<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? --> ## Summary of the Pull Request Continuation of https://github.com/microsoft/terminal/pull/10972 to handle multiple windows, requires that to be merged first. <!-- Other than the issue solved, is this relevant to any other issues/existing PRs? --> ## References <!-- Please review the items on the PR checklist before submitting--> ## PR Checklist * [x] Also closes #766 * [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA * [ ] Tests added/passed * [ ] Documentation updated. If checked, please file a pull request on [our docs repo](https://github.com/MicrosoftDocs/terminal) and link it here: #xxx * [x] Schema updated. * [ ] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #xxx <!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here --> ## Detailed Description of the Pull Request / Additional comments Rough changelog: Normally saving is triggered to occur every 30s, or sooner if a window is created/closed. The existing behavior of saving on last close is maintained to bypass that throttling. The automatic saving allows for crash recovery. Additionally all window layouts will be saved upon taking the `quit` action. For loading we will check if we are the first window, that there are any saved layouts, and if the setting is enabled, and then depending on if we were given command line args or startup actions. - create a new window for each saved layout, or - take the first layout for our self and then a new window for each other layout. This also saves the layout when the quit action is taken. Misc changes - A -s,--saved argument was added to the command line to facilitate opening all of the windows with the right settings. This also means that while a terminal session is running you can do wt -s idx to open a copy of window idx. There isn't a stable ordering of which idx each window gets saved as (it is whatever the iteration order of _peasants is), so it is just a cute hack for now. - All position calculation has been moved up to AppHost this does mean we need to awkwardly pass around positions in a couple of unexpected places, but no solution was perfect. - Renamed "Open tabs from a previous session" to "Open windows from a previous session". (not reflected in video below) - Now save runtime tab color and window names - Only enabled for non-elevated windows - Add some change tracking to ApplicationState <!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well --> ## Validation Steps Performed 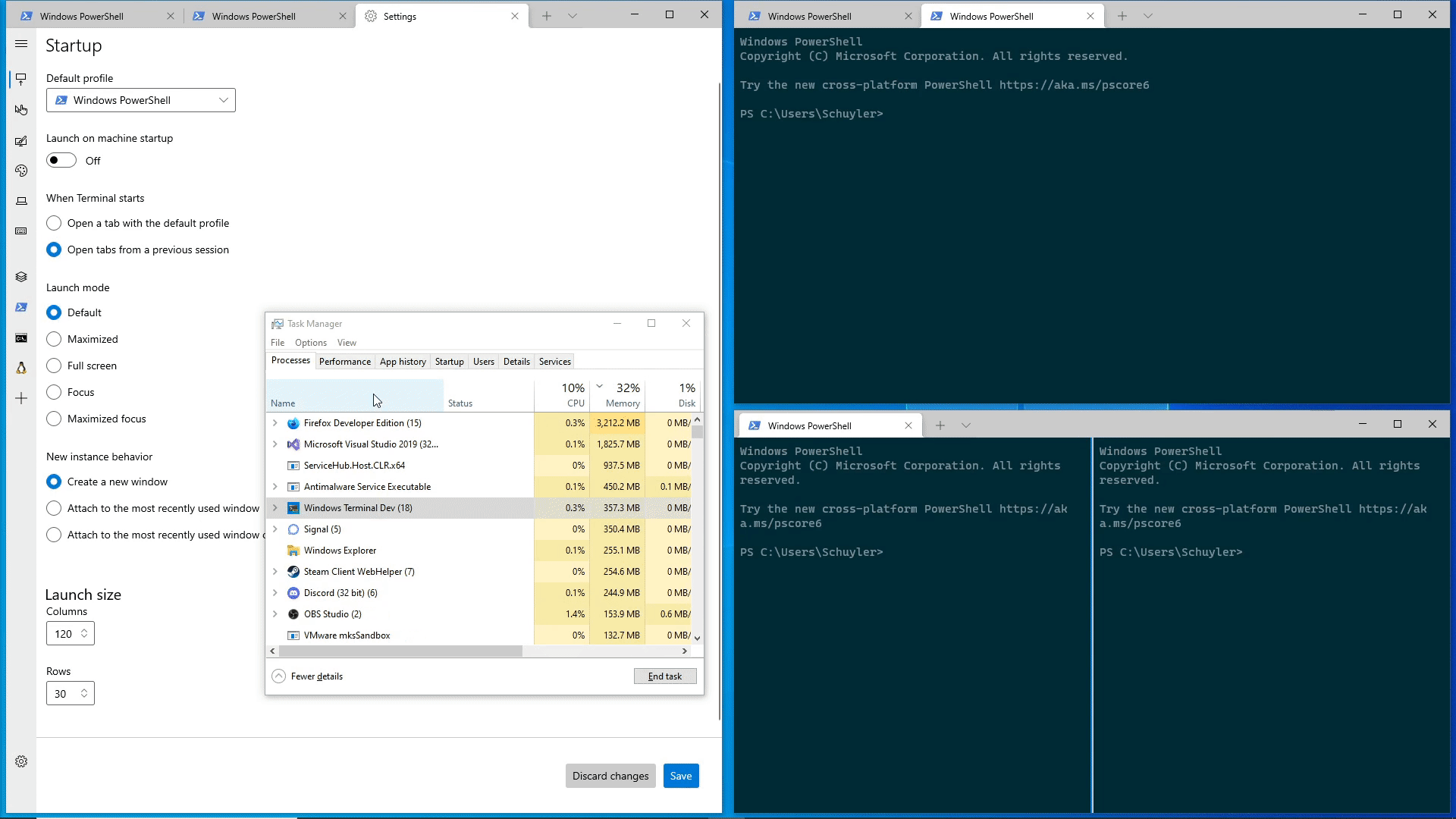
235 lines
10 KiB
C++
235 lines
10 KiB
C++
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
#include "pch.h"
|
|
#include "FileUtils.h"
|
|
|
|
#include <appmodel.h>
|
|
#include <shlobj.h>
|
|
#include <WtExeUtils.h>
