## Summary of the Pull Request This PR adds support for renaming windows. 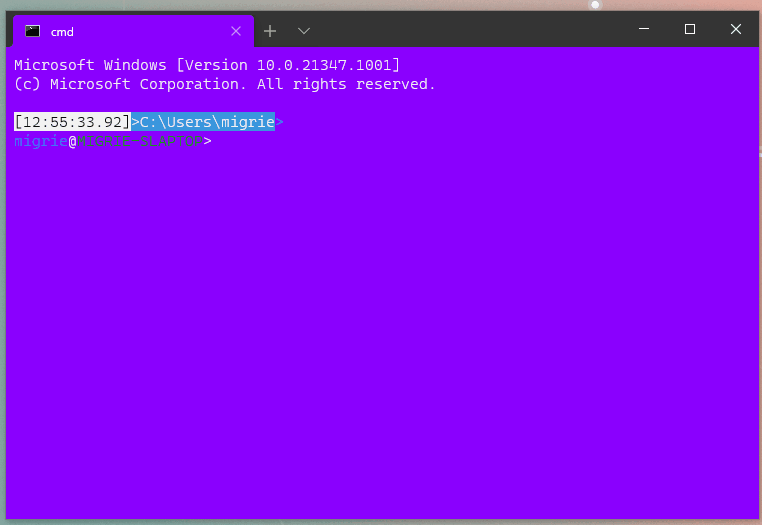 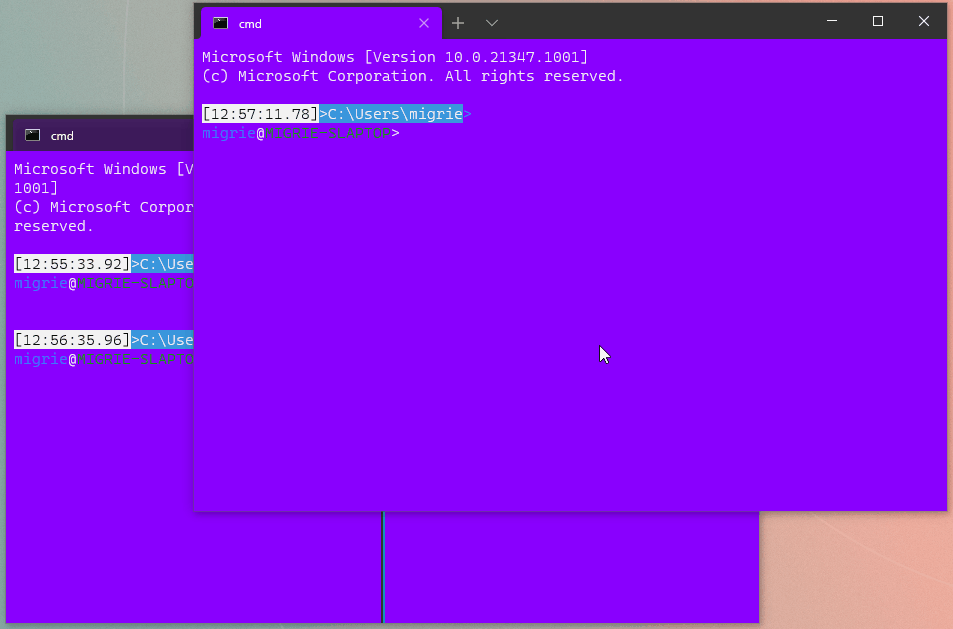 It does so through two new actions: * `renameWindow` takes a `name` parameter, and attempts to set the window's name to the provided name. This is useful if you always want to hit <kbd>F3</kbd> and rename a window to "foo" (READ: probably not that useful) * `openWindowRenamer` is more interesting: it opens a `TeachingTip` with a `TextBox`. When the user hits Ok, it'll request a rename for the provided value. This lets the user pick a new name for the window at runtime. In both cases, if there's already a window with that name, then the monarch will reject the rename, and pop a `Toast` in the window informing the user that the rename failed. Nifty! ## References * Builds on the toasts from #9523 * #5000 - process model megathread ## PR Checklist * [x] Closes https://github.com/microsoft/terminal/projects/5#card-50771747 * [x] I work here * [x] Tests addded (and pass with the help of #9660) * [ ] Requires documentation to be updated ## Detailed Description of the Pull Request / Additional comments I'm sending this PR while finishing up the tests. I figured I'll have time to sneak them in before I get the necessary reviews. > PAIN: We can't immediately focus the textbox in the TeachingTip. It's > not technically focusable until it is opened. However, it doesn't > provide an even tto tell us when it is opened. That's tracked in > microsoft/microsoft-ui-xaml#1607. So for now, the user _needs_ to > click on the text box manually. > We're also not using a ContentDialog for this, because in Xaml > Islands a text box in a ContentDialog won't recieve _any_ keypresses. > Fun! ## Validation Steps Performed I've been playing with ```json { "keys": "f1", "command": "identifyWindow" }, { "keys": "f2", "command": "identifyWindows" }, { "keys": "f3", "command": "openWindowRenamer" }, { "keys": "f4", "command": { "action": "renameWindow", "name": "foo" } }, { "keys": "f5", "command": { "action": "renameWindow", "name": "bar" } }, ``` and they seem to work as expected
255 lines
6.5 KiB
Plaintext
255 lines
6.5 KiB
Plaintext
// Copyright (c) Microsoft Corporation.
