## Summary of the Pull Request This PR lays the foundation for a new Actions page in the Settings UI as designed in #6900. The Actions page now leverages the `ActionMap` to display all of the key bindings and allow the user to modify the associated key chord or delete the key binding entirely. ## References #9621 - ActionMap #9926 - ActionMap serialization #9428 - ActionMap Spec #6900 - Actions page #9427 - Actions page design doc ## Detailed Description of the Pull Request / Additional comments ### Settings Model Changes - `Command::Copy()` now copies the `ActionAndArgs` - `ActionMap::RebindKeys()` handles changing the key chord of a key binding. If a conflict occurs, the conflicting key chord is overwritten. - `ActionMap::DeleteKeyBinding()` "deletes" a key binding by binding "unbound" to the given key chord. - `ActionMap::KeyBindings()` presents another view (similar to `NameMap`) of the `ActionMap`. It specifically presents a map of key chords to commands. It is generated similar to how `NameMap` is generated. ### Editor Changes - `Actions.xaml` is mainly split into two parts: - `ListView` (as before) holds the list of key bindings. We _could_ explore the idea of an items repeater, but the `ListView` seems to provide some niceties with regards to navigating the list via the key board (though none are selectable). - `DataTemplate` is used to represent each key binding inside the `ListView`. This is tricky because it is bound to a `KeyBindingViewModel` which must provide _all_ context necessary to modify the UI and the settings model. We cannot use names to target UI elements inside this template, so we must make the view model smart and force updates to the UI via changes in the view model. - `KeyBindingViewModel` is a view model object that controls the UI and the settings model. There are a number of TODOs in Actions.cpp will be long-term follow-ups and would be nice to have. This includes... - a binary search by name on `Actions::KeyBindingList` - presenting an error when the provided key chord is invalid. ## Demo 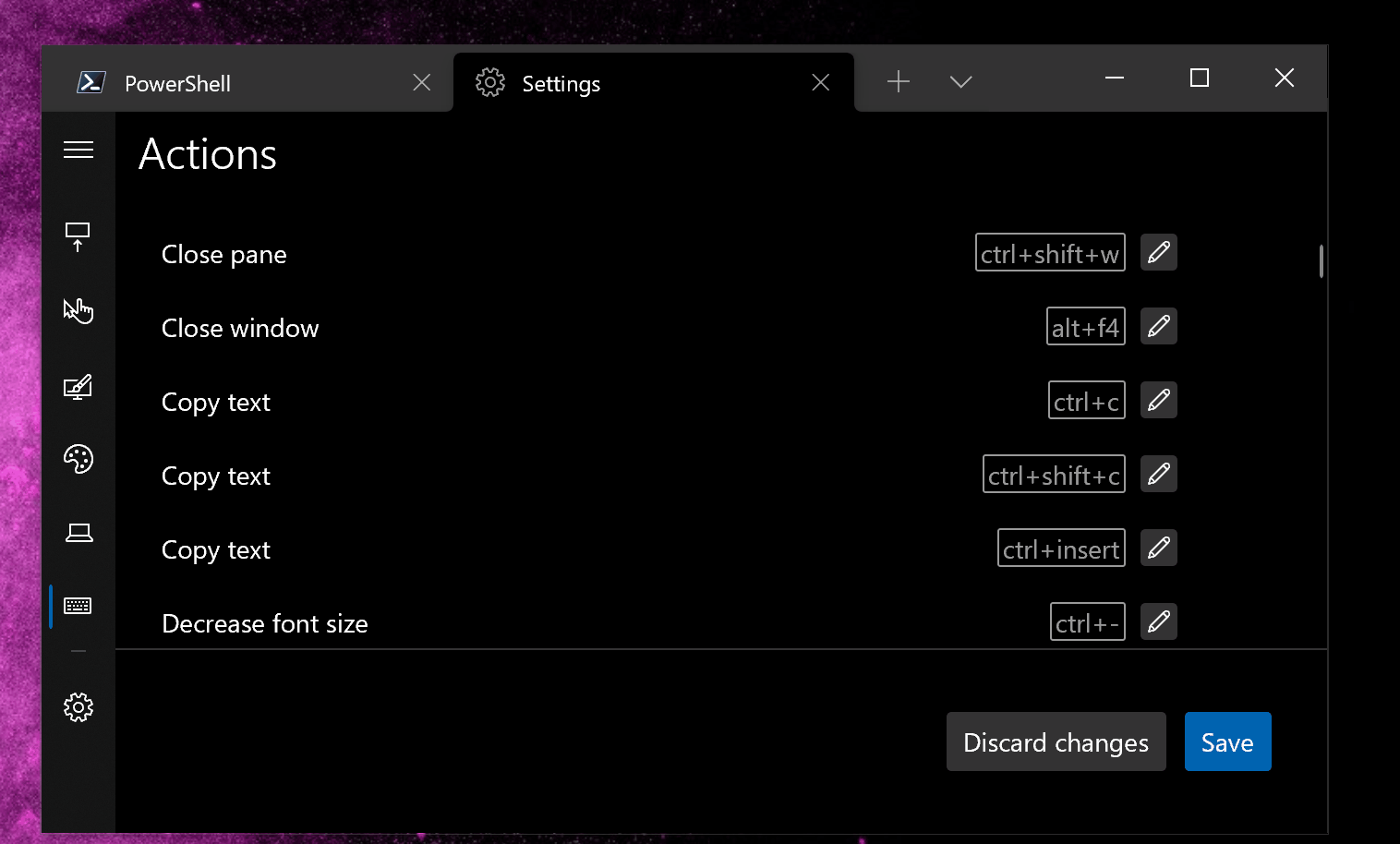
50 lines
1.4 KiB
C++
50 lines
1.4 KiB
C++
/*++
|
|
Copyright (c) Microsoft Corporation
|
|
Licensed under the MIT license.
|
|
|
|
Module Name:
|
|
- SettingContainer
|
|
|
|
Abstract:
|
|
- This is a XAML container that wraps settings in the Settings UI.
|
|
It interacts with the inheritance logic from the TerminalSettingsModel
|
|
and represents it in the Settings UI.
|
|
|
|
Author(s):
|
|
- Carlos Zamora - January 2021
|
|
|
|
--*/
|
|
|
|
#pragma once
|
|
|
|
#include "SettingContainer.g.h"
|
|
#include "Utils.h"
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Editor::implementation
|
|
{
|
|
struct SettingContainer : SettingContainerT<SettingContainer>
|
|
{
|
|
public:
|
|
SettingContainer();
|
|
|
|
void OnApplyTemplate();
|
|
|
|
DEPENDENCY_PROPERTY(Windows::Foundation::IInspectable, Header);
|
|
DEPENDENCY_PROPERTY(hstring, HelpText);
|
|
DEPENDENCY_PROPERTY(bool, HasSettingValue);
|
|
DEPENDENCY_PROPERTY(IInspectable, SettingOverrideSource);
|
|
TYPED_EVENT(ClearSettingValue, Editor::SettingContainer, Windows::Foundation::IInspectable);
|
|
|
|
private:
|
|
static void _InitializeProperties();
|
|
static void _OnHasSettingValueChanged(Windows::UI::Xaml::DependencyObject const& d, Windows::UI::Xaml::DependencyPropertyChangedEventArgs const& e);
|
|
static hstring _GenerateOverrideMessage(const Model::Profile& profile);
|
|
void _UpdateOverrideSystem();
|
|
};
|
|
}
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Editor::factory_implementation
|
|
{
|
|
BASIC_FACTORY(SettingContainer);
|
|
}
|