2019-05-03 00:29:04 +02:00
|
|
|
// Copyright (c) Microsoft Corporation.
|
|
|
|
// Licensed under the MIT license.
|
|
|
|
|
|
|
|
#include "pch.h"
|
2020-05-04 22:57:12 +02:00
|
|
|
#include <LibraryResources.h>
|
|
|
|
#include "ColorPickupFlyout.h"
|
2019-05-03 00:29:04 +02:00
|
|
|
#include "Tab.h"
|
2020-02-04 22:51:11 +01:00
|
|
|
#include "Tab.g.cpp"
|
2019-08-15 01:12:14 +02:00
|
|
|
#include "Utils.h"
|
2020-05-04 22:57:12 +02:00
|
|
|
#include "ColorHelper.h"
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
using namespace winrt;
|
2019-05-03 00:29:04 +02:00
|
|
|
using namespace winrt::Windows::UI::Xaml;
|
|
|
|
using namespace winrt::Windows::UI::Core;
|
2019-06-07 23:56:44 +02:00
|
|
|
using namespace winrt::Microsoft::Terminal::TerminalControl;
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
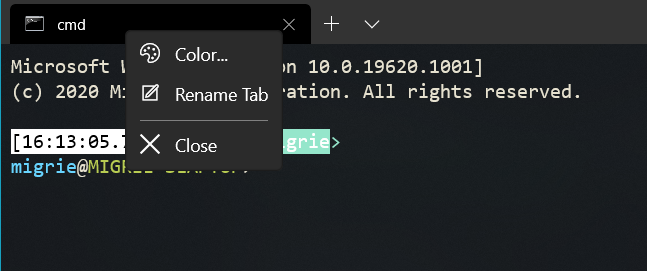
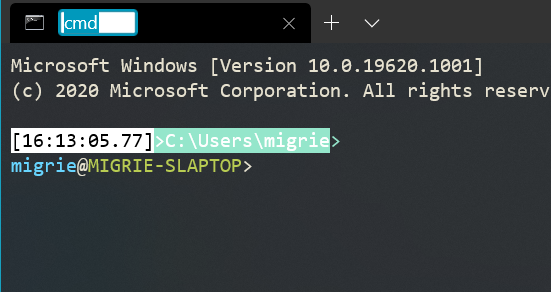
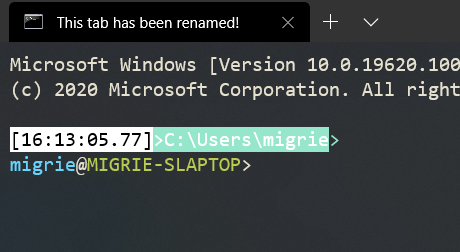
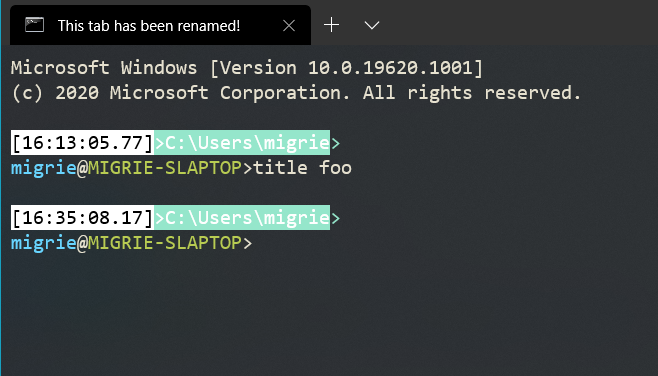
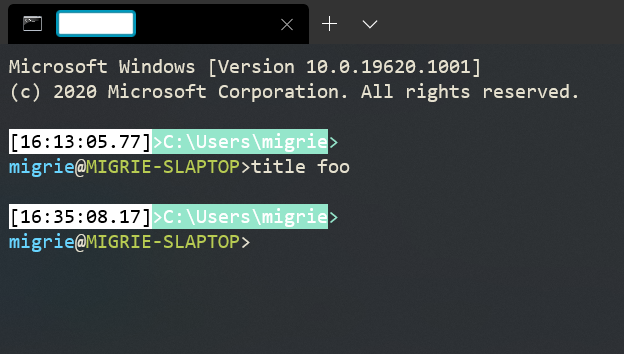
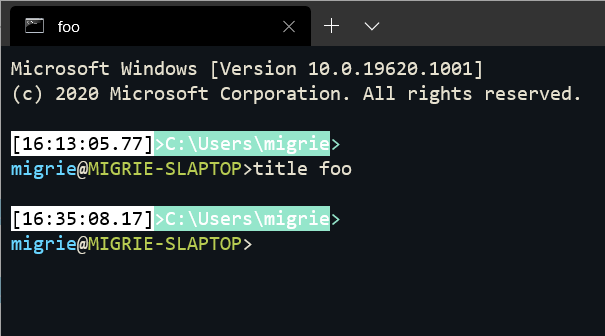
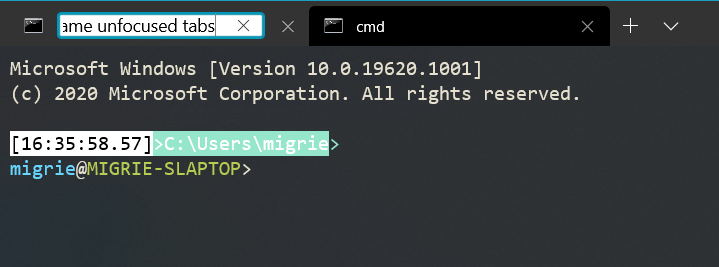
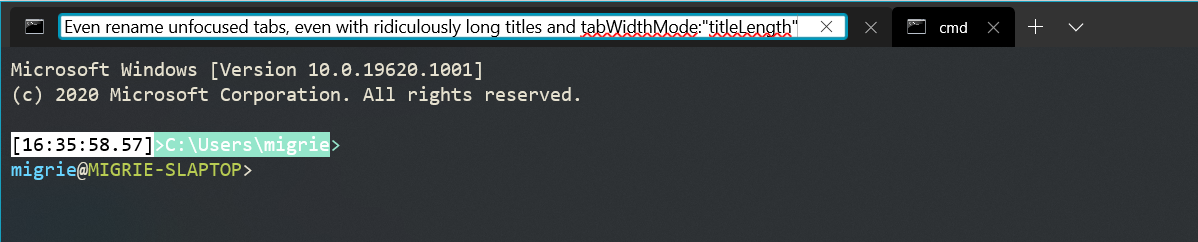
2020-05-28 23:06:17 +02:00
|
|
|
using namespace winrt::Windows::System;
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2019-10-15 07:41:43 +02:00
|
|
|
namespace winrt
|
|
|
|
{
|
|
|
|
namespace MUX = Microsoft::UI::Xaml;
|
|
|
|
}
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
namespace winrt::TerminalApp::implementation
|
2019-05-03 00:29:04 +02:00
|
|
|
{
|
2020-02-04 22:51:11 +01:00
|
|
|
Tab::Tab(const GUID& profile, const TermControl& control)
|
|
|
|
{
|
|
|
|
_rootPane = std::make_shared<Pane>(profile, control, true);
|
Decouple "Active Terminal" and "Focused Control" (#3540)
## Summary of the Pull Request
Unties the concept of "focused control" from "active control".
Previously, we were exclusively using the "Focused" state of `TermControl`s to determine which one was active. This was fraught with gotchas - if anything else became focused, then suddenly there was _no_ pane focused in the Tab. This happened especially frequently if the user clicked on a tab to focus the window. Furthermore, in experimental branches with more UI added to the Terminal (such as [dev/migrie/f/2046-command-palette](https://github.com/microsoft/terminal/tree/dev/migrie/f/2046-command-palette)), when these UIs were added to the Terminal, they'd take focus, which again meant that there was no focused pane.
