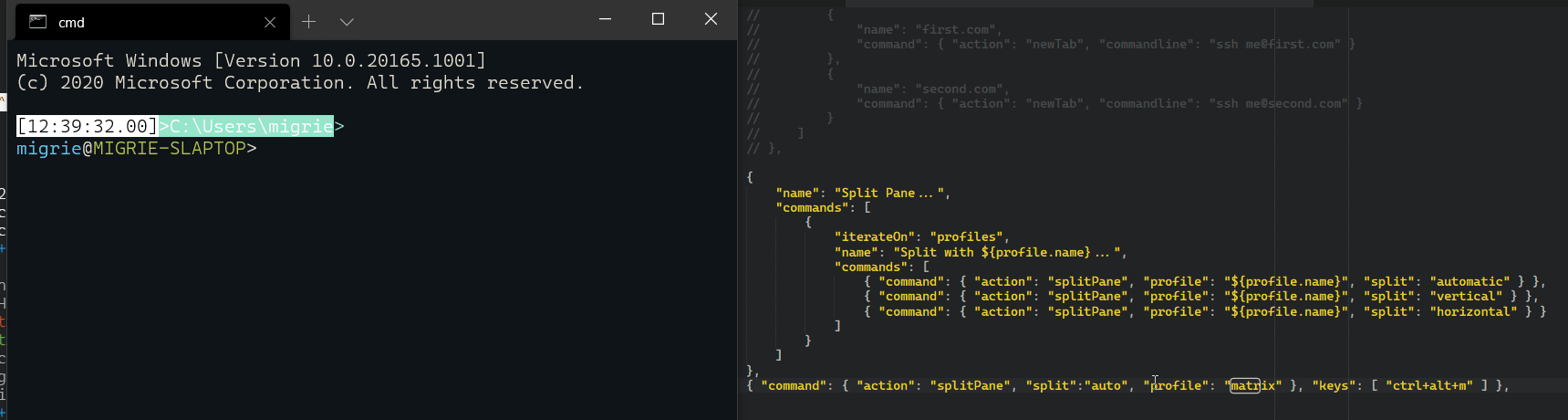
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
{
|
2020-10-06 18:56:59 +02:00
|
|
|
|
std::vector<Model::Command> newCommands;
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
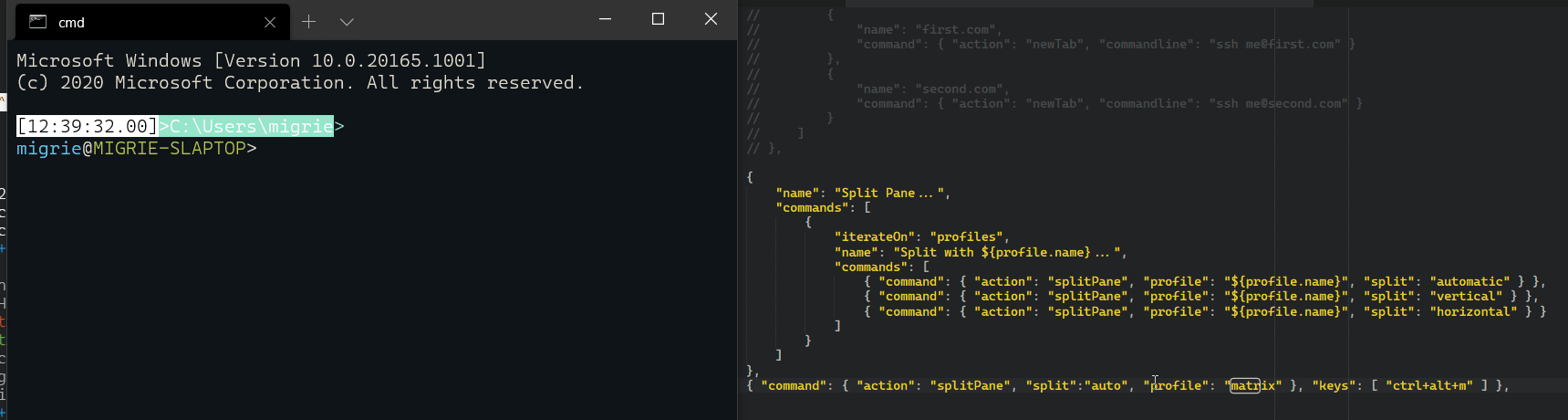
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
|
|
|
|
|
if (expandable->HasNestedCommands())
|
|
|
|
|
{
|
2020-08-19 19:33:19 +02:00
|
|
|
|
ExpandCommands(expandable->_subcommands, profiles, schemes, warnings);
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
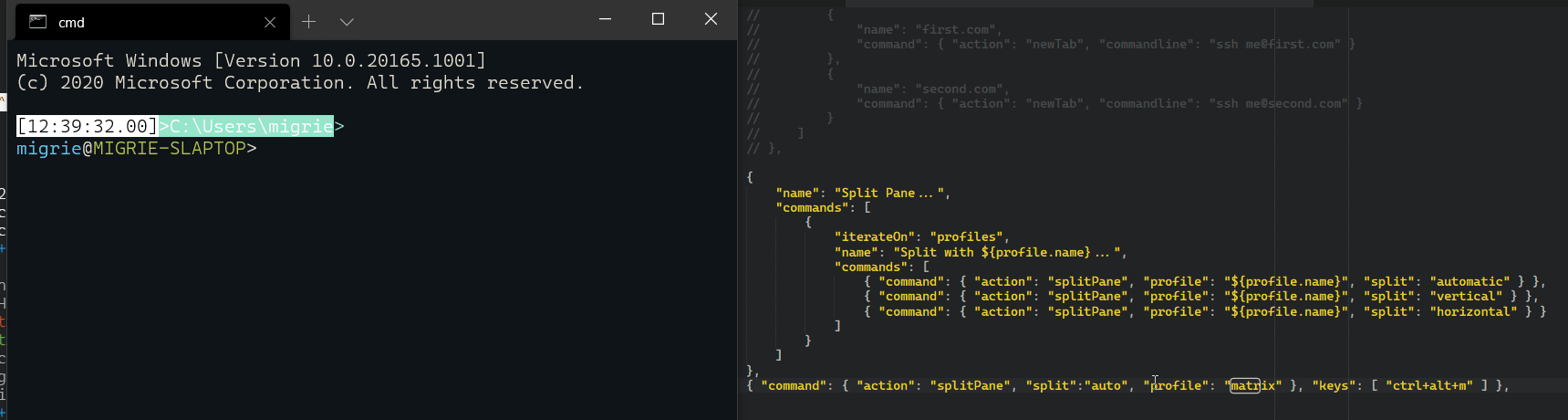
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
if (expandable->_IterateOn == ExpandCommandType::None)
|
|
|
|
|
{
|
|
|
|
|
return newCommands;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
std::string errs; // This string will receive any error text from failing to parse.
|
|
|
|
|
std::unique_ptr<Json::CharReader> reader{ Json::CharReaderBuilder::CharReaderBuilder().newCharReader() };
|
|
|
|
|
|
|
|
|
|
// First, get a string for the original Json::Value
|
|
|
|
|
auto oldJsonString = expandable->_originalJson.toStyledString();
|
|
|
|
|
|
2020-08-19 19:33:19 +02:00
|
|
|
|
auto reParseJson = [&](const auto& newJsonString) -> bool {
|
|
|
|
|
// - Now, re-parse the modified value.
|
|
|
|
|
Json::Value newJsonValue;
|
|
|
|
|
const auto actualDataStart = newJsonString.data();
|
|
|
|
|
const auto actualDataEnd = newJsonString.data() + newJsonString.size();
|
|
|
|
|
if (!reader->parse(actualDataStart, actualDataEnd, &newJsonValue, &errs))
|
|
|
|
|
{
|
2020-10-06 18:56:59 +02:00
|
|
|
|
warnings.Append(SettingsLoadWarnings::FailedToParseCommandJson);
|
2020-08-19 19:33:19 +02:00
|
|
|
|
// If we encounter a re-parsing error, just stop processing the rest of the commands.
|
|
|
|
|
return false;
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
// Pass the new json back though FromJson, to get the new expanded value.
