## Summary of the Pull Request 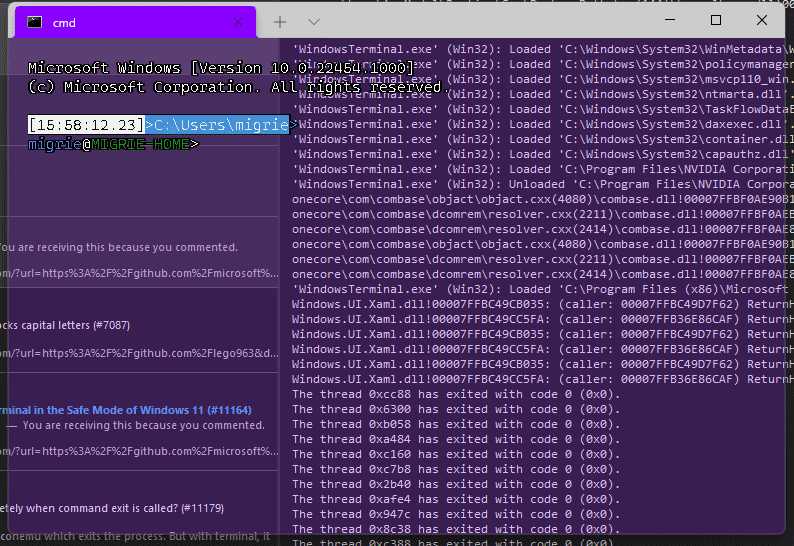 Adds support for vintage style opacity, on Windows 11+. The API we're using for this exists since the time immemorial, but there's a bug in XAML Islands that prevents it from working right until Windows 11 (which we're working on backporting). Replaces the `acrylicOpacity` setting with `opacity`, which is a uint between 0 and 100 (inclusive), default to 100. `useAcrylic` now controls whether acrylic is used or not. Setting an opacity < 100 with `"useAcrylic": false` will use vintage style opacity. Mouse wheeling adjusts opacity. Whether acrylic is used or not is dependent upon `useAcrylic`. `opacity` will stealthily default to 50 if `useAcrylic:true` is set. ## PR Checklist * [x] Closes #603 * [x] I work here * [x] Tests added/passed * [x] https://github.com/MicrosoftDocs/terminal/pull/416 ## Detailed Description of the Pull Request / Additional comments Opacity was moved to AppearanceConfig. In the future, I have a mind to allow unfocused acrylic, so that'll be important then. ## Validation Steps Performed _just look at it_
200 lines
9.3 KiB
C++
200 lines
9.3 KiB
C++
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
#pragma once
|
|
|
|
#include "Profiles.g.h"
|
|
#include "ProfilePageNavigationState.g.h"
|
|
#include "DeleteProfileEventArgs.g.h"
|
|
#include "ProfileViewModel.g.h"
|
|
#include "Utils.h"
|
|
#include "ViewModelHelpers.h"
|
|
#include "Appearances.h"
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Editor::implementation
|
|
{
|
|
struct ProfileViewModel : ProfileViewModelT<ProfileViewModel>, ViewModelHelper<ProfileViewModel>
|
|
{
|
|
public:
|
|
ProfileViewModel(const Model::Profile& profile, const Model::CascadiaSettings& settings);
|
|
|
|
Model::TerminalSettings TermSettings() const;
|
|
|
|
void SetAcrylicOpacityPercentageValue(double value)
|
|
{
|
|
_profile.DefaultAppearance().Opacity(winrt::Microsoft::Terminal::Settings::Editor::Converters::PercentageValueToPercentage(value));
|
|
};
|
|
|
|
void SetPadding(double value)
|
|
{
|
|
Padding(to_hstring(value));
|
|
}
|
|
|
|
// starting directory
|
|
bool UseParentProcessDirectory();
|
|
void UseParentProcessDirectory(const bool useParent);
|
|
bool UseCustomStartingDirectory();
|
|
|
|
// font face
|
|
static void UpdateFontList() noexcept;
|
|
Windows::Foundation::Collections::IObservableVector<Editor::Font> CompleteFontList() const noexcept;
|
|
Windows::Foundation::Collections::IObservableVector<Editor::Font> MonospaceFontList() const noexcept;
|
|
|
|
// general profile knowledge
|
|
winrt::guid OriginalProfileGuid() const noexcept;
|
|
bool CanDeleteProfile() const;
|
|
Editor::AppearanceViewModel DefaultAppearance();
|
|
Editor::AppearanceViewModel UnfocusedAppearance();
|
|
bool HasUnfocusedAppearance();
|
|
bool EditableUnfocusedAppearance();
|
|
bool ShowUnfocusedAppearance();
|
|
|
|
void CreateUnfocusedAppearance(const Windows::Foundation::Collections::IMapView<hstring, Model::ColorScheme>& schemes,
|
|
const IHostedInWindow& windowRoot);
|
|
void DeleteUnfocusedAppearance();
|
|
|
|
WINRT_PROPERTY(bool, IsBaseLayer, false);
|
|
|
|
PERMANENT_OBSERVABLE_PROJECTED_SETTING(_profile, Guid);
|
|
