Add support for displaying the version with wt --version
(#5501)
## Summary of the Pull Request Here's 3000 words: 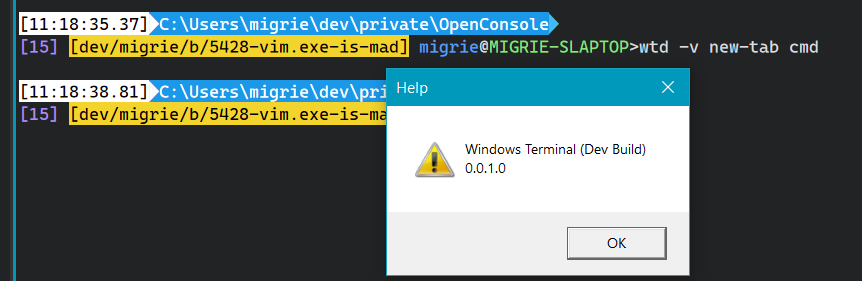 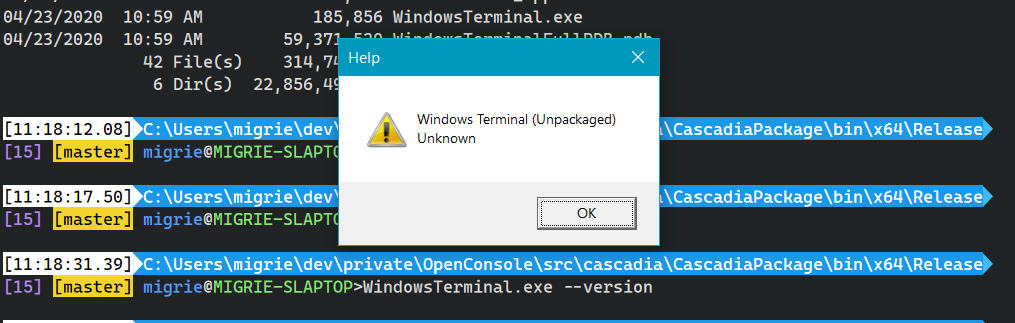 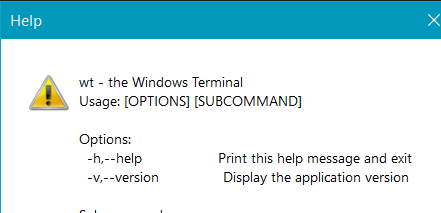 ## References * #4632 ## PR Checklist * [x] Closes #5494 * [x] I work here * [ ] Tests added/passed * [n/a] Requires documentation to be updated
This commit is contained in:
parent
48617b7e06
commit
6ce3357bab
|
@ -2,6 +2,7 @@
|
|||
// Licensed under the MIT license.
|
||||
|
||||
#include "pch.h"
|
||||
#include "AppLogic.h"
|
||||
#include "AppCommandlineArgs.h"
|
||||
#include "ActionArgs.h"
|
||||
#include <LibraryResources.h>
|
||||
|
@ -140,6 +141,11 @@ int AppCommandlineArgs::_handleExit(const CLI::App& command, const CLI::Error& e
|
|||
{
|
||||
_exitMessage = err.str();
|
||||
}
|
||||
|
||||
// We're displaying an error message - we should always exit instead of
|
||||
// actually starting the Terminal.
|
||||
_shouldExitEarly = true;
|
||||
|
||||
return result;
|
||||
}
|
||||
|
||||
|
@ -151,6 +157,22 @@ int AppCommandlineArgs::_handleExit(const CLI::App& command, const CLI::Error& e
|
|||
// - <none>
|
||||
void AppCommandlineArgs::_buildParser()
|
||||
{
|
||||
auto versionCallback = [this](int64_t /*count*/) {
|
||||
if (const auto appLogic{ winrt::TerminalApp::implementation::AppLogic::Current() })
|
||||
{
|
||||
// Set our message to display the application name and the current version.
|
||||
_exitMessage = fmt::format("{0}\n{1}",
|
||||
til::u16u8(appLogic->ApplicationDisplayName()),
|
||||
til::u16u8(appLogic->ApplicationVersion()));
|
||||
// Theoretically, we don't need to exit now, since this isn't really
|
||||
// an error case. However, in practice, it feels weird to have `wt
|
||||
// -v` open a new tab, and makes enough sense that `wt -v ;
|
||||
// split-pane` (or whatever) just displays the version and exits.