|
2020-10-06 18:56:59 +02:00
|
|
|
|
std::vector<SettingsLoadWarnings> newWarnings;
|
|
|
|
|
if (auto newCmd{ Command::FromJson(newJsonValue, newWarnings) })
|
2020-08-19 19:33:19 +02:00
|
|
|
|
{
|
|
|
|
|
newCommands.push_back(*newCmd);
|
|
|
|
|
}
|
2020-10-06 18:56:59 +02:00
|
|
|
|
std::for_each(newWarnings.begin(), newWarnings.end(), [warnings](auto& warn) { warnings.Append(warn); });
|
2020-08-19 19:33:19 +02:00
|
|
|
|
return true;
|
|
|
|
|
};
|
|
|
|
|
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
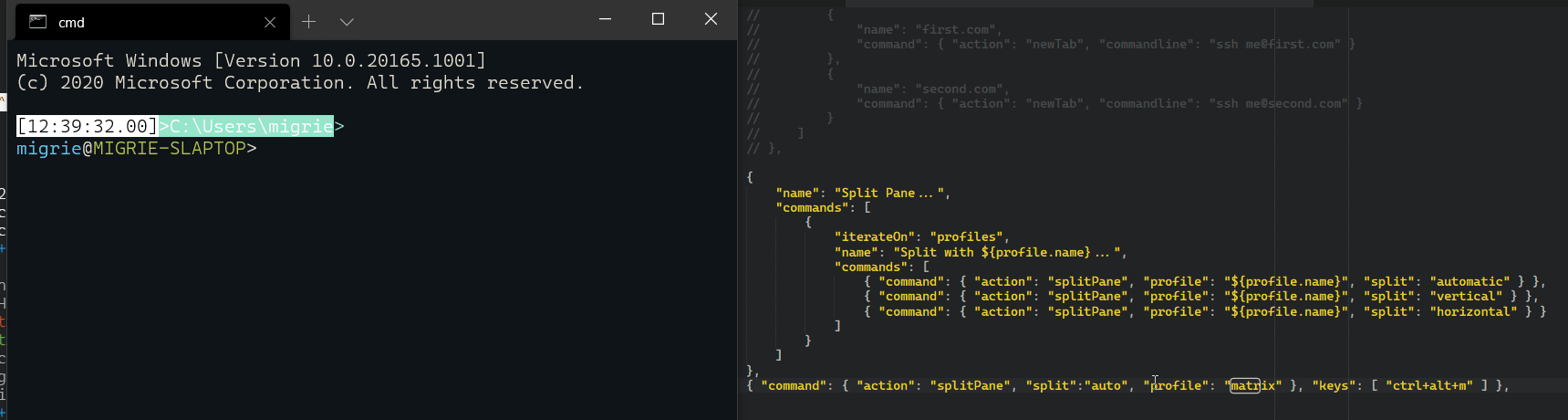
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
if (expandable->_IterateOn == ExpandCommandType::Profiles)
|
|
|
|
|
{
|
|
|
|
|
for (const auto& p : profiles)
|
|
|
|
|
{
|
|
|
|
|
// For each profile, create a new command. This command will have:
|
|
|
|
|
// * the icon path and keychord text of the original command
|
|
|
|
|
// * the Name will have any instances of "${profile.name}"
|
|
|
|
|
// replaced with the profile's name
|
|
|
|
|
