## Summary of the Pull Request 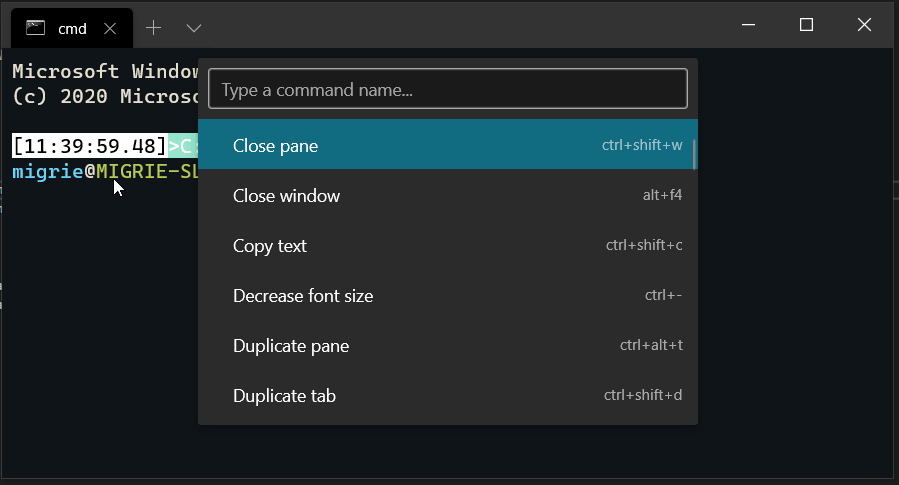 This adds a first iteration on the command palette. Notable missing features are: * Commandline mode: This will be a follow-up PR, following the merge of #6537 * nested and iterable commands: These will additionally be a follow-up PR. This is also additionally based off the addenda in #6532. This does not bind a key for the palette by default. That will be done when the above follow-ups are completed. ## References * #2046 - The original command palette thread * #5400 - This is the megathread for all command palette issues, which is tracking a bunch of additional follow up work * #5674 and #6532 - specs * #6537 - related ## PR Checklist * [x] Closes #2046 - incidentally also closes #6645 * [x] I work here * [x] Tests added/passed * [ ] Requires documentation to be updated - delaying this until it's more polished. ## Detailed Description of the Pull Request / Additional comments * There's a lot of code for autogenerating command names. That's all in `ActionArgs.cpp`, because each case is so _not_ boilerplate, unlike the rest of the code in `ActionArgs.h`. ## Validation Steps Performed * I've been playing with this for months. * Tests * Selfhost with the team
111 lines
3.8 KiB
C++
111 lines
3.8 KiB
C++
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
#include "pch.h"
|
|
#include "AppKeyBindings.h"
|
|
#include "KeyChordSerialization.h"
|
|
|
|
#include "AppKeyBindings.g.cpp"
|
|
|
|
using namespace winrt::Microsoft::Terminal;
|
|
using namespace winrt::TerminalApp;
|
|
using namespace winrt::Microsoft::Terminal::Settings;
|
|
|
|
namespace winrt::TerminalApp::implementation
|
|
{
|
|
void AppKeyBindings::SetKeyBinding(const TerminalApp::ActionAndArgs& actionAndArgs,
|
|
const Settings::KeyChord& chord)
|
|
{
|
|
_keyShortcuts[chord] = actionAndArgs;
|
|
}
|
|
|
|
// Method Description:
|
|
// - Remove the action that's bound to a particular KeyChord.
|
|
// Arguments:
|
|
// - chord: the keystroke to remove the action for.
|
|
// Return Value:
|
|
// - <none>
|
|
void AppKeyBindings::ClearKeyBinding(const Settings::KeyChord& chord)
|
|
{
|
|
_keyShortcuts.erase(chord);
|
|
}
|
|
|
|
KeyChord AppKeyBindings::GetKeyBindingForAction(TerminalApp::ShortcutAction const& action)
|
|
{
|
|
for (auto& kv : _keyShortcuts)
|
|
{
|
|
if (kv.second.Action() == action)
|
|
{
|
|
return kv.first;
|
|
}
|
|
}
|
|
return { nullptr };
|
|
}
|
|
|
|
// Method Description:
|
|
// - Lookup the keychord bound to a particular combination of ShortcutAction
|
|
// and IActionArgs. This enables searching no only for the binding of a
|
|
// particular ShortcutAction, but also a particular set of values for
|
|
// arguments to that action.
|
|
// Arguments:
|
|
// - actionAndArgs: The ActionAndArgs to lookup the keybinding for.
|
|
// Return Value:
|
|
// - The bound keychord, if this ActionAndArgs is bound to a key, otherwise nullptr.
|
|
KeyChord AppKeyBindings::GetKeyBindingForActionWithArgs(TerminalApp::ActionAndArgs const& actionAndArgs)
|
|
{
|
|
for (auto& kv : _keyShortcuts)
|
|
{
|
|
const auto action = kv.second.Action();
|
|
const auto args = kv.second.Args();
|
|
const auto actionMatched = action == actionAndArgs.Action();
|
|
const auto argsMatched = args ? args.Equals(actionAndArgs.Args()) : args == actionAndArgs.Args();
|
|
if (actionMatched && argsMatched)
|
|
{
|
|
return kv.first;
|
|
}
|
|
}
|
|
return { nullptr };
|
|
}
|
|
|
|
bool AppKeyBindings::TryKeyChord(const Settings::KeyChord& kc)
|
|
{
|
|
const auto keyIter = _keyShortcuts.find(kc);
|
|
if (keyIter != _keyShortcuts.end())
|
|
{
|
|
const auto actionAndArgs = keyIter->second;
|
|
return _dispatch.DoAction(actionAndArgs);
|
|
}
|
|
return false;
|
|
}
|
|
|
|
void AppKeyBindings::SetDispatch(const winrt::TerminalApp::ShortcutActionDispatch& dispatch)
|
|
{
|
|
_dispatch = dispatch;
|
|
}
|
|
|
|
// Method Description:
|
|
// - Takes the KeyModifier flags from Terminal and maps them to the WinRT types which are used by XAML
|
|
// Return Value:
|
|
// - a Windows::System::VirtualKeyModifiers object with the flags of which modifiers used.
|
|
Windows::System::VirtualKeyModifiers AppKeyBindings::ConvertVKModifiers(Settings::KeyModifiers modifiers)
|
|
{
|
|
Windows::System::VirtualKeyModifiers keyModifiers = Windows::System::VirtualKeyModifiers::None;
|
|
|
|
if (WI_IsFlagSet(modifiers, Settings::KeyModifiers::Ctrl))
|
|
{
|
|
keyModifiers |= Windows::System::VirtualKeyModifiers::Control;
|
|
}
|
|
if (WI_IsFlagSet(modifiers, Settings::KeyModifiers::Shift))
|
|
{
|
|
keyModifiers |= Windows::System::VirtualKeyModifiers::Shift;
|
|
}
|
|
if (WI_IsFlagSet(modifiers, Settings::KeyModifiers::Alt))
|
|
{
|
|
// note: Menu is the Alt VK_MENU
|
|
keyModifiers |= Windows::System::VirtualKeyModifiers::Menu;
|
|
}
|
|
|
|
return keyModifiers;
|
|
}
|
|
}
|