|
|
|
|
static constexpr std::string_view Utf8Bom{ u8"\uFEFF" };
|
|
static constexpr std::wstring_view UnpackagedSettingsFolderName{ L"Microsoft\\Windows Terminal\\" };
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Model
|
|
{
|
|
// Returns a path like C:\Users\<username>\AppData\Local\Packages\<packagename>\LocalState
|
|
// You can put your settings.json or state.json in this directory.
|
|
std::filesystem::path GetBaseSettingsPath()
|
|
{
|
|
static std::filesystem::path baseSettingsPath = []() {
|
|
wil::unique_cotaskmem_string localAppDataFolder;
|
|
// KF_FLAG_FORCE_APP_DATA_REDIRECTION, when engaged, causes SHGet... to return
|
|
// the new AppModel paths (Packages/xxx/RoamingState, etc.) for standard path requests.
|
|
// Using this flag allows us to avoid Windows.Storage.ApplicationData completely.
|
|
THROW_IF_FAILED(SHGetKnownFolderPath(FOLDERID_LocalAppData, KF_FLAG_FORCE_APP_DATA_REDIRECTION, nullptr, &localAppDataFolder));
|
|
|
|
std::filesystem::path parentDirectoryForSettingsFile{ localAppDataFolder.get() };
|
|
|
|
if (!IsPackaged())
|
|
{
|
|
parentDirectoryForSettingsFile /= UnpackagedSettingsFolderName;
|
|
}
|
|
|
|
// Create the directory if it doesn't exist
|
|
std::filesystem::create_directories(parentDirectoryForSettingsFile);
|
|
|
|
return parentDirectoryForSettingsFile;
|
|
}();
|
|
return baseSettingsPath;
|
|
}
|
|
|
|
locked_hfile OpenFileReadSharedLocked(const std::filesystem::path& path)
|
|
{
|
|
wil::unique_hfile file{ CreateFileW(path.c_str(), GENERIC_READ, FILE_SHARE_READ | FILE_SHARE_WRITE, nullptr, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, nullptr) };
|
|
THROW_LAST_ERROR_IF(!file);
|
|
// just lock the entire file
|
|
OVERLAPPED sOverlapped;
|
|
sOverlapped.Offset = 0;
|
|
sOverlapped.OffsetHigh = 0;
|
|
// Shared lock
|
|
THROW_LAST_ERROR_IF(!LockFileEx(file.get(),
|
|
0, // lock shared, wait to return until lock is obtained
|
|
0, // reserved, does nothing
|
|
INT_MAX, // lock INT_MAX bytes
|
|
0, // higher-order bytes, if our state file is greater than 2GB I guess this will be a problem
|
|
&sOverlapped));
|
|
return { std::move(file), sOverlapped };
|
|
}
|
|
|
|
locked_hfile OpenFileRWExclusiveLocked(const std::filesystem::path& path)
|
|
{
|
|
wil::unique_hfile file{ CreateFileW(path.c_str(), GENERIC_READ | GENERIC_WRITE, FILE_SHARE_READ | FILE_SHARE_WRITE, nullptr, OPEN_ALWAYS, FILE_ATTRIBUTE_NORMAL, nullptr) };
|
|
THROW_LAST_ERROR_IF(!file);
|
|
// just lock the entire file
|
|
OVERLAPPED sOverlapped;
|
|
sOverlapped.Offset = 0;
|
|
sOverlapped.OffsetHigh = 0;
|
|
// Shared lock
|
|
THROW_LAST_ERROR_IF(!LockFileEx(file.get(),
|
|
LOCKFILE_EXCLUSIVE_LOCK, // lock exclusive, wait to return until lock is obtained
|
|
0, // reserved, does nothing
|
|