This fixes these issue by having each Tab manually track which Pane is active in that tab. The Tab is now the arbiter of who in the tree is "active". Panes still track this state, for them to be able to MoveFocus appropriately.
It also contains a related fix to prevent the tab separator from stealing focus from the TermControl. This required us to set the color of the un-focused Pane border to some color other that Transparent, so I went with the TabViewBackground. Panes now look like the following:
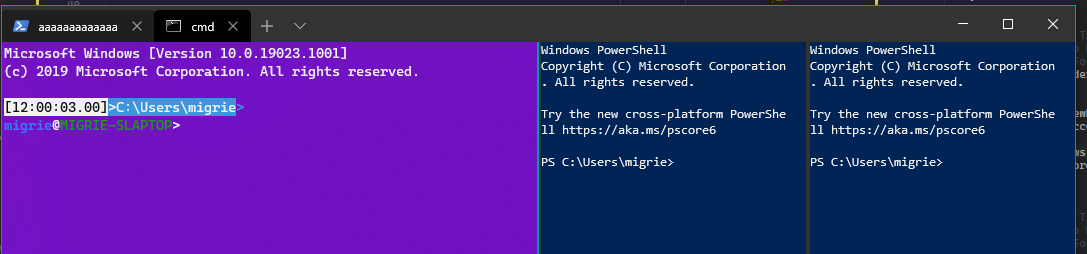
## References
See also: #2046
## PR Checklist
* [x] Closes #1205
* [x] Closes #522
* [x] Closes #999
* [x] I work here
* [😢] Tests added/passed
* [n/a] Requires documentation to be updated
## Validation Steps Performed
Tested manually opening panes, closing panes, clicking around panes, the whole dance.
---------------------------------------------------
* this is janky but is close for some reason?
* This is _almost_ right to solve #1205
If I want to double up and also fix #522 (which I do), then I need to also
* when a tab GetsFocus, send the focus instead to the Pane
* When the border is clicked on, focus that pane's control
And like a lot of cleanup, because this is horrifying
* hey this autorevoker is really nice
* Encapsulate Pane::pfnGotFocus
* Propogate the events back up on close
* Encapsulate Tab::pfnFocusChanged, and clean up TerminalPage a bit
* Mostly just code cleanup, commenting
* This works to hittest on the borders
If the border is `Transparent`, then it can't hittest for Tapped events, and it'll fall through (to someone)
THis at least works, but looks garish
* Match the pane border to the TabViewHeader
* Fix a bit of dead code and a bad copy-pasta
* This _works_ to use a winrt event, but it's dirty
* Clean up everything from the winrt::event debacle.
* This is dead code that shouldn't have been there
* Turn Tab's callback into a winrt::event as well
2019-11-18 22:41:25 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
_rootPane->Closed([=](auto&& /*s*/, auto&& /*e*/) {
|
|
|
|
_ClosedHandlers(nullptr, nullptr);
|
|
|
|
});
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
_activePane = _rootPane;
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
_MakeTabViewItem();
|
|
|
|
}
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Initializes a TabViewItem for this Tab instance.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_MakeTabViewItem()
|
|
|
|
{
|
|
|
|
_tabViewItem = ::winrt::MUX::Controls::TabViewItem{};
|
2020-06-22 18:17:25 +02:00
|
|
|
|
|
|
|
_tabViewItem.DoubleTapped([weakThis = get_weak()](auto&& /*s*/, auto&& /*e*/) {
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
|
|
|
tab->_inRename = true;
|
|
|
|
tab->_UpdateTabHeader();
|
|
|
|
}
|
|
|
|
});
|
|
|
|
|
2020-06-09 23:47:13 +02:00
|
|
|
_UpdateTitle();
|
2020-02-04 22:51:11 +01:00
|
|
|
}
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Get the root UIElement of this Tab's root pane.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - The UIElement acting as root of the Tab's root pane.
|
|
|
|
UIElement Tab::GetRootElement()
|
|
|
|
{
|
|
|
|
return _rootPane->GetRootElement();
|
|
|
|
}
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Returns nullptr if no children of this tab were the last control to be
|
|
|
|
// focused, or the TermControl that _was_ the last control to be focused (if
|
|
|
|
// there was one).
