## Summary of the Pull Request Renderer: Add support for backoff and auto-disable on failed retry This commit introduces a backoff (150ms * number of tries) to the renderer's retry logic (introduced in #2830). It also changes the FAIL_FAST to a less globally-harmful render thread disable, so that we stop blowing up any application hosting a terminal when the graphics driver goes away. In addition, it adds a callback that a Renderer consumer can use to determine when the renderer _has_ failed, and a public method to kick it back into life. Fixes #5340. This PR also wires up TermControl so that it shows some UI when the renderer tastes clay. 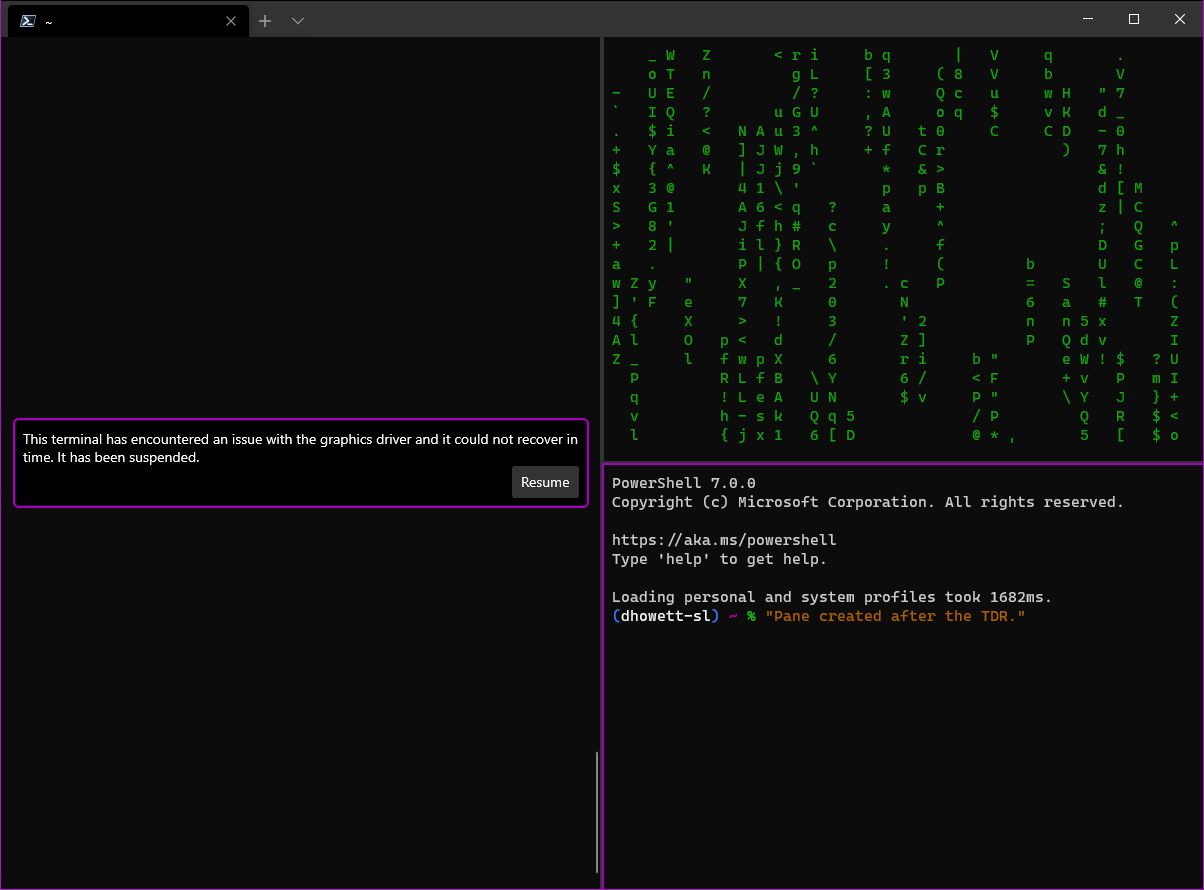 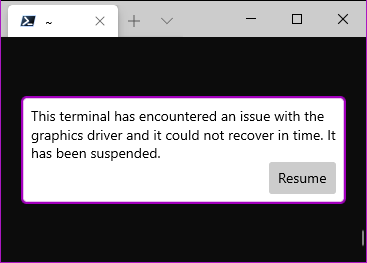 ## PR Checklist * [x] Closes #5340 * [x] cla * [ ] Tests added/passed * [ ] Requires documentation to be updated * [x] I've discussed this with core contributors already. ## Validation Steps Performed I tested this by dropping the number of retries to 1 and forcing a TDR while doing `wsl cmatrix -u0`. It picked up exactly where it left off. As a bonus, you can actually still type into the terminal when it's graphically suspended (and `exit` still works.). The block is _entirely graphical_.
38 lines
990 B
C++
38 lines
990 B
C++
/*++
|
|
Copyright (c) Microsoft Corporation
|
|
Licensed under the MIT license.
|
|
|
|
Module Name:
|
|
- IRenderThread.hpp
|
|
|
|
Abstract:
|
|
- an abstraction for all the actions a render thread needs to perform.
|
|
|
|
Author(s):
|
|
- Mike Griese (migrie) 16 Jan 2019
|
|
--*/
|
|
|
|
#pragma once
|
|
namespace Microsoft::Console::Render
|
|
{
|
|
class IRenderThread
|
|
{
|
|
public:
|
|
virtual ~IRenderThread() = 0;
|
|
IRenderThread(const IRenderThread&) = default;
|
|
IRenderThread(IRenderThread&&) = default;
|
|
IRenderThread& operator=(const IRenderThread&) = default;
|
|
IRenderThread& operator=(IRenderThread&&) = default;
|
|
|
|
virtual void NotifyPaint() = 0;
|
|
virtual void EnablePainting() = 0;
|
|
virtual void DisablePainting() = 0;
|
|
virtual void WaitForPaintCompletionAndDisable(const DWORD dwTimeoutMs) = 0;
|
|
|
|
protected:
|
|
IRenderThread() = default;
|
|
};
|
|
|
|
inline Microsoft::Console::Render::IRenderThread::~IRenderThread(){};
|
|
}
|