INT_MAX, // lock INT_MAX bytes
|
|
0, // higher-order bytes, if our state file is greater than 2GB I guess this will be a problem
|
|
&sOverlapped));
|
|
return { std::move(file), sOverlapped };
|
|
}
|
|
|
|
std::string ReadUTF8FileLocked(const locked_hfile& file)
|
|
{
|
|
const auto fileSize = GetFileSize(file.get(), nullptr);
|
|
THROW_LAST_ERROR_IF(fileSize == INVALID_FILE_SIZE);
|
|
|
|
// By making our buffer just slightly larger we can detect if
|
|
// the file size changed and we've failed to read the full file.
|
|
std::string buffer(static_cast<size_t>(fileSize) + 1, '\0');
|
|
DWORD bytesRead = 0;
|
|
THROW_IF_WIN32_BOOL_FALSE(ReadFile(file.get(), buffer.data(), gsl::narrow<DWORD>(buffer.size()), &bytesRead, nullptr));
|
|
|
|
// As mentioned before our buffer was allocated oversized.
|
|
buffer.resize(bytesRead);
|
|
|
|
if (til::starts_with(buffer, Utf8Bom))
|
|
{
|
|
// Yeah this memmove()s the entire content.
|
|
// But I don't really want to deal with UTF8 BOMs any more than necessary,
|
|
// as basically not a single editor writes a BOM for UTF8.
|
|
buffer.erase(0, Utf8Bom.size());
|
|
}
|
|
|
|
return buffer;
|
|
}
|
|
|
|
void WriteUTF8FileLocked(const locked_hfile& file, const std::string_view& content)
|
|
{
|
|
// truncate the file because we want to overwrite it
|
|
SetFilePointer(file.get(), 0, nullptr, FILE_BEGIN);
|
|
THROW_IF_WIN32_BOOL_FALSE(SetEndOfFile(file.get()));
|
|
|
|
const auto fileSize = gsl::narrow<DWORD>(content.size());
|
|
DWORD bytesWritten = 0;
|
|
THROW_IF_WIN32_BOOL_FALSE(WriteFile(file.get(), content.data(), fileSize, &bytesWritten, nullptr));
|
|
|
|
if (bytesWritten != fileSize)
|
|
{
|
|
THROW_WIN32_MSG(ERROR_WRITE_FAULT, "failed to write whole file");
|
|
}
|
|
}
|
|
|
|
// Tries to read a file somewhat atomically without locking it.
|
|
// Strips the UTF8 BOM if it exists.
|
|
std::string ReadUTF8File(const std::filesystem::path& path)
|
|
{
|
|
// From some casual observations we can determine that:
|
|
// * ReadFile() always returns the requested amount of data (unless the file is smaller)
|
|
// * It's unlikely that the file was changed between GetFileSize() and ReadFile()
|
|
// -> Lets add a retry-loop just in case, to not fail if the file size changed while reading.
|
|
for (int i = 0; i < 3; ++i)
|
|
{
|
|
wil::unique_hfile file{ CreateFileW(path.c_str(), GENERIC_READ, FILE_SHARE_READ | FILE_SHARE_WRITE, nullptr, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, nullptr) };
|
|
THROW_LAST_ERROR_IF(!file);
|
|
|
|
const auto fileSize = GetFileSize(file.get(), nullptr);
|
|
THROW_LAST_ERROR_IF(fileSize == INVALID_FILE_SIZE);
|
|
|
|
// By making our buffer just slightly larger we can detect if
|
|
// the file size changed and we've failed to read the full file.