// * for the action, we'll take the original json, replace any
|
|
|
|
|
// instances of "${profile.name}" with the profile's name,
|
|
|
|
|
// then re-attempt to parse the action and args.
|
|
|
|
|
|
|
|
|
|
// Replace all the keywords in the original json, and try and parse that
|
|
|
|
|
|
|
|
|
|
// - Escape the profile name for JSON appropriately
|
2020-08-28 03:09:22 +02:00
|
|
|
|
auto escapedProfileName = _escapeForJson(til::u16u8(p.Name()));
|
2020-10-08 20:29:04 +02:00
|
|
|
|
auto escapedProfileIcon = _escapeForJson(til::u16u8(p.Icon()));
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
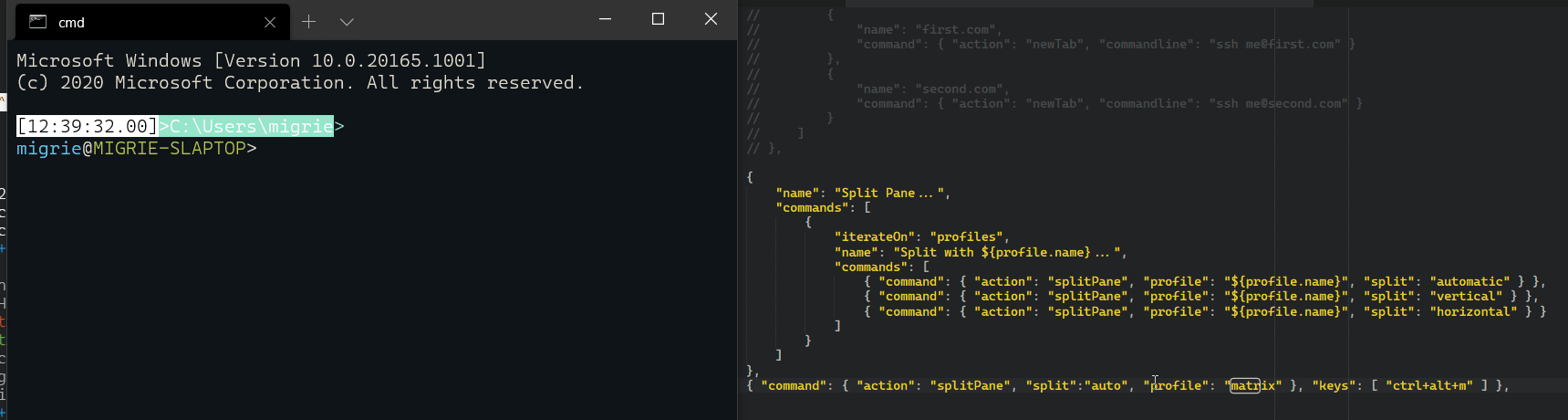
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
auto newJsonString = til::replace_needle_in_haystack(oldJsonString,
|
2020-08-19 19:33:19 +02:00
|
|
|
|
ProfileNameToken,
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
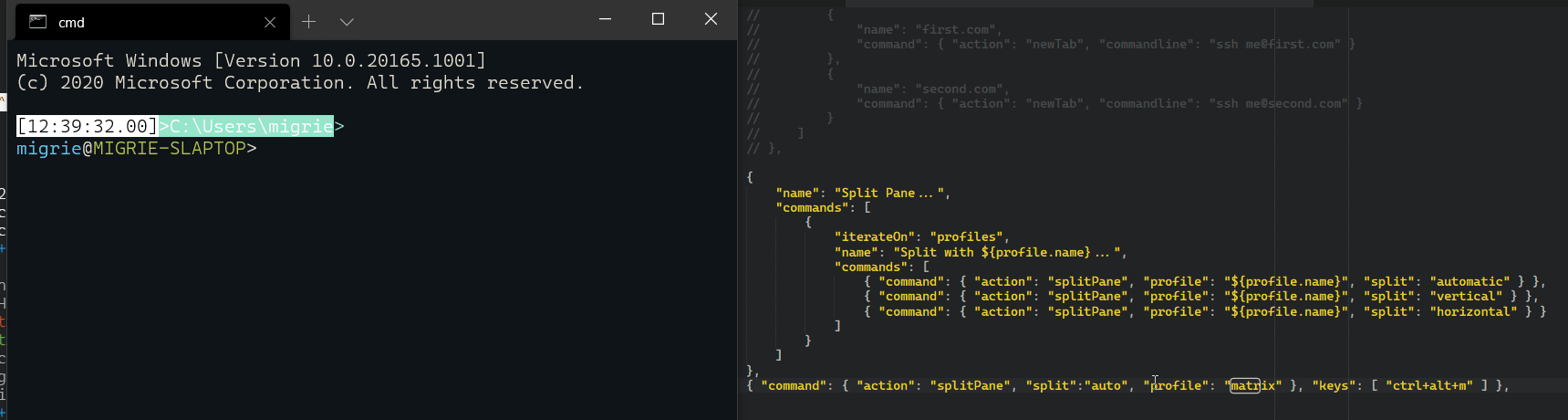
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
escapedProfileName);
|
2020-08-21 20:08:02 +02:00
|
|
|
|