PERMANENT_OBSERVABLE_PROJECTED_SETTING(_profile, ConnectionType);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, Name);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, Source);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, Hidden);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, Icon);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, CloseOnExit);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, TabTitle);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, TabColor);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, SuppressApplicationTitle);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, UseAcrylic);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, ScrollState);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, Padding);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, Commandline);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, StartingDirectory);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, AntialiasingMode);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, ForceFullRepaintRendering);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, SoftwareRendering);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile.DefaultAppearance(), Foreground);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile.DefaultAppearance(), Background);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile.DefaultAppearance(), SelectionBackground);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile.DefaultAppearance(), CursorColor);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile.DefaultAppearance(), Opacity);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, HistorySize);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, SnapOnInput);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, AltGrAliasing);
|
|
OBSERVABLE_PROJECTED_SETTING(_profile, BellStyle);
|
|
|
|
private:
|
|
Model::Profile _profile;
|
|
winrt::guid _originalProfileGuid;
|
|
winrt::hstring _lastBgImagePath;
|
|
winrt::hstring _lastStartingDirectoryPath;
|
|
Editor::AppearanceViewModel _defaultAppearanceViewModel;
|
|
|
|
static Windows::Foundation::Collections::IObservableVector<Editor::Font> _MonospaceFontList;
|
|
static Windows::Foundation::Collections::IObservableVector<Editor::Font> _FontList;
|
|
|
|
static Editor::Font _GetFont(com_ptr<IDWriteLocalizedStrings> localizedFamilyNames);
|
|
|
|
Model::CascadiaSettings _appSettings;
|
|
Editor::AppearanceViewModel _unfocusedAppearanceViewModel;
|
|
};
|
|
|
|
struct DeleteProfileEventArgs :
|
|
public DeleteProfileEventArgsT<DeleteProfileEventArgs>
|
|
{
|
|
public:
|
|
DeleteProfileEventArgs(guid profileGuid) :
|
|
_ProfileGuid(profileGuid) {}
|
|
|
|
guid ProfileGuid() const noexcept { return _ProfileGuid; }
|
|
|
|
private:
|
|
guid _ProfileGuid{};
|
|
};
|
|
|
|
struct ProfilePageNavigationState : ProfilePageNavigationStateT<ProfilePageNavigationState>
|
|
{
|
|
public:
|
|
ProfilePageNavigationState(const Editor::ProfileViewModel& viewModel,
|
|
const Windows::Foundation::Collections::IMapView<hstring, Model::ColorScheme>& schemes,
|
|
const Editor::ProfilePageNavigationState& lastState,
|
|
const IHostedInWindow& windowRoot) :
|
|
_Profile{ viewModel },
|
|
_Schemes{ schemes },
|
|
_WindowRoot{ windowRoot }
|
|
{
|
|
// If there was a previous nav state copy the selected pivot from it.
