## Summary of the Pull Request **If you're reading this PR and haven't signed off on #8135, go there first.** 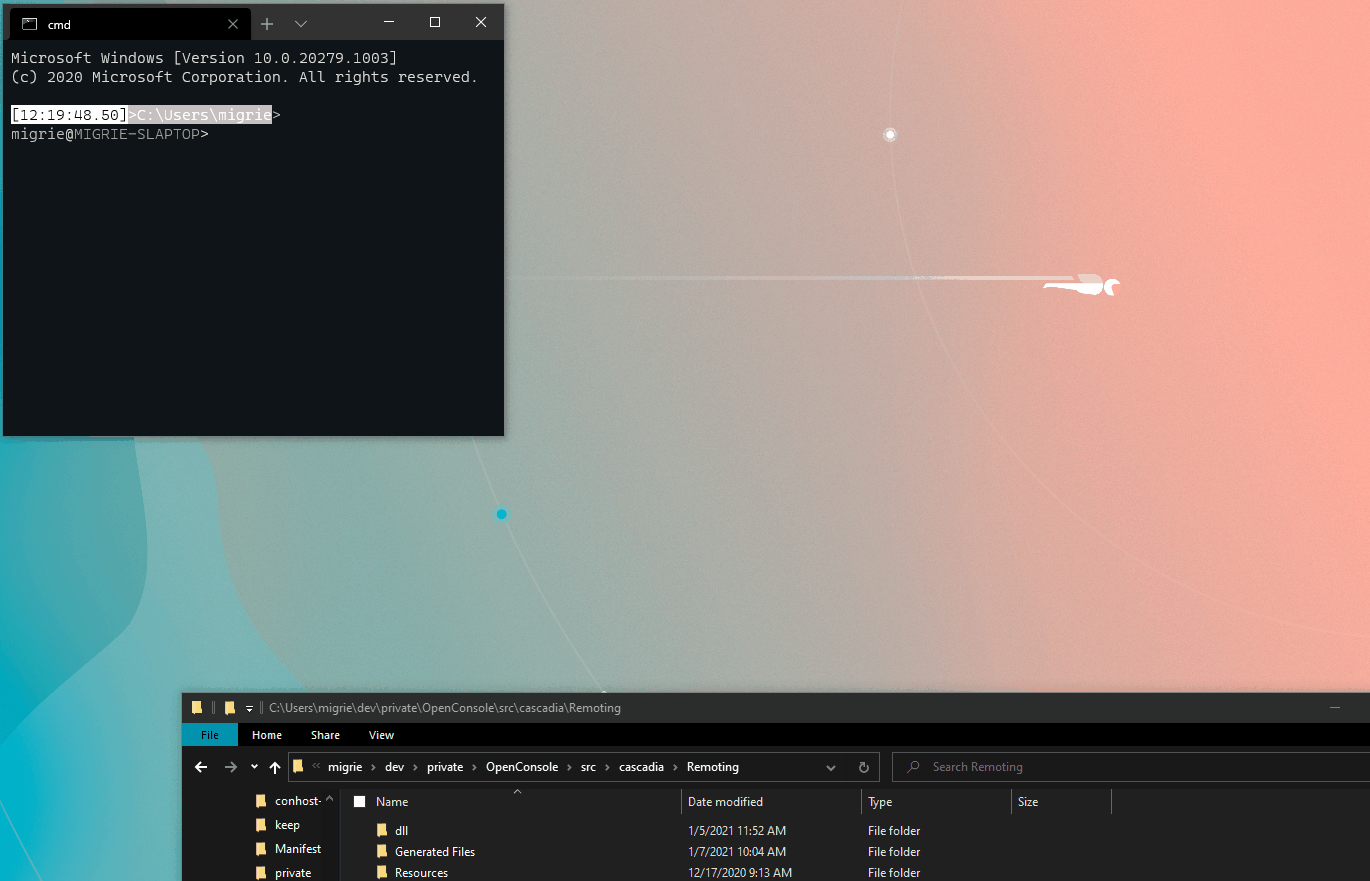 This provides the basic parts of the implementation of #4472. Namely: * We add support for the `--window,-w <window-id>` argument to `wt.exe`, to allow a commandline to be given to another window. * If `window-id` is `0`, run the given commands in _the current window_. * If `window-id` is a negative number, run the commands in a _new_ Terminal window. * If `window-id` is the ID of an existing window, then run the commandline in that window. * If `window-id` is _not_ the ID of an existing window, create a new window. That window will be assigned the ID provided in the commandline. The provided subcommands will be run in that new window. * If `window-id` is omitted, then create a new window. ## References * Spec: #8135 * Megathread: #5000 * Project: projects/5 ## PR Checklist * [x] Closes #4472 * [x] I work here * [x] Tests added/passed * [ ] Requires documentation to be updated - **sure does** ## Detailed Description of the Pull Request / Additional comments Note that `wt -w 1 -d c:\foo cmd.exe` does work, by causing window 1 to change There are limitations, and there are plenty of things to work on in the future: * [ ] We don't support names for windows yet * [ ] We don't support window glomming by default, or a setting to configure what happens when `-w` is omitted. I thought it best to lay the groundwork first, then come back to that. * [ ] `-w 0` currently just uses the "last activated" window, not "the current". There's more follow-up work to try and smartly find the actual window we're being called from. * [ ] Basically anything else that's listed in projects/5. I'm cutting this PR where it currently is, because this is already a huge PR. I believe the remaining tasks will all be easier to land, once this is in. ## Validation Steps Performed I've been creating windows, and closing them, and running cmdlines for a while now. I'm gonna keep doing that while the PR is open, till no bugs remain. # TODOs * [x] There are a bunch of `GetID`, `GetPID` calls that aren't try/caught 😬 - [x] `Monarch.cpp` - [x] `Peasant.cpp` - [x] `WindowManager.cpp` - [x] `AppHost.cpp` * [x] If the monarch gets hung, then _you can't launch any Terminals_ 😨 We should handle this gracefully. - Proposed idea: give the Monarch some time to respond to a proposal for a commandline. If there's no response in that timeframe, this window is now a _hermit_, outside of society entirely. It can't be elected Monarch. It can't receive command lines. It has no ID. - Could we gracefully recover from such a state? maybe, probably not though. - Same deal if a peasant hangs, it could end up hanging the monarch, right? Like if you do `wt -w 2`, and `2` is hung, then does the monarch get hung waiting on the hung peasant? - After talking with @miniksa, **we're gonna punt this from the initial implementation**. If people legit hit this in the wild, we'll fix it then.