|
|
|
|
// - This control might not currently be focused, if the tab itself is not
|
|
|
|
// currently focused.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - nullptr if no children were marked `_lastFocused`, else the TermControl
|
|
|
|
// that was last focused.
|
|
|
|
TermControl Tab::GetActiveTerminalControl() const
|
2019-05-03 00:29:04 +02:00
|
|
|
{
|
2020-02-04 22:51:11 +01:00
|
|
|
return _activePane->GetTerminalControl();
|
2019-05-03 00:29:04 +02:00
|
|
|
}
|
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Gets the TabViewItem that represents this Tab
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - The TabViewItem that represents this Tab
|
|
|
|
winrt::MUX::Controls::TabViewItem Tab::GetTabViewItem()
|
|
|
|
{
|
|
|
|
return _tabViewItem;
|
|
|
|
}
|
2019-06-07 23:56:44 +02:00
|
|
|
|
2020-05-04 22:57:12 +02:00
|
|
|
// Method Description:
|
|
|
|
// - Called after construction of a Tab object to bind event handlers to its
|
|
|
|
// associated Pane and TermControl object and to create the context menu of
|
|
|
|
// the tab item
|
|
|
|
// Arguments:
|
|
|
|
// - control: reference to the TermControl object to bind event to
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::Initialize(const TermControl& control)
|
|
|
|
{
|
|
|
|
_BindEventHandlers(control);
|
|
|
|
_CreateContextMenu();
|
|
|
|
}
|
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Returns true if this is the currently focused tab. For any set of tabs,
|
|
|
|
// there should only be one tab that is marked as focused, though each tab has
|
|
|
|
// no control over the other tabs in the set.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - true iff this tab is focused.
|
|
|
|
bool Tab::IsFocused() const noexcept
|
|
|
|
{
|
|
|
|
return _focused;
|
|
|
|
}
|
Fixed self reference capture in Tab and TerminalPage (#3835)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
Every lambda capture in `Tab` and `TerminalPage` has been changed from capturing raw `this` to `std::weak_ptr<Tab>` or `winrt::weak_ref<TerminalPage>`. Lambda bodies have been changed to check the weak reference before use.
Capturing raw `this` in `Tab`'s [title change event handler](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/Tab.cpp#L299) was the root cause of #3776, and is fixed in this PR among other instance of raw `this` capture.
The lambda fixes to `TerminalPage` are unrelated to the core issue addressed in the PR checklist. Because I was already editing `TerminalPage`, figured I'd do a [weak_ref pass](https://github.com/microsoft/terminal/issues/3776#issuecomment-560575575).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3776, potentially #2248, likely closes others
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3776
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
`Tab` now inherits from `enable_shared_from_this`, which enable accessing `Tab` objects as `std::weak_ptr<Tab>` objects. All instances of lambdas capturing `this` now capture `std::weak_ptr<Tab>` instead. `TerminalPage` is a WinRT type which supports `winrt::weak_ref<TerminalPage>`. All previous instance of `TerminalPage` lambdas capturing `this` has been replaced to capture `winrt::weak_ref<TerminalPage>`. These weak pointers/references can only be created after object construction necessitating for `Tab` a new function called after construction to bind lambdas.
Any anomalous crash related to the following functionality during closing a tab or WT may be fixed by this PR:
- Tab icon updating
- Tab text updating
- Tab dragging
- Clicking new tab button
- Changing active pane
- Closing an active tab
- Clicking on a tab
- Creating the new tab flyout menu
Sorry about all the commits. Will fix my fork after this PR! 😅
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Attempted to repro the steps indicated in issue #3776 with the new changes and failed. When before the changes, the issue could consistently be reproed.
