## Summary of the Pull Request
Add support for "focus" mode, which only displays the actual terminal content, no tabs or titlebar. The edges of the window are draggable to resize, but the window can't be moved in borderless mode.
The window looks _slightly_ different bewteen different values for `showTabsInTitlebar`, because switching between the `NonClientIslandWindow` and the `IslandWindow` is _hard_.
`showTabsInTitlebar` | Preview
-- | --
`true` | 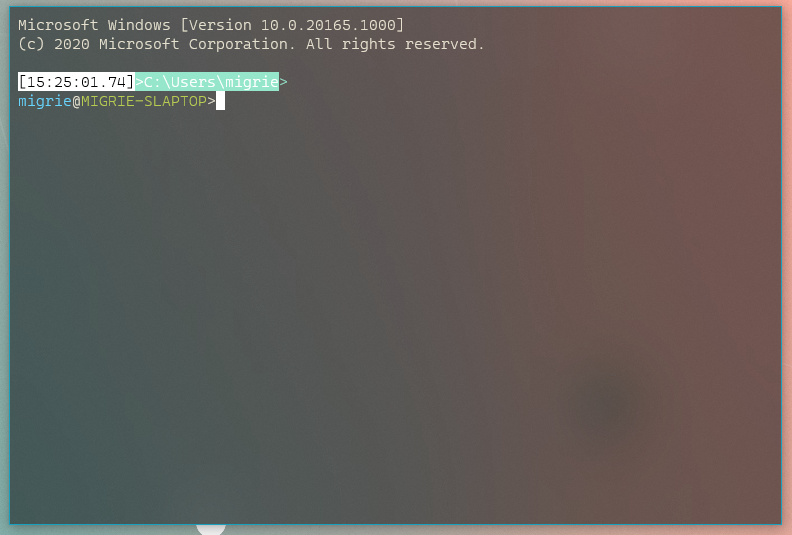
`false` | 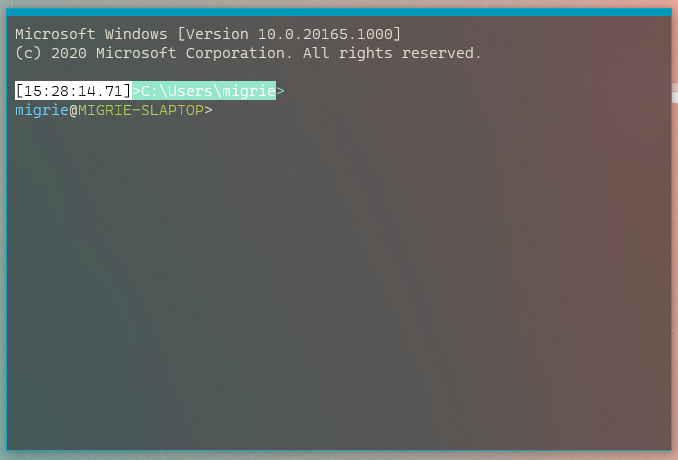
## PR Checklist
* [x] Closes #2238
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## Detailed Description of the Pull Request / Additional comments
* **KNOWN ISSUE**: Upon resizing the NCIW, the top frame margin disappears, making that border disappear entirely. 6356aaf
has a bunch of WIP work for me trying to fix that, but I couldn't get it quite right.
## Validation Steps Performed
* Toggled between focus and fullscreen a _bunch_ in both modes.
65 lines
2.5 KiB
Plaintext
65 lines
2.5 KiB
Plaintext
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
import "../TerminalPage.idl";
|
|
import "../ShortcutActionDispatch.idl";
|
|
import "../IDirectKeyListener.idl";
|
|
|
|
namespace TerminalApp
|
|
{
|
|
enum LaunchMode
|
|
{
|
|
DefaultMode,
|
|
MaximizedMode,
|
|
FullscreenMode,
|
|
};
|
|
|
|
[default_interface] runtimeclass AppLogic : IDirectKeyListener, IDialogPresenter
|
|
{
|
|
AppLogic();
|
|
|
|
// For your own sanity, it's better to do setup outside the ctor.
|
|
// If you do any setup in the ctor that ends up throwing an exception,
|
|
// then it might look like TermApp just failed to activate, which will
|
|
// cause you to chase down the rabbit hole of "why is TermApp not
|
|
// registered?" when it definitely is.
|
|
void Create();
|
|
|
|
Boolean IsUwp();
|
|
void RunAsUwp();
|
|
Boolean IsElevated();
|
|
|
|
Int32 SetStartupCommandline(String[] commands);
|
|
String ParseCommandlineMessage { get; };
|
|
Boolean ShouldExitEarly { get; };
|
|
|
|
void LoadSettings();
|
|
Windows.UI.Xaml.UIElement GetRoot();
|
|
|
|
String Title { get; };
|
|
|
|
String ApplicationDisplayName { get; };
|
|
String ApplicationVersion { get; };
|
|
|
|
Windows.Foundation.Point GetLaunchDimensions(UInt32 dpi);
|
|
Windows.Foundation.Point GetLaunchInitialPositions(Int32 defaultInitialX, Int32 defaultInitialY);
|
|
Windows.UI.Xaml.ElementTheme GetRequestedTheme();
|
|
LaunchMode GetLaunchMode();
|
|
Boolean GetShowTabsInTitlebar();
|
|
Single CalcSnappedDimension(Boolean widthOrHeight, Single dimension);
|
|
void TitlebarClicked();
|
|
void WindowCloseButtonClicked();
|
|
|
|
// See IDialogPresenter and TerminalPage's DialogPresenter for more
|
|
// information.
|
|
Windows.Foundation.IAsyncOperation<Windows.UI.Xaml.Controls.ContentDialogResult> ShowDialog(Windows.UI.Xaml.Controls.ContentDialog dialog);
|
|
|
|
event Windows.Foundation.TypedEventHandler<Object, Windows.UI.Xaml.UIElement> SetTitleBarContent;
|
|
event Windows.Foundation.TypedEventHandler<Object, String> TitleChanged;
|
|
event Windows.Foundation.TypedEventHandler<Object, LastTabClosedEventArgs> LastTabClosed;
|
|
event Windows.Foundation.TypedEventHandler<Object, Windows.UI.Xaml.ElementTheme> RequestedThemeChanged;
|
|
event Windows.Foundation.TypedEventHandler<Object, ToggleFullscreenEventArgs> ToggleFullscreen;
|
|
event Windows.Foundation.TypedEventHandler<Object, ToggleFocusModeEventArgs> ToggleFocusMode;
|
|
}
|
|
}
|