til::replace_needle_in_haystack_inplace(newJsonString,
|
|
|
|
|
ProfileIconToken,
|
|
|
|
|
escapedProfileIcon);
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
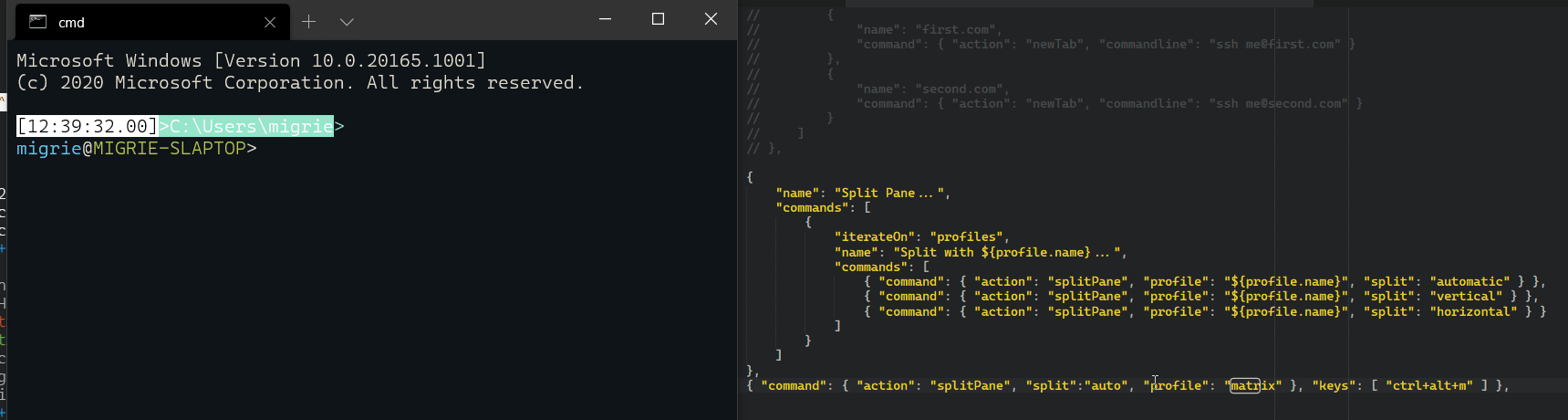
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
|
2020-08-19 19:33:19 +02:00
|
|
|
|
// If we encounter a re-parsing error, just stop processing the rest of the commands.
|
|
|
|
|
if (!reParseJson(newJsonString))
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
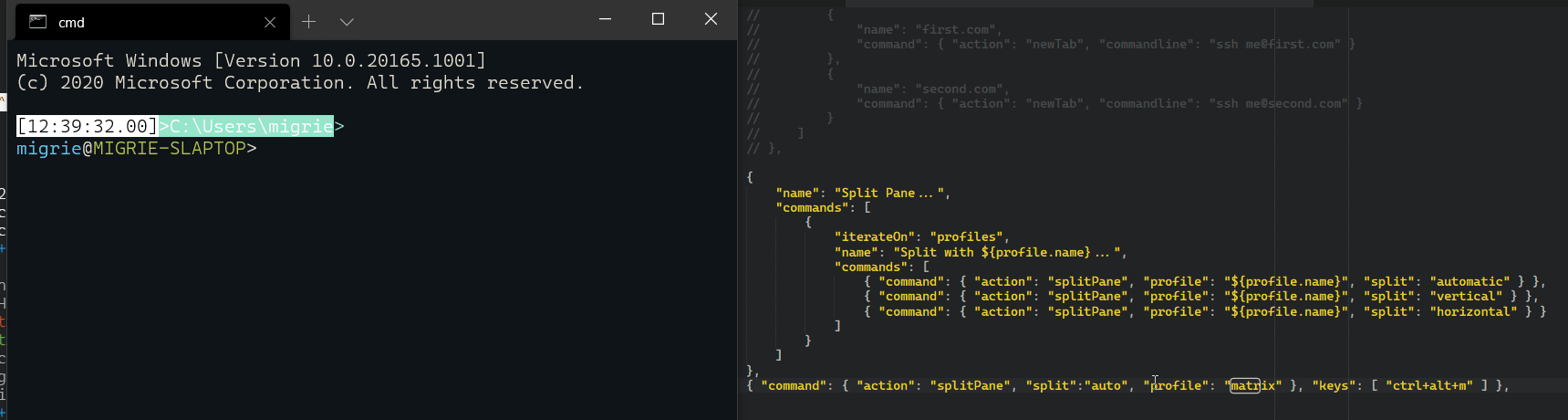
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
{
|
|
|
|
|
break;
|
|
|
|
|
}
|
2020-08-19 19:33:19 +02:00
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
else if (expandable->_IterateOn == ExpandCommandType::ColorSchemes)
|
|
|
|
|
{
|
|
|
|
|
for (const auto& s : schemes)
|
|
|
|
|
{
|
|
|
|
|
// For each scheme, create a new command. We'll take the
|
|
|
|
|
// original json, replace any instances of "${scheme.name}" with
|
|
|
|
|
// the scheme's name, then re-attempt to parse the action and
|
|
|
|
|
// args.