|
||||
_shouldExitEarly = true;
|
||||
}
|
||||
};
|
||||
_app.add_flag_function("-v,--version", versionCallback, RS_A(L"CmdVersionDesc"));
|
||||
|
||||
_buildNewTabParser();
|
||||
_buildSplitPaneParser();
|
||||
_buildFocusTabParser();
|
||||
|
@ -540,6 +562,19 @@ const std::string& AppCommandlineArgs::GetExitMessage()
|
|||
return _exitMessage;
|
||||
}
|
||||
|
||||
// Method Description:
|
||||
// - Returns true if we should exit the application before even starting the
|
||||
// window. We might want to do this if we're displaying an error message or
|
||||
// the version string, or if we want to open the settings file.
|
||||
// Arguments:
|
||||
// - <none>
|
||||
// Return Value:
|
||||
// - true iff we should exit the application before even starting the window
|
||||
bool AppCommandlineArgs::ShouldExitEarly() const noexcept
|
||||
{
|
||||
return _shouldExitEarly;
|
||||
}
|
||||
|
||||
// Method Description:
|
||||
// - Ensure that the first command in our list of actions is a NewTab action.
|
||||
// This makes sure that if the user passes a commandline like "wt split-pane
|
||||
|
|
|
@ -36,6 +36,7 @@ public:
|
|||
void ValidateStartupCommands();
|
||||
std::deque<winrt::TerminalApp::ActionAndArgs>& GetStartupActions();
|
||||
const std::string& GetExitMessage();
|
||||
bool ShouldExitEarly() const noexcept;
|
||||
|
||||
private:
|
||||
static const std::wregex _commandDelimiterRegex;
|
||||
|
@ -79,6 +80,7 @@ private:
|
|||
|
||||
std::deque<winrt::TerminalApp::ActionAndArgs> _startupActions;
|
||||
std::string _exitMessage;
|
||||
bool _shouldExitEarly{ false };
|
||||
|
||||
winrt::TerminalApp::NewTerminalArgs _getNewTerminalArgs(NewTerminalSubcommand& subcommand);
|
||||
void _addNewTerminalArgs(NewTerminalSubcommand& subcommand);
|
||||
|
|
|
@ -902,15 +902,24 @@ namespace winrt::TerminalApp::implementation
|
|||
return 0;
|
||||
}
|
||||
|
||||
winrt::hstring AppLogic::EarlyExitMessage()
|
||||
winrt::hstring AppLogic::ParseCommandlineMessage()
|
||||
{
|
||||
if (_root)
|
||||
{
|
||||
return _root->EarlyExitMessage();
|
||||
return _root->ParseCommandlineMessage();
|
||||
}
|
||||
return { L"" };
|
||||
}
|
||||
|
||||
bool AppLogic::ShouldExitEarly()
|
||||
{
|
||||
if (_root)
|
||||
{
|
||||
return _root->ShouldExitEarly();
|
||||
}
|
||||
return false;
|
||||
}
|
||||
|
||||
winrt::hstring AppLogic::ApplicationDisplayName() const
|
||||
{
|
||||
try
|
||||
|
|
|
@ -28,7 +28,8 @@ namespace winrt::TerminalApp::implementation
|
|||
[[nodiscard]] std::shared_ptr<::TerminalApp::CascadiaSettings> GetSettings() const noexcept;
|
||||
|
||||
int32_t SetStartupCommandline(array_view<const winrt::hstring> actions);
|
||||
winrt::hstring EarlyExitMessage();
|
||||
winrt::hstring ParseCommandlineMessage();
|
||||
bool ShouldExitEarly();
|
||||
|
||||
winrt::hstring ApplicationDisplayName() const;
|
||||
winrt::hstring ApplicationVersion() const;
|
||||
|
|
|
@ -13,7 +13,8 @@ namespace TerminalApp
|
|||
MaximizedMode,
|
||||
};
|
||||
|
||||
[default_interface] runtimeclass AppLogic: IF7Listener {
|
||||
[default_interface] runtimeclass AppLogic : IF7Listener
|
||||
{
|
||||
AppLogic();
|
||||
|
||||
// For your own sanity, it's better to do setup outside the ctor.