2019-12-06 00:18:22 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Updates our focus state. If we're gaining focus, make sure to transfer
|
|
|
|
// focus to the last focused terminal control in our tree of controls.
|
|
|
|
// Arguments:
|
|
|
|
// - focused: our new focus state. If true, we should be focused. If false, we
|
|
|
|
// should be unfocused.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::SetFocused(const bool focused)
|
|
|
|
{
|
|
|
|
_focused = focused;
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
if (_focused)
|
|
|
|
{
|
|
|
|
_Focus();
|
|
|
|
}
|
|
|
|
}
|
2019-06-07 23:56:44 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Returns nullopt if no children of this tab were the last control to be
|
|
|
|
// focused, or the GUID of the profile of the last control to be focused (if
|
|
|
|
// there was one).
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - nullopt if no children of this tab were the last control to be
|
|
|
|
// focused, else the GUID of the profile of the last control to be focused
|
|
|
|
std::optional<GUID> Tab::GetFocusedProfile() const noexcept
|
2019-06-07 23:56:44 +02:00
|
|
|
{
|
2020-02-04 22:51:11 +01:00
|
|
|
return _activePane->GetFocusedProfile();
|
2019-06-07 23:56:44 +02:00
|
|
|
}
|
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Called after construction of a Tab object to bind event handlers to its
|
|
|
|
// associated Pane and TermControl object
|
|
|
|
// Arguments:
|
|
|
|
// - control: reference to the TermControl object to bind event to
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
2020-05-04 22:57:12 +02:00
|
|
|
void Tab::_BindEventHandlers(const TermControl& control) noexcept
|
2019-08-15 01:12:14 +02:00
|
|
|
{
|
2020-02-04 22:51:11 +01:00
|
|
|
_AttachEventHandlersToPane(_rootPane);
|
|
|
|
_AttachEventHandlersToControl(control);
|
2019-08-15 01:12:14 +02:00
|
|
|
}
|
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Attempts to update the settings of this tab's tree of panes.
|
|
|
|
// Arguments:
|
|
|
|
// - settings: The new TerminalSettings to apply to any matching controls
|
|
|
|
// - profile: The GUID of the profile these settings should apply to.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::UpdateSettings(const TerminalSettings& settings, const GUID& profile)
|
|
|
|
{
|
|
|
|
_rootPane->UpdateSettings(settings, profile);
|
|
|
|
}
|
2019-08-15 01:12:14 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Focus the last focused control in our tree of panes.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_Focus()
|
|
|
|
{
|
|
|
|
_focused = true;
|
Fixed self reference capture in Tab and TerminalPage (#3835)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
Every lambda capture in `Tab` and `TerminalPage` has been changed from capturing raw `this` to `std::weak_ptr<Tab>` or `winrt::weak_ref<TerminalPage>`. Lambda bodies have been changed to check the weak reference before use.
Capturing raw `this` in `Tab`'s [title change event handler](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/Tab.cpp#L299) was the root cause of #3776, and is fixed in this PR among other instance of raw `this` capture.
The lambda fixes to `TerminalPage` are unrelated to the core issue addressed in the PR checklist. Because I was already editing `TerminalPage`, figured I'd do a [weak_ref pass](https://github.com/microsoft/terminal/issues/3776#issuecomment-560575575).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3776, potentially #2248, likely closes others
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3776
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
`Tab` now inherits from `enable_shared_from_this`, which enable accessing `Tab` objects as `std::weak_ptr<Tab>` objects. All instances of lambdas capturing `this` now capture `std::weak_ptr<Tab>` instead. `TerminalPage` is a WinRT type which supports `winrt::weak_ref<TerminalPage>`. All previous instance of `TerminalPage` lambdas capturing `this` has been replaced to capture `winrt::weak_ref<TerminalPage>`. These weak pointers/references can only be created after object construction necessitating for `Tab` a new function called after construction to bind lambdas.
Any anomalous crash related to the following functionality during closing a tab or WT may be fixed by this PR:
- Tab icon updating
- Tab text updating
- Tab dragging
- Clicking new tab button
- Changing active pane
- Closing an active tab
- Clicking on a tab
- Creating the new tab flyout menu
Sorry about all the commits. Will fix my fork after this PR! 😅
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Attempted to repro the steps indicated in issue #3776 with the new changes and failed. When before the changes, the issue could consistently be reproed.
2019-12-06 00:18:22 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
auto lastFocusedControl = GetActiveTerminalControl();
|
|
|
|
if (lastFocusedControl)
|
|
|
|
{
|
|
|
|
lastFocusedControl.Focus(FocusState::Programmatic);
|
|
|
|
}
|
|
|
|
}
|
Converts Dispatcher().RunAsync to WinRT Coroutines (#4051)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
This PR turns all* instances of `Dispatcher().RunAsync` to WinRT coroutines 👌.
This was good coding fodder to fill my plane ride ✈️. Enjoy your holidays everyone!
*With the exception of three functions whose signatures cannot be changed due to inheritance and function overriding in `TermControlAutomationPeer` [`L44`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L44), [`L58`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L58), [`L72`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L72).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3919
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3919
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
My thought pattern here was to minimally disturb the existing code where possible. So where I could, I converted existing functions into coroutine using functions (like in the [core example](https://github.com/microsoft/terminal/issues/3919#issue-536598706)). For ~the most part~ all instances, I used the format where [`this` is accessed safely within a locked scope](https://github.com/microsoft/terminal/issues/3919#issuecomment-564730620). Some function signatures were changed to take objects by value instead of reference, so the coroutines don't crash when the objects are accessed past their original lifetime. The [copy](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1132) and [paste](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1170) event handler entry points were originally set to a high priority; however, the WinRT coroutines don't appear to support a priority scheme so this priority setting was not preserved in the translation.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Compiles and runs, and for every event with a clear trigger repro, I triggered it to ensure crashes weren't introduced.
2020-01-10 04:29:49 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Set the icon on the TabViewItem for this tab.
|
|
|
|
// Arguments:
|
|
|
|
// - iconPath: The new path string to use as the IconPath for our TabViewItem
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
winrt::fire_and_forget Tab::UpdateIcon(const winrt::hstring iconPath)
|
Converts Dispatcher().RunAsync to WinRT Coroutines (#4051)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
This PR turns all* instances of `Dispatcher().RunAsync` to WinRT coroutines 👌.
This was good coding fodder to fill my plane ride ✈️. Enjoy your holidays everyone!
*With the exception of three functions whose signatures cannot be changed due to inheritance and function overriding in `TermControlAutomationPeer` [`L44`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L44), [`L58`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L58), [`L72`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L72).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3919
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3919
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
My thought pattern here was to minimally disturb the existing code where possible. So where I could, I converted existing functions into coroutine using functions (like in the [core example](https://github.com/microsoft/terminal/issues/3919#issue-536598706)). For ~the most part~ all instances, I used the format where [`this` is accessed safely within a locked scope](https://github.com/microsoft/terminal/issues/3919#issuecomment-564730620). Some function signatures were changed to take objects by value instead of reference, so the coroutines don't crash when the objects are accessed past their original lifetime. The [copy](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1132) and [paste](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1170) event handler entry points were originally set to a high priority; however, the WinRT coroutines don't appear to support a priority scheme so this priority setting was not preserved in the translation.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Compiles and runs, and for every event with a clear trigger repro, I triggered it to ensure crashes weren't introduced.
2020-01-10 04:29:49 +01:00
|
|
|
{
|
2020-02-04 22:51:11 +01:00
|
|
|
// Don't reload our icon if it hasn't changed.
|
|
|
|
if (iconPath == _lastIconPath)
|
|
|
|
{
|
|
|
|
return;
|
|
|
|
}
|
2019-08-15 01:12:14 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
_lastIconPath = iconPath;
|
2019-06-07 23:56:44 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
auto weakThis{ get_weak() };
|
Fixed self reference capture in Tab and TerminalPage (#3835)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
Every lambda capture in `Tab` and `TerminalPage` has been changed from capturing raw `this` to `std::weak_ptr<Tab>` or `winrt::weak_ref<TerminalPage>`. Lambda bodies have been changed to check the weak reference before use.
Capturing raw `this` in `Tab`'s [title change event handler](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/Tab.cpp#L299) was the root cause of #3776, and is fixed in this PR among other instance of raw `this` capture.
The lambda fixes to `TerminalPage` are unrelated to the core issue addressed in the PR checklist. Because I was already editing `TerminalPage`, figured I'd do a [weak_ref pass](https://github.com/microsoft/terminal/issues/3776#issuecomment-560575575).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3776, potentially #2248, likely closes others
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3776
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
`Tab` now inherits from `enable_shared_from_this`, which enable accessing `Tab` objects as `std::weak_ptr<Tab>` objects. All instances of lambdas capturing `this` now capture `std::weak_ptr<Tab>` instead. `TerminalPage` is a WinRT type which supports `winrt::weak_ref<TerminalPage>`. All previous instance of `TerminalPage` lambdas capturing `this` has been replaced to capture `winrt::weak_ref<TerminalPage>`. These weak pointers/references can only be created after object construction necessitating for `Tab` a new function called after construction to bind lambdas.
Any anomalous crash related to the following functionality during closing a tab or WT may be fixed by this PR:
- Tab icon updating
- Tab text updating
- Tab dragging
- Clicking new tab button
- Changing active pane
- Closing an active tab
- Clicking on a tab
- Creating the new tab flyout menu
Sorry about all the commits. Will fix my fork after this PR! 😅
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Attempted to repro the steps indicated in issue #3776 with the new changes and failed. When before the changes, the issue could consistently be reproed.
2019-12-06 00:18:22 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
co_await winrt::resume_foreground(_tabViewItem.Dispatcher());
|
Converts Dispatcher().RunAsync to WinRT Coroutines (#4051)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
This PR turns all* instances of `Dispatcher().RunAsync` to WinRT coroutines 👌.
This was good coding fodder to fill my plane ride ✈️. Enjoy your holidays everyone!
*With the exception of three functions whose signatures cannot be changed due to inheritance and function overriding in `TermControlAutomationPeer` [`L44`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L44), [`L58`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L58), [`L72`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L72).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3919
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3919
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
My thought pattern here was to minimally disturb the existing code where possible. So where I could, I converted existing functions into coroutine using functions (like in the [core example](https://github.com/microsoft/terminal/issues/3919#issue-536598706)). For ~the most part~ all instances, I used the format where [`this` is accessed safely within a locked scope](https://github.com/microsoft/terminal/issues/3919#issuecomment-564730620). Some function signatures were changed to take objects by value instead of reference, so the coroutines don't crash when the objects are accessed past their original lifetime. The [copy](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1132) and [paste](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1170) event handler entry points were originally set to a high priority; however, the WinRT coroutines don't appear to support a priority scheme so this priority setting was not preserved in the translation.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Compiles and runs, and for every event with a clear trigger repro, I triggered it to ensure crashes weren't introduced.
2020-01-10 04:29:49 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
|
|
|
IconPath(_lastIconPath);
|
|
|
|
_tabViewItem.IconSource(GetColoredIcon<winrt::MUX::Controls::IconSource>(_lastIconPath));
|
|
|
|
}
|
Converts Dispatcher().RunAsync to WinRT Coroutines (#4051)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
This PR turns all* instances of `Dispatcher().RunAsync` to WinRT coroutines 👌.
This was good coding fodder to fill my plane ride ✈️. Enjoy your holidays everyone!
*With the exception of three functions whose signatures cannot be changed due to inheritance and function overriding in `TermControlAutomationPeer` [`L44`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L44), [`L58`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L58), [`L72`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L72).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3919
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3919
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
My thought pattern here was to minimally disturb the existing code where possible. So where I could, I converted existing functions into coroutine using functions (like in the [core example](https://github.com/microsoft/terminal/issues/3919#issue-536598706)). For ~the most part~ all instances, I used the format where [`this` is accessed safely within a locked scope](https://github.com/microsoft/terminal/issues/3919#issuecomment-564730620). Some function signatures were changed to take objects by value instead of reference, so the coroutines don't crash when the objects are accessed past their original lifetime. The [copy](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1132) and [paste](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1170) event handler entry points were originally set to a high priority; however, the WinRT coroutines don't appear to support a priority scheme so this priority setting was not preserved in the translation.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Compiles and runs, and for every event with a clear trigger repro, I triggered it to ensure crashes weren't introduced.
2020-01-10 04:29:49 +01:00
|
|
|
}
|
2019-05-03 00:29:04 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Gets the title string of the last focused terminal control in our tree.
|
|
|
|
// Returns the empty string if there is no such control.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - the title string of the last focused terminal control in our tree.
|
|
|
|
winrt::hstring Tab::GetActiveTitle() const
|
|
|
|
{
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
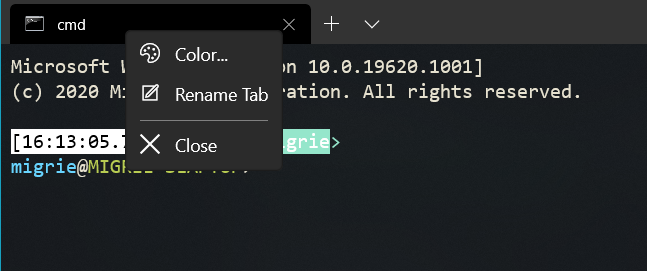
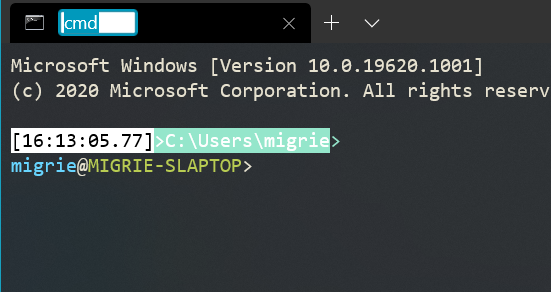
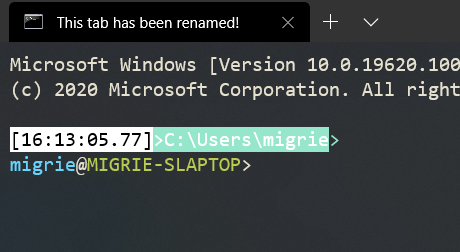
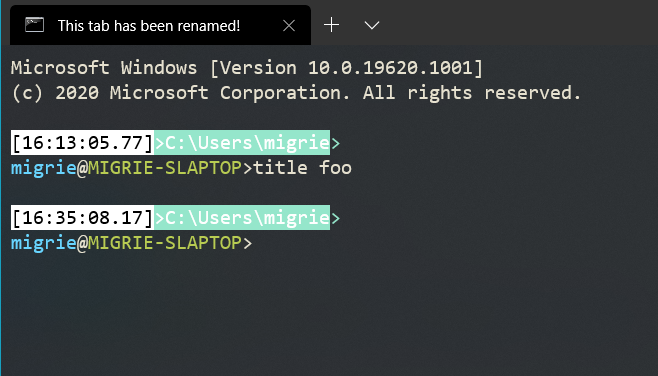
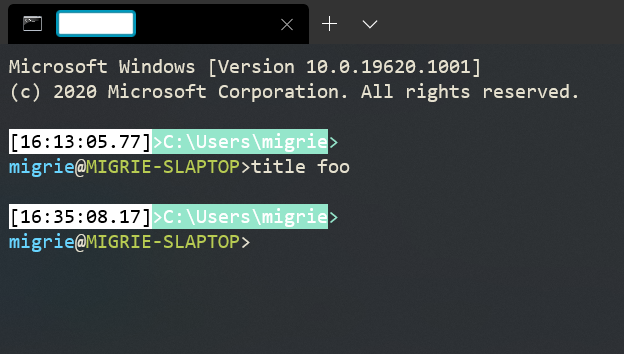