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
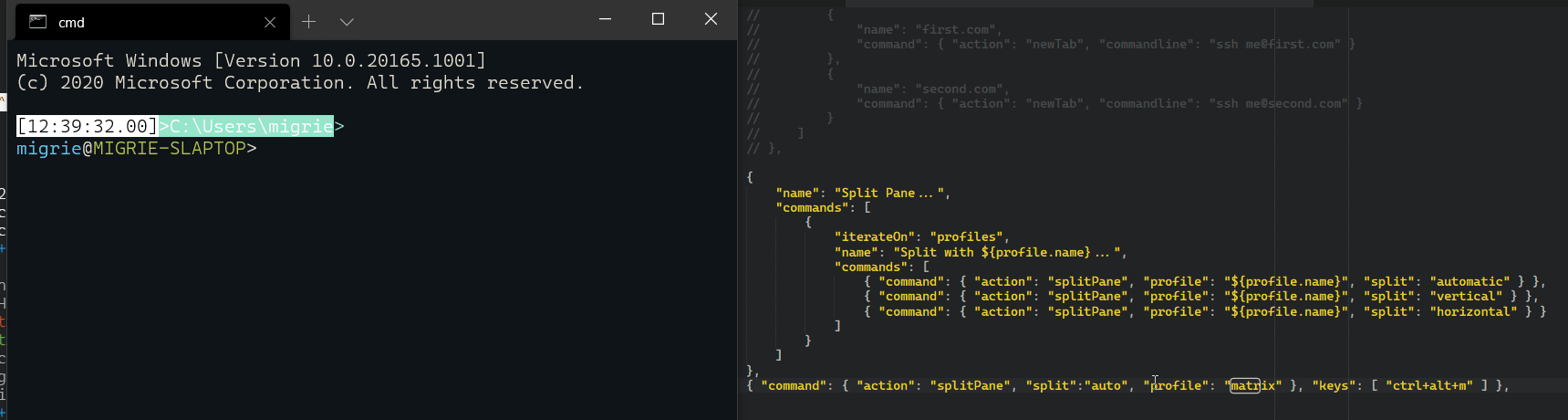
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
|
2020-08-19 19:33:19 +02:00
|
|
|
|
// - Escape the profile name for JSON appropriately
|
|
|
|
|
auto escapedSchemeName = _escapeForJson(til::u16u8(s.Name()));
|
|
|
|
|
auto newJsonString = til::replace_needle_in_haystack(oldJsonString,
|
|
|
|
|
SchemeNameToken,
|
|
|
|
|
escapedSchemeName);
|
|
|
|
|
|
|
|
|
|
// If we encounter a re-parsing error, just stop processing the rest of the commands.
|
|
|
|
|
if (!reParseJson(newJsonString))
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
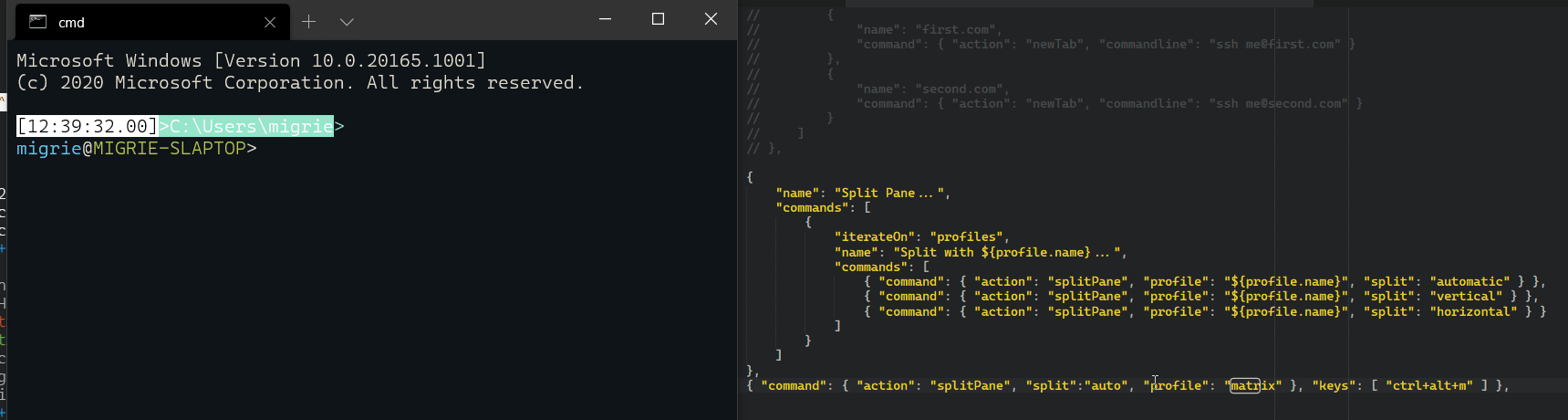
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
{
|
2020-08-19 19:33:19 +02:00
|
|
|
|
break;
|
Add support for iterable, nested commands (#6856)
## Summary of the Pull Request
This PR adds support for both _nested_ and _iterable_ commands in the Command palette.