|
|
// Licensed under the MIT license.
|
|
|
|
namespace Microsoft.Terminal.Settings.Model
|
|
{
|
|
interface IActionArgs
|
|
{
|
|
Boolean Equals(IActionArgs other);
|
|
String GenerateName();
|
|
IActionArgs Copy();
|
|
};
|
|
|
|
interface IActionEventArgs
|
|
{
|
|
Boolean Handled;
|
|
IActionArgs ActionArgs { get; };
|
|
};
|
|
|
|
enum ResizeDirection
|
|
{
|
|
None = 0,
|
|
Left,
|
|
Right,
|
|
Up,
|
|
Down
|
|
};
|
|
|
|
enum FocusDirection
|
|
{
|
|
None = 0,
|
|
Left,
|
|
Right,
|
|
Up,
|
|
Down,
|
|
Previous
|
|
};
|
|
|
|
enum SplitState
|
|
{
|
|
Automatic = -1,
|
|
None = 0,
|
|
Vertical = 1,
|
|
Horizontal = 2
|
|
};
|
|
|
|
enum SplitType
|
|
{
|
|
Manual = 0,
|
|
Duplicate = 1
|
|
};
|
|
|
|
enum SettingsTarget
|
|
{
|
|
SettingsFile = 0,
|
|
DefaultsFile,
|
|
AllFiles,
|
|
SettingsUI
|
|
};
|
|
|
|
enum MoveTabDirection
|
|
{
|
|
None = 0,
|
|
Forward,
|
|
Backward
|
|
};
|
|
|
|
enum FindMatchDirection
|
|
{
|
|
None = 0,
|
|
Next,
|
|
Previous
|
|
};
|
|
|
|
enum CommandPaletteLaunchMode
|
|
{
|
|
Action = 0,
|
|
CommandLine
|
|
};
|
|
|
|
enum TabSwitcherMode
|
|
{
|
|
MostRecentlyUsed,
|
|
InOrder,
|
|
Disabled,
|
|
};
|
|
|
|
[default_interface] runtimeclass NewTerminalArgs {
|
|
NewTerminalArgs();
|
|
NewTerminalArgs(Int32 profileIndex);
|
|
NewTerminalArgs Copy();
|
|
|
|
String Commandline;
|
|
String StartingDirectory;
|
|
String TabTitle;
|
|
Windows.Foundation.IReference<Windows.UI.Color> TabColor;
|
|
String Profile; // Either a GUID or a profile's name if the GUID isn't a match
|
|
// ProfileIndex can be null (for "use the default"), so this needs to be
|
|
// a IReference, so it's nullable
|
|
Windows.Foundation.IReference<Int32> ProfileIndex { get; };
|
|
|
|
Windows.Foundation.IReference<Boolean> SuppressApplicationTitle;
|
|
|
|
String ColorScheme;
|
|
|
|
Boolean Equals(NewTerminalArgs other);
|
|
String GenerateName();
|
|
String ToCommandline();
|
|
};
|
|
|
|
[default_interface] runtimeclass ActionEventArgs : IActionEventArgs
|
|
{
|
|
ActionEventArgs();
|
|
ActionEventArgs(IActionArgs args);
|
|
};
|
|
|
|
[default_interface] runtimeclass CopyTextArgs : IActionArgs
|
|
{
|
|
Boolean SingleLine { get; };
|
|
Windows.Foundation.IReference<Microsoft.Terminal.Control.CopyFormat> CopyFormatting { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass NewTabArgs : IActionArgs
|
|
{
|
|
NewTabArgs(NewTerminalArgs terminalArgs);
|
|
NewTerminalArgs TerminalArgs { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass SwitchToTabArgs : IActionArgs
|
|
{
|
|
SwitchToTabArgs(UInt32 tabIndex);
|
|
UInt32 TabIndex;
|
|
};
|
|
|
|
[default_interface] runtimeclass ResizePaneArgs : IActionArgs
|
|
{
|
|
ResizeDirection ResizeDirection { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass MoveFocusArgs : IActionArgs
|
|
{
|
|
MoveFocusArgs(FocusDirection direction);
|
|
FocusDirection FocusDirection { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass AdjustFontSizeArgs : IActionArgs
|
|
{
|
|
Int32 Delta { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass SendInputArgs : IActionArgs