86 lines
2.6 KiB
C++
86 lines
2.6 KiB
C++
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
#pragma once
|
|
|
|
#include "Monarch.g.h"
|
|
#include "Peasant.h"
|
|
#include "../cascadia/inc/cppwinrt_utils.h"
|
|
|
|
// We sure different GUIDs here depending on whether we're running a Release,
|
|
// Preview, or Dev build. This ensures that different installs don't
|
|
// accidentally talk to one another.
|
|
//
|
|
// * Release: {06171993-7eb1-4f3e-85f5-8bdd7386cce3}
|
|
// * Preview: {04221993-7eb1-4f3e-85f5-8bdd7386cce3}
|
|
// * Dev: {08302020-7eb1-4f3e-85f5-8bdd7386cce3}
|
|
constexpr GUID Monarch_clsid
|
|
{
|
|
#if defined(WT_BRANDING_RELEASE)
|
|
0x06171993,
|
|
#elif defined(WT_BRANDING_PREVIEW)
|
|
0x04221993,
|
|
#else
|
|
0x08302020,
|
|
#endif
|
|
0x7eb1,
|
|
0x4f3e,
|
|
{
|
|
0x85, 0xf5, 0x8b, 0xdd, 0x73, 0x86, 0xcc, 0xe3
|
|
}
|
|
};
|
|
|
|
enum class WindowingBehavior : uint64_t
|
|
{
|
|
UseNew = 0,
|
|
UseExisting = 1,
|
|
};
|
|
|
|
namespace RemotingUnitTests
|
|
{
|
|
class RemotingTests;
|
|
};
|
|
|
|
namespace winrt::Microsoft::Terminal::Remoting::implementation
|
|
{
|
|
struct Monarch : public MonarchT<Monarch>
|
|
{
|
|
Monarch();
|
|
~Monarch();
|
|
|
|
uint64_t GetPID();
|
|
|
|
uint64_t AddPeasant(winrt::Microsoft::Terminal::Remoting::IPeasant peasant);
|
|
|
|
winrt::Microsoft::Terminal::Remoting::ProposeCommandlineResult ProposeCommandline(const winrt::Microsoft::Terminal::Remoting::CommandlineArgs& args);
|
|
void HandleActivatePeasant(const winrt::Microsoft::Terminal::Remoting::WindowActivatedArgs& args);
|
|
|
|
TYPED_EVENT(FindTargetWindowRequested, winrt::Windows::Foundation::IInspectable, winrt::Microsoft::Terminal::Remoting::FindTargetWindowArgs);
|
|
|
|
private:
|
|
Monarch(const uint64_t testPID);
|
|
uint64_t _ourPID;
|
|
|
|
uint64_t _nextPeasantID{ 1 };
|
|
uint64_t _thisPeasantID{ 0 };
|
|
uint64_t _mostRecentPeasant{ 0 };
|
|
winrt::Windows::Foundation::DateTime _lastActivatedTime{};
|
|
|
|
WindowingBehavior _windowingBehavior{ WindowingBehavior::UseNew };
|
|
std::unordered_map<uint64_t, winrt::Microsoft::Terminal::Remoting::IPeasant> _peasants;
|
|
|
|
winrt::Microsoft::Terminal::Remoting::IPeasant _getPeasant(uint64_t peasantID);
|
|
uint64_t _getMostRecentPeasantID();
|
|
|
|
void _peasantWindowActivated(const winrt::Windows::Foundation::IInspectable& sender,
|
|
const winrt::Microsoft::Terminal::Remoting::WindowActivatedArgs& args);
|
|
|
|
friend class RemotingUnitTests::RemotingTests;
|
|
};
|
|
}
|
|
|
|
namespace winrt::Microsoft::Terminal::Remoting::factory_implementation
|
|
{
|
|
BASIC_FACTORY(Monarch);
|
|
}
|