|
|
if (lastState)
|
|
{
|
|
_LastActivePivot = lastState.LastActivePivot();
|
|
}
|
|
viewModel.DefaultAppearance().Schemes(schemes);
|
|
viewModel.DefaultAppearance().WindowRoot(windowRoot);
|
|
|
|
if (viewModel.UnfocusedAppearance())
|
|
{
|
|
viewModel.UnfocusedAppearance().Schemes(schemes);
|
|
viewModel.UnfocusedAppearance().WindowRoot(windowRoot);
|
|
}
|
|
}
|
|
|
|
void DeleteProfile();
|
|
void CreateUnfocusedAppearance();
|
|
void DeleteUnfocusedAppearance();
|
|
|
|
Windows::Foundation::Collections::IMapView<hstring, Model::ColorScheme> Schemes() { return _Schemes; }
|
|
void Schemes(const Windows::Foundation::Collections::IMapView<hstring, Model::ColorScheme>& val) { _Schemes = val; }
|
|
TYPED_EVENT(DeleteProfile, Editor::ProfilePageNavigationState, Editor::DeleteProfileEventArgs);
|
|
WINRT_PROPERTY(IHostedInWindow, WindowRoot, nullptr);
|
|
WINRT_PROPERTY(Editor::ProfilesPivots, LastActivePivot, Editor::ProfilesPivots::General);
|
|
WINRT_PROPERTY(Editor::ProfileViewModel, Profile, nullptr);
|
|
|
|
private:
|
|
Windows::Foundation::Collections::IMapView<hstring, Model::ColorScheme> _Schemes;
|
|
};
|
|
|
|
struct Profiles : public HasScrollViewer<Profiles>, ProfilesT<Profiles>
|
|
{
|
|
public:
|
|
Profiles();
|
|
|
|
void OnNavigatedTo(const Windows::UI::Xaml::Navigation::NavigationEventArgs& e);
|
|
void OnNavigatedFrom(const Windows::UI::Xaml::Navigation::NavigationEventArgs& e);
|
|
|
|
// bell style bits
|
|
bool IsBellStyleFlagSet(const uint32_t flag);
|
|
void SetBellStyleAudible(winrt::Windows::Foundation::IReference<bool> on);
|
|
void SetBellStyleWindow(winrt::Windows::Foundation::IReference<bool> on);
|
|
void SetBellStyleTaskbar(winrt::Windows::Foundation::IReference<bool> on);
|
|
|
|
fire_and_forget Commandline_Click(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
fire_and_forget StartingDirectory_Click(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
fire_and_forget Icon_Click(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void DeleteConfirmation_Click(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void Pivot_SelectionChanged(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void CreateUnfocusedAppearance_Click(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void DeleteUnfocusedAppearance_Click(Windows::Foundation::IInspectable const& sender, Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
|
|
WINRT_CALLBACK(PropertyChanged, Windows::UI::Xaml::Data::PropertyChangedEventHandler);
|
|
|
|
WINRT_PROPERTY(Editor::ProfilePageNavigationState, State, nullptr);
|
|
GETSET_BINDABLE_ENUM_SETTING(AntiAliasingMode, Microsoft::Terminal::Control::TextAntialiasingMode, State().Profile, AntialiasingMode);
|
|
GETSET_BINDABLE_ENUM_SETTING(CloseOnExitMode, Microsoft::Terminal::Settings::Model::CloseOnExitMode, State().Profile, CloseOnExit);
|
|
GETSET_BINDABLE_ENUM_SETTING(ScrollState, Microsoft::Terminal::Control::ScrollbarState, State().Profile, ScrollState);
|
|
|
|
private:
|
|
void _UpdateBIAlignmentControl(const int32_t val);
|
|
|
|
std::array<Windows::UI::Xaml::Controls::Primitives::ToggleButton, 9> _BIAlignmentButtons;
|
|
Windows::UI::Xaml::Data::INotifyPropertyChanged::PropertyChanged_revoker _ViewModelChangedRevoker;
|
|
Windows::UI::Xaml::Data::INotifyPropertyChanged::PropertyChanged_revoker _AppearanceViewModelChangedRevoker;
|
|
|
|
Microsoft::Terminal::Control::TermControl _previewControl;
|
|
};
|
|
};
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Editor::factory_implementation
|
|
{
|
|
BASIC_FACTORY(Profiles);
|
|
}
|