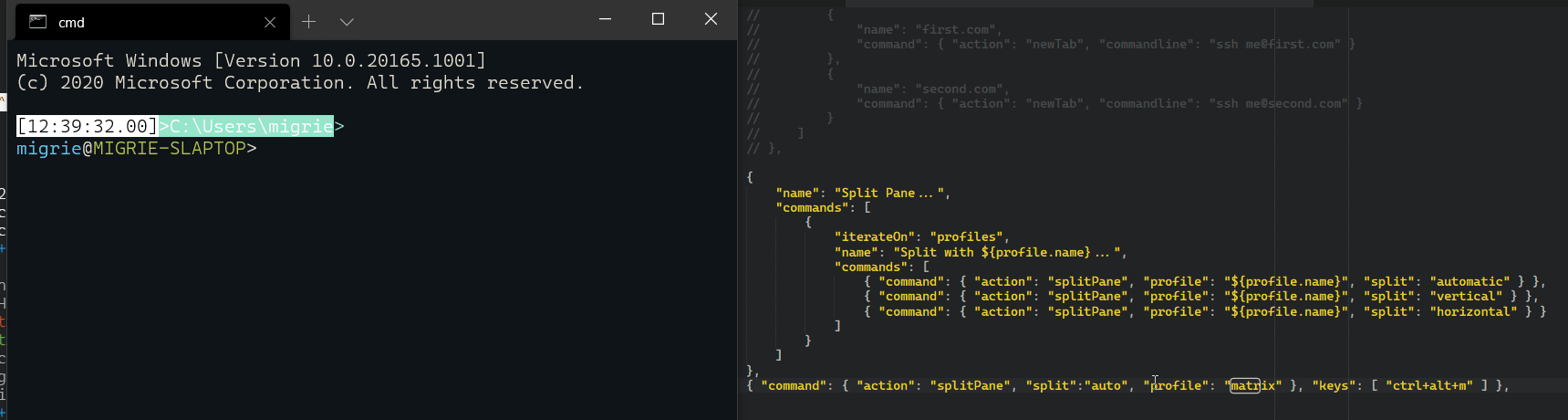
* **Nested commands**: These are commands that include additional sub-commands. When the user selects on of these, the palette will update to only show the nested commands.
* **Iterable commands**: These are commands what allow the user to define only a single command, which is repeated once for every profile. (in the future, also repeated for color schemes, themes, etc.)
The above gif uses the following json:
```json
{
"name": "Split Pane...",
"commands": [
{
"iterateOn": "profiles",
"name": "Split with ${profile.name}...",
"commands": [
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "automatic" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "vertical" } },
{ "command": { "action": "splitPane", "profile": "${profile.name}", "split": "horizontal" } }
]
}
]
},
```
## References
## PR Checklist
* [x] Closes #3994
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - Sure does, but we'll finish polishing this first.
## Detailed Description of the Pull Request / Additional comments
We've now gotta keep the original json for a command around, so that once we know what all the profiles will be, we can expand the commands that need it.
We've also got to parse commands recursively, because they might have any number of child commands.
These together made the command parsing a _lot_ more complicated, but it feels good so far.
## Validation Steps Performed
* wrote a bunch of tests
* Played with it a bunch
2020-08-13 23:22:46 +02:00
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
}
|
|
|
|
|
|
|
|
|
|
return newCommands;
|
|
|
|
|
}
|
2020-06-26 22:38:02 +02:00
|
|
|
|
}
|