## Summary of the Pull Request This is a follow up to #9300. Now that we have names on our windows, it would be nice to see who is named what. So this adds two actions: * `identifyWindow`: This action will pop up a little toast (#8592) displaying the name and ID of the window, and is bound by default. 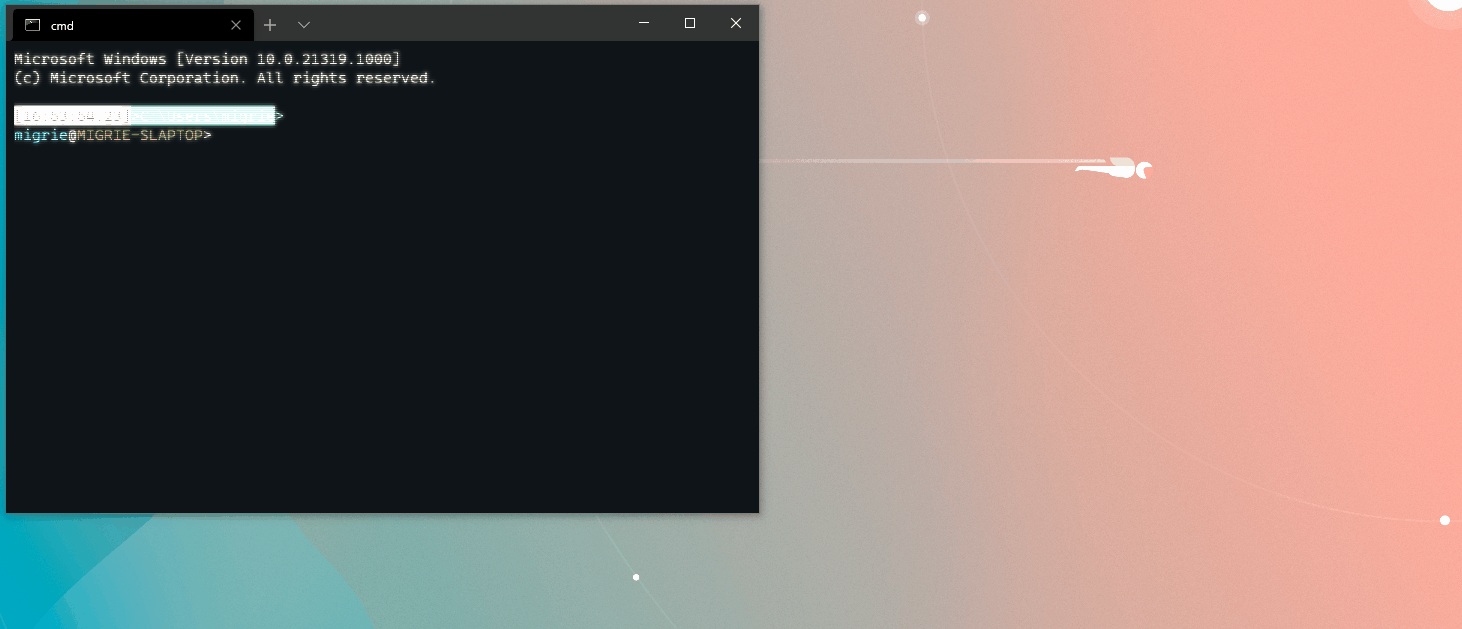 * `identifyWindows`: This action will request that ALL windows pop up that toast. This is meant to feel like the "Identify" button on the Windows display settings. However, sometimes, it's wonky. 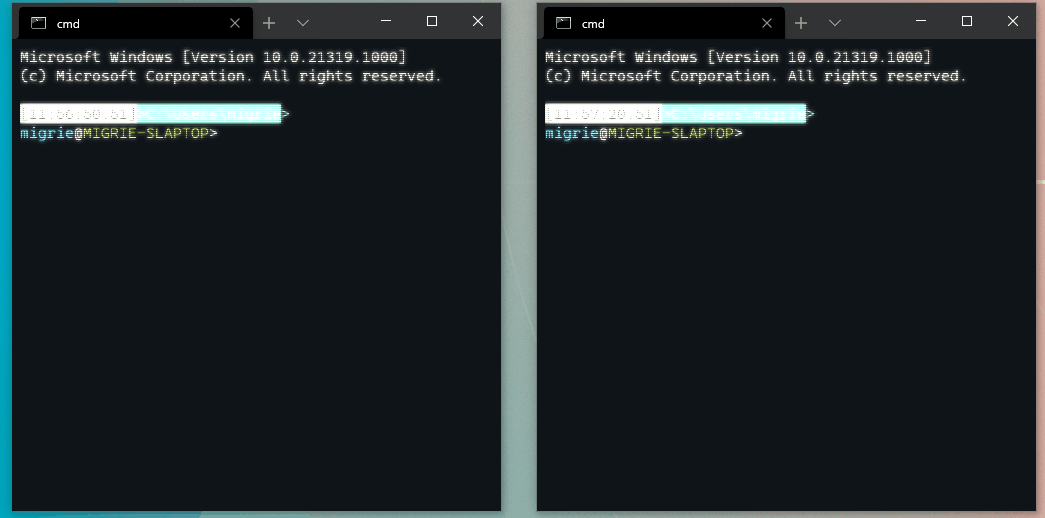 That's being tracked upstream on https://github.com/microsoft/microsoft-ui-xaml/issues/4382 Because it's so wonky, we won't bind that by default. Maybe if we get that fixed, then we'll change the default binding from `identifyWindow` to `identifyWindows` ## References ## PR Checklist * [x] Closes https://github.com/microsoft/terminal/projects/5#card-51431492 * [x] I work here * [x] Tests added/passed * [ ] Requires documentation to be updated ## Detailed Description of the Pull Request / Additional comments You may note that there are some macros to make interacting with lots and lots of actions easier. There's a lot of boilerplate whenever you need to make a new action, so I thought: "Can we make that easier?" Turns out you can make it a _LOT_ easier, but that work is still behind another PR after this one. Get excited
86 lines
2.8 KiB
C++
86 lines
2.8 KiB
C++
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
#pragma once
|
|
|
|
#include "ShortcutActionDispatch.g.h"
|
|
#include "../inc/cppwinrt_utils.h"
|
|
|
|
// fwdecl unittest classes
|
|
namespace TerminalAppLocalTests
|
|
{
|
|
class SettingsTests;
|
|
class KeyBindingsTests;
|
|
}
|
|
|
|
#define DECLARE_ACTION(action) TYPED_EVENT(action, TerminalApp::ShortcutActionDispatch, Microsoft::Terminal::Settings::Model::ActionEventArgs);
|
|
|
|
namespace winrt::TerminalApp::implementation
|
|
{
|
|
struct ShortcutActionDispatch : ShortcutActionDispatchT<ShortcutActionDispatch>
|
|
{
|