|
||||
|
@ -28,7 +29,8 @@ namespace TerminalApp
|
|||
Boolean IsElevated();
|
||||
|
||||
Int32 SetStartupCommandline(String[] commands);
|
||||
String EarlyExitMessage { get; };
|
||||
String ParseCommandlineMessage { get; };
|
||||
Boolean ShouldExitEarly { get; };
|
||||
|
||||
void LoadSettings();
|
||||
Windows.UI.Xaml.UIElement GetRoot();
|
||||
|
|
|
@ -232,6 +232,9 @@
|
|||
<value>Open in the given directory instead of the profile's set "startingDirectory"</value>
|
||||
<comment>{Locked="\"startingDirectory\""}</comment>
|
||||
</data>
|
||||
<data name="CmdVersionDesc" xml:space="preserve">
|
||||
<value>Display the application version</value>
|
||||
</data>
|
||||
<data name="NewTabSplitButton.[using:Windows.UI.Xaml.Automation]AutomationProperties.HelpText" xml:space="preserve">
|
||||
<value>Press the button to open a new terminal tab with your default profile. Open the flyout to select which profile you want to open.</value>
|
||||
</data>
|
||||
|
|
|
@ -1739,16 +1739,31 @@ namespace winrt::TerminalApp::implementation
|
|||
// message. If there were no errors, this message will be blank.
|
||||
// - If the user requested help on any command (using --help), this will
|
||||
// contain the help message.
|
||||
// - If the user requested the version number (using --version), this will
|
||||
// contain the version string.
|
||||
// Arguments:
|
||||
// - <none>
|
||||
// Return Value:
|
||||
// - the help text or error message for the provided commandline, if one
|
||||
// exists, otherwise the empty string.
|
||||
winrt::hstring TerminalPage::EarlyExitMessage()
|
||||
winrt::hstring TerminalPage::ParseCommandlineMessage()
|
||||
{
|
||||
return winrt::to_hstring(_appArgs.GetExitMessage());
|
||||
}
|
||||
|
||||
// Method Description:
|
||||
// - Returns true if we should exit the application before even starting the
|
||||
// window. We might want to do this if we're displaying an error message or
|
||||
// the version string, or if we want to open the settings file.
|
||||
// Arguments:
|
||||
// - <none>
|
||||
// Return Value:
|
||||
// - true iff we should exit the application before even starting the window
|
||||
bool TerminalPage::ShouldExitEarly()
|
||||
{
|
||||
return _appArgs.ShouldExitEarly();
|
||||
}
|
||||
|
||||
// Method Description:
|
||||
// - Returns a com_ptr to the implementation type of the tab at the given index
|
||||
// Arguments:
|
||||
|
|
|
@ -50,7 +50,8 @@ namespace winrt::TerminalApp::implementation
|
|||
void CloseWindow();
|
||||
|
||||
int32_t SetStartupCommandline(winrt::array_view<const hstring> args);
|
||||
winrt::hstring EarlyExitMessage();
|
||||
winrt::hstring ParseCommandlineMessage();
|
||||
bool ShouldExitEarly();
|
||||
|
||||
// -------------------------------- WinRT Events ---------------------------------
|
||||
DECLARE_EVENT_WITH_TYPED_EVENT_HANDLER(TitleChanged, _titleChangeHandlers, winrt::Windows::Foundation::IInspectable, winrt::hstring);
|
||||
|
|
|
@ -11,7 +11,8 @@ namespace TerminalApp
|
|||
TerminalPage();
|
||||
|
||||
Int32 SetStartupCommandline(String[] commands);
|
||||
String EarlyExitMessage { get; };
|
||||
String ParseCommandlineMessage { get; };
|
||||
Boolean ShouldExitEarly { get; };
|
||||
|
||||
// XAML bound properties
|
||||
String ApplicationDisplayName { get; };
|
||||
|
|
|
@ -102,7 +102,7 @@ void AppHost::_HandleCommandlineArgs()
|
|||
}
|
||||
|
||||
const auto result = _logic.SetStartupCommandline({ args });
|
||||
const auto message = _logic.EarlyExitMessage();
|
||||
const auto message = _logic.ParseCommandlineMessage();
|
||||
if (!message.empty())
|
||||
{
|
||||
const auto displayHelp = result == 0;
|
||||
|
@ -115,7 +115,10 @@ void AppHost::_HandleCommandlineArgs()
|
|||
GetStringResource(messageTitle).data(),
|
||||
MB_OK | messageIcon);
|
||||
|
||||
ExitProcess(result);
|
||||
if (_logic.ShouldExitEarly())
|
||||
{
|
||||
ExitProcess(result);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
|
Loading…
Reference in a new issue