|
|
std::string buffer(static_cast<size_t>(fileSize) + 1, '\0');
|
|
DWORD bytesRead = 0;
|
|
THROW_IF_WIN32_BOOL_FALSE(ReadFile(file.get(), buffer.data(), gsl::narrow<DWORD>(buffer.size()), &bytesRead, nullptr));
|
|
|
|
// This implementation isn't atomic as we'd need to use an exclusive file lock.
|
|
// But this would be annoying for users as it forces them to close the file in their editor.
|
|
// The next best alternative is to at least try to detect file changes and retry the read.
|
|
if (bytesRead != fileSize)
|
|
{
|
|
// This continue is unlikely to be hit (see the prior for loop comment).
|
|
std::this_thread::sleep_for(std::chrono::milliseconds(100));
|
|
continue;
|
|
}
|
|
|
|
// As mentioned before our buffer was allocated oversized.
|
|
buffer.resize(bytesRead);
|
|
|
|
if (til::starts_with(buffer, Utf8Bom))
|
|
{
|
|
// Yeah this memmove()s the entire content.
|
|
// But I don't really want to deal with UTF8 BOMs any more than necessary,
|
|
// as basically not a single editor writes a BOM for UTF8.
|
|
buffer.erase(0, Utf8Bom.size());
|
|
}
|
|
|
|
return buffer;
|
|
}
|
|
|
|
THROW_WIN32_MSG(ERROR_READ_FAULT, "file size changed while reading");
|
|
}
|
|
|
|
// Same as ReadUTF8File, but returns an empty optional, if the file couldn't be opened.
|
|
std::optional<std::string> ReadUTF8FileIfExists(const std::filesystem::path& path)
|
|
{
|
|
try
|
|
{
|
|
return { ReadUTF8File(path) };
|
|
}
|
|
catch (const wil::ResultException& exception)
|
|
{
|
|
if (exception.GetErrorCode() == HRESULT_FROM_WIN32(ERROR_FILE_NOT_FOUND))
|
|
{
|
|
return {};
|
|
}
|
|
|
|
throw;
|
|
}
|
|
}
|
|
|
|
void WriteUTF8File(const std::filesystem::path& path, const std::string_view& content)
|
|
{
|
|
wil::unique_hfile file{ CreateFileW(path.c_str(), GENERIC_WRITE, FILE_SHARE_READ | FILE_SHARE_WRITE, nullptr, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, nullptr) };
|
|
THROW_LAST_ERROR_IF(!file);
|
|
|
|
const auto fileSize = gsl::narrow<DWORD>(content.size());
|
|
DWORD bytesWritten = 0;
|
|
THROW_IF_WIN32_BOOL_FALSE(WriteFile(file.get(), content.data(), fileSize, &bytesWritten, nullptr));
|
|
|
|
if (bytesWritten != fileSize)
|
|
{
|
|
THROW_WIN32_MSG(ERROR_WRITE_FAULT, "failed to write whole file");
|
|
}
|
|
}
|
|
|
|
void WriteUTF8FileAtomic(const std::filesystem::path& path, const std::string_view& content)
|
|
{
|
|
// GH#10787: rename() will replace symbolic links themselves and not the path they point at.
|
|
// It's thus important that we first resolve them before generating temporary path.
|
|
std::error_code ec;
|
|
const auto resolvedPath = std::filesystem::is_symlink(path) ? std::filesystem::canonical(path, ec) : path;
|
|
if (ec)
|
|
{
|
|
if (ec.value() != ERROR_FILE_NOT_FOUND)
|
|
{
|
|
THROW_WIN32_MSG(ec.value(), "failed to compute canonical path");
|
|
}
|
|
|
|
// The original file is a symbolic link, but the target doesn't exist.
|
|
// Consider two fall-backs:
|
|
// * resolve the link manually, which might be less accurate and more prone to race conditions
|
|
// * write to the file directly, which lets the system resolve the symbolic link but leaves the write non-atomic
|
|
// The latter is chosen, as this is an edge case and our 'atomic' writes are only best-effort.
|
|
WriteUTF8File(path, content);
|
|
return;
|
|
}
|
|
|
|
auto tmpPath = resolvedPath;
|
|
tmpPath += L".tmp";
|
|
|
|
// Writing to a file isn't atomic, but...
|
|
WriteUTF8File(tmpPath, content);
|
|
|
|
// renaming one is (supposed to be) atomic.
|
|
// Wait... "supposed to be"!? Well it's technically not always atomic,
|
|
// but it's pretty darn close to it, so... better than nothing.
|
|
std::filesystem::rename(tmpPath, resolvedPath);
|
|
}
|
|
}
|