The flyout wasn't very polished, so I did some adjustments. It's all visual changes, functionality should be the same. * made the flyout use OverlayCornerRadius and 16px padding (to match WinUI 2.6) * changed ColorPicker to muxc:ColorPicker for new styles (the color schemes picker too) * changed "Custom" Button into a ToggleButton * no longer needs ellipsis - localization files should be updated * OK button was moved to the right and uses accent color * adjusted margins and padding * tweaked the color boxes to _look_ like the ones in color schemes 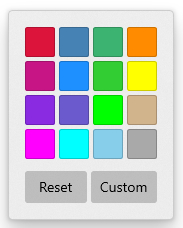 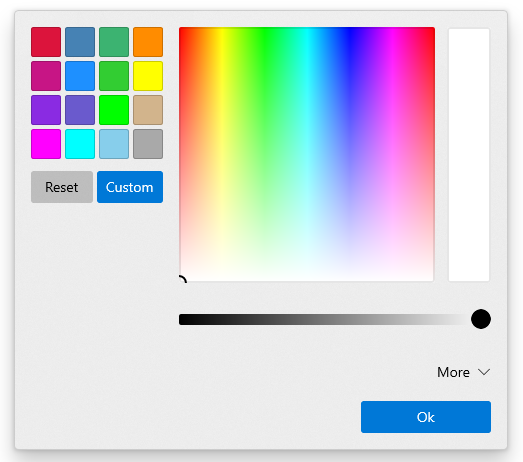 ## Validation Steps Performed * Color picker in settings UI still works ✔️ * Color picker for tabs still works ✔️
77 lines
4.1 KiB
C++
77 lines
4.1 KiB
C++
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
#pragma once
|
|
|
|
#include "ColorTableEntry.g.h"
|
|
#include "ColorSchemes.g.h"
|
|
#include "ColorSchemesPageNavigationState.g.h"
|
|
#include "Utils.h"
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Editor::implementation
|
|
{
|
|
struct ColorSchemesPageNavigationState : ColorSchemesPageNavigationStateT<ColorSchemesPageNavigationState>
|
|
{
|
|
public:
|
|
ColorSchemesPageNavigationState(const Model::CascadiaSettings& settings) :
|
|
_Settings{ settings } {}
|
|
|
|
WINRT_PROPERTY(Model::CascadiaSettings, Settings, nullptr);
|
|
WINRT_PROPERTY(winrt::hstring, LastSelectedScheme, L"");
|
|
};
|
|
|
|
struct ColorSchemes : ColorSchemesT<ColorSchemes>
|
|
{
|
|
ColorSchemes();
|
|
|
|
void OnNavigatedTo(const winrt::Windows::UI::Xaml::Navigation::NavigationEventArgs& e);
|
|
|
|
void ColorSchemeSelectionChanged(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::Controls::SelectionChangedEventArgs const& args);
|
|
void ColorPickerChanged(winrt::Windows::Foundation::IInspectable const& sender, winrt::Microsoft::UI::Xaml::Controls::ColorChangedEventArgs const& args);
|
|
void AddNew_Click(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
|
|
void Rename_Click(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void RenameAccept_Click(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void RenameCancel_Click(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
void NameBox_PreviewKeyDown(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::Input::KeyRoutedEventArgs const& e);
|
|
|
|
bool CanDeleteCurrentScheme() const;
|
|
void DeleteConfirmation_Click(winrt::Windows::Foundation::IInspectable const& sender, winrt::Windows::UI::Xaml::RoutedEventArgs const& e);
|
|
|
|
WINRT_PROPERTY(Editor::ColorSchemesPageNavigationState, State, nullptr);
|
|
WINRT_PROPERTY(Model::ColorScheme, CurrentColorScheme, nullptr);
|
|
WINRT_PROPERTY(Windows::Foundation::Collections::IVector<Editor::ColorTableEntry>, CurrentNonBrightColorTable, nullptr);
|
|
WINRT_PROPERTY(Windows::Foundation::Collections::IVector<Editor::ColorTableEntry>, CurrentBrightColorTable, nullptr);
|
|
WINRT_PROPERTY(Windows::Foundation::Collections::IObservableVector<Model::ColorScheme>, ColorSchemeList, nullptr);
|
|
|
|
WINRT_CALLBACK(PropertyChanged, Windows::UI::Xaml::Data::PropertyChangedEventHandler);
|
|
WINRT_OBSERVABLE_PROPERTY(bool, IsRenaming, _PropertyChangedHandlers, nullptr);
|
|
WINRT_OBSERVABLE_PROPERTY(Editor::ColorTableEntry, CurrentForegroundColor, _PropertyChangedHandlers, nullptr);
|
|
WINRT_OBSERVABLE_PROPERTY(Editor::ColorTableEntry, CurrentBackgroundColor, _PropertyChangedHandlers, nullptr);
|
|
WINRT_OBSERVABLE_PROPERTY(Editor::ColorTableEntry, CurrentCursorColor, _PropertyChangedHandlers, nullptr);
|
|
WINRT_OBSERVABLE_PROPERTY(Editor::ColorTableEntry, CurrentSelectionBackgroundColor, _PropertyChangedHandlers, nullptr);
|
|
|
|
private:
|
|
void _UpdateColorTable(const winrt::Microsoft::Terminal::Settings::Model::ColorScheme& colorScheme);
|
|
void _UpdateColorSchemeList();
|
|
void _RenameCurrentScheme(hstring newName);
|
|
};
|
|
|
|
struct ColorTableEntry : ColorTableEntryT<ColorTableEntry>
|
|
{
|
|
public:
|
|
ColorTableEntry(uint8_t index, Windows::UI::Color color);
|
|
ColorTableEntry(std::wstring_view tag, Windows::UI::Color color);
|
|
|
|
WINRT_CALLBACK(PropertyChanged, Windows::UI::Xaml::Data::PropertyChangedEventHandler);
|
|
WINRT_OBSERVABLE_PROPERTY(winrt::hstring, Name, _PropertyChangedHandlers);
|
|
WINRT_OBSERVABLE_PROPERTY(IInspectable, Tag, _PropertyChangedHandlers);
|
|
WINRT_OBSERVABLE_PROPERTY(Windows::UI::Color, Color, _PropertyChangedHandlers);
|
|
};
|
|
}
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Editor::factory_implementation
|
|
{
|
|
BASIC_FACTORY(ColorSchemes);
|
|
}
|