|
ShortcutActionDispatch() = default;
|
|
|
|
bool DoAction(const Microsoft::Terminal::Settings::Model::ActionAndArgs& actionAndArgs);
|
|
|
|
// clang-format off
|
|
DECLARE_ACTION(CopyText);
|
|
DECLARE_ACTION(PasteText);
|
|
DECLARE_ACTION(OpenNewTabDropdown);
|
|
DECLARE_ACTION(DuplicateTab);
|
|
DECLARE_ACTION(NewTab);
|
|
DECLARE_ACTION(CloseWindow);
|
|
DECLARE_ACTION(CloseTab);
|
|
DECLARE_ACTION(ClosePane);
|
|
DECLARE_ACTION(SwitchToTab);
|
|
DECLARE_ACTION(NextTab);
|
|
DECLARE_ACTION(PrevTab);
|
|
DECLARE_ACTION(SendInput);
|
|
DECLARE_ACTION(SplitPane);
|
|
DECLARE_ACTION(TogglePaneZoom);
|
|
DECLARE_ACTION(AdjustFontSize);
|
|
DECLARE_ACTION(ResetFontSize);
|
|
DECLARE_ACTION(ScrollUp);
|
|
DECLARE_ACTION(ScrollDown);
|
|
DECLARE_ACTION(ScrollUpPage);
|
|
DECLARE_ACTION(ScrollDownPage);
|
|
DECLARE_ACTION(ScrollToTop);
|
|
DECLARE_ACTION(ScrollToBottom);
|
|
DECLARE_ACTION(OpenSettings);
|
|
DECLARE_ACTION(ResizePane);
|
|
DECLARE_ACTION(Find);
|
|
DECLARE_ACTION(MoveFocus);
|
|
DECLARE_ACTION(ToggleShaderEffects);
|
|
DECLARE_ACTION(ToggleFocusMode);
|
|
DECLARE_ACTION(ToggleFullscreen);
|
|
DECLARE_ACTION(ToggleAlwaysOnTop);
|
|
DECLARE_ACTION(ToggleCommandPalette);
|
|
DECLARE_ACTION(SetColorScheme);
|
|
DECLARE_ACTION(SetTabColor);
|
|
DECLARE_ACTION(OpenTabColorPicker);
|
|
DECLARE_ACTION(RenameTab);
|
|
DECLARE_ACTION(OpenTabRenamer);
|
|
DECLARE_ACTION(ExecuteCommandline);
|
|
DECLARE_ACTION(CloseOtherTabs);
|
|
DECLARE_ACTION(CloseTabsAfter);
|
|
DECLARE_ACTION(TabSearch);
|
|
DECLARE_ACTION(MoveTab);
|
|
DECLARE_ACTION(BreakIntoDebugger);
|
|
DECLARE_ACTION(FindMatch);
|
|
DECLARE_ACTION(TogglePaneReadOnly);
|
|
DECLARE_ACTION(NewWindow);
|
|
DECLARE_ACTION(IdentifyWindow);
|
|
DECLARE_ACTION(IdentifyWindows);
|
|
// clang-format on
|
|
|
|
private:
|
|
friend class TerminalAppLocalTests::SettingsTests;
|
|
friend class TerminalAppLocalTests::KeyBindingsTests;
|
|
};
|
|
}
|
|
|
|
namespace winrt::TerminalApp::factory_implementation
|
|
{
|
|
BASIC_FACTORY(ShortcutActionDispatch);
|
|
}
|