## Summary of the Pull Request 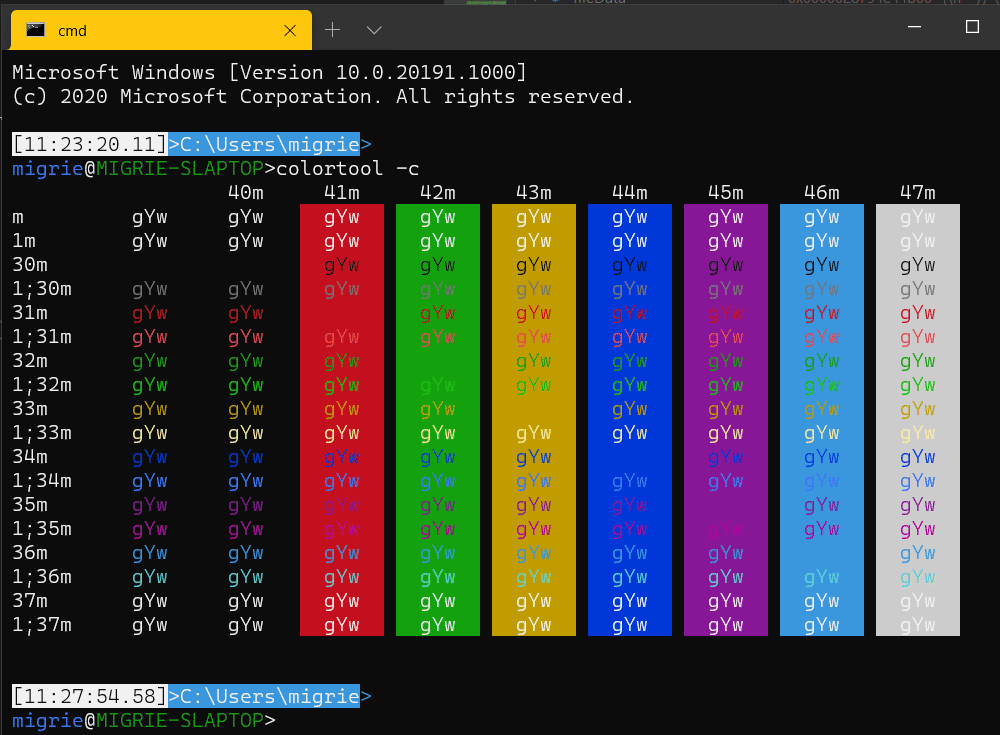 Allows for creating commands that iterate over the user's color schemes. Also adds a top-level nested command to `defaults.json` that allows the user to select a color scheme (pictured above). I'm not sure there are really any other use cases that make sense, but it _really_ makes sense for this one. ## References * #5400 - cmdpal megathread * made possible by #6856, _and support from viewers like you._ * All this is being done in pursuit of #6689 ## PR Checklist * [x] Closes wait what? I could have swore there was an issue for this one... * [x] I work here * [x] Tests added/passed * [ ] Requires documentation to be updated - okay maybe now I'll write some docs ## Detailed Description of the Pull Request / Additional comments Most of the hard work for this was already done in #6856. This is just another thing to iterate over. ## Validation Steps Performed * Played with this default command. It works great. * Added tests.
80 lines
3.3 KiB
C++
80 lines
3.3 KiB
C++
/*++
|
|
Copyright (c) Microsoft Corporation
|
|
Licensed under the MIT license.
|
|
|
|
Module Name:
|
|
- Command.h
|
|
|
|
Abstract:
|
|
- A command represents a single entry in the Command Palette. This is an object
|
|
that has a user facing "name" to display to the user, and an associated action
|
|
which can be dispatched.
|
|
|
|
- For more information, see GH#2046, #5400, #5674, and #6635
|
|
|
|
Author(s):
|
|
- Mike Griese - June 2020
|
|
|
|
--*/
|
|
#pragma once
|
|
|
|
#include "Command.g.h"
|
|
#include "TerminalWarnings.h"
|
|
#include "Profile.h"
|
|
#include "..\inc\cppwinrt_utils.h"
|
|
#include "SettingsTypes.h"
|
|
|
|
// fwdecl unittest classes
|
|
namespace TerminalAppLocalTests
|
|
{
|
|
class SettingsTests;
|
|
class CommandTests;
|
|
};
|
|
|
|
namespace winrt::TerminalApp::implementation
|
|
{
|
|
struct Command : CommandT<Command>
|
|
{
|
|
Command();
|
|
|
|
static winrt::com_ptr<Command> FromJson(const Json::Value& json,
|
|
std::vector<::TerminalApp::SettingsLoadWarnings>& warnings);
|
|
|
|
static void ExpandCommands(Windows::Foundation::Collections::IMap<winrt::hstring, winrt::TerminalApp::Command>& commands,
|
|
gsl::span<const ::TerminalApp::Profile> profiles,
|
|
gsl::span<winrt::TerminalApp::ColorScheme> schemes,
|
|
std::vector<::TerminalApp::SettingsLoadWarnings>& warnings);
|
|
|
|
static std::vector<::TerminalApp::SettingsLoadWarnings> LayerJson(Windows::Foundation::Collections::IMap<winrt::hstring, winrt::TerminalApp::Command>& commands,
|
|
const Json::Value& json);
|
|
bool HasNestedCommands();
|
|
Windows::Foundation::Collections::IMapView<winrt::hstring, TerminalApp::Command> NestedCommands();
|
|
|
|
winrt::Windows::UI::Xaml::Data::INotifyPropertyChanged::PropertyChanged_revoker propertyChangedRevoker;
|
|
|
|
WINRT_CALLBACK(PropertyChanged, Windows::UI::Xaml::Data::PropertyChangedEventHandler);
|
|
OBSERVABLE_GETSET_PROPERTY(winrt::hstring, Name, _PropertyChangedHandlers);
|
|
OBSERVABLE_GETSET_PROPERTY(winrt::TerminalApp::ActionAndArgs, Action, _PropertyChangedHandlers);
|
|
OBSERVABLE_GETSET_PROPERTY(winrt::hstring, KeyChordText, _PropertyChangedHandlers);
|
|
OBSERVABLE_GETSET_PROPERTY(winrt::Windows::UI::Xaml::Controls::IconSource, IconSource, _PropertyChangedHandlers, nullptr);
|
|
|
|
GETSET_PROPERTY(::TerminalApp::ExpandCommandType, IterateOn, ::TerminalApp::ExpandCommandType::None);
|
|
|
|
private:
|
|
Json::Value _originalJson;
|
|
Windows::Foundation::Collections::IMap<winrt::hstring, winrt::TerminalApp::Command> _subcommands{ nullptr };
|
|
|
|
static std::vector<winrt::TerminalApp::Command> _expandCommand(Command* const expandable,
|
|
gsl::span<const ::TerminalApp::Profile> profiles,
|
|
gsl::span<winrt::TerminalApp::ColorScheme> schemes,
|
|
std::vector<::TerminalApp::SettingsLoadWarnings>& warnings);
|
|
friend class TerminalAppLocalTests::SettingsTests;
|
|
friend class TerminalAppLocalTests::CommandTests;
|
|
};
|
|
}
|
|
|
|
namespace winrt::TerminalApp::factory_implementation
|
|
{
|
|
BASIC_FACTORY(Command);
|
|
}
|