|
|
{
|
|
String Input { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass SplitPaneArgs : IActionArgs
|
|
{
|
|
SplitPaneArgs(SplitType splitMode, SplitState split, Double size, NewTerminalArgs terminalArgs);
|
|
SplitPaneArgs(SplitState split, Double size, NewTerminalArgs terminalArgs);
|
|
SplitPaneArgs(SplitState split, NewTerminalArgs terminalArgs);
|
|
SplitPaneArgs(SplitType splitMode);
|
|
|
|
SplitState SplitStyle { get; };
|
|
NewTerminalArgs TerminalArgs { get; };
|
|
SplitType SplitMode { get; };
|
|
Double SplitSize { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass OpenSettingsArgs : IActionArgs
|
|
{
|
|
OpenSettingsArgs(SettingsTarget target);
|
|
SettingsTarget Target { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass SetColorSchemeArgs : IActionArgs
|
|
{
|
|
String SchemeName { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass SetTabColorArgs : IActionArgs
|
|
{
|
|
Windows.Foundation.IReference<Windows.UI.Color> TabColor { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass RenameTabArgs : IActionArgs
|
|
{
|
|
String Title { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass ExecuteCommandlineArgs : IActionArgs
|
|
{
|
|
ExecuteCommandlineArgs(String commandline);
|
|
String Commandline;
|
|
};
|
|
|
|
[default_interface] runtimeclass CloseOtherTabsArgs : IActionArgs
|
|
{
|
|
CloseOtherTabsArgs(UInt32 tabIndex);
|
|
Windows.Foundation.IReference<UInt32> Index { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass CloseTabsAfterArgs : IActionArgs
|
|
{
|
|
CloseTabsAfterArgs(UInt32 tabIndex);
|
|
Windows.Foundation.IReference<UInt32> Index { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass MoveTabArgs : IActionArgs
|
|
{
|
|
MoveTabArgs(MoveTabDirection direction);
|
|
MoveTabDirection Direction { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass ScrollUpArgs : IActionArgs
|
|
{
|
|
Windows.Foundation.IReference<UInt32> RowsToScroll { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass ScrollDownArgs : IActionArgs
|
|
{
|
|
Windows.Foundation.IReference<UInt32> RowsToScroll { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass ToggleCommandPaletteArgs : IActionArgs
|
|
{
|
|
CommandPaletteLaunchMode LaunchMode { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass FindMatchArgs : IActionArgs
|
|
{
|
|
FindMatchArgs(FindMatchDirection direction);
|
|
FindMatchDirection Direction { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass NewWindowArgs : IActionArgs
|
|
{
|
|
NewWindowArgs(NewTerminalArgs terminalArgs);
|
|
NewTerminalArgs TerminalArgs { get; };
|
|
};
|
|
|
|
[default_interface] runtimeclass PrevTabArgs : IActionArgs
|
|
{
|
|
Windows.Foundation.IReference<TabSwitcherMode> SwitcherMode;
|
|
};
|
|
|
|
[default_interface] runtimeclass NextTabArgs : IActionArgs
|
|
{
|
|
Windows.Foundation.IReference<TabSwitcherMode> SwitcherMode;
|
|
};
|
|
|
|
[default_interface] runtimeclass RenameWindowArgs : IActionArgs
|
|
{
|
|
String Name { get; };
|
|
};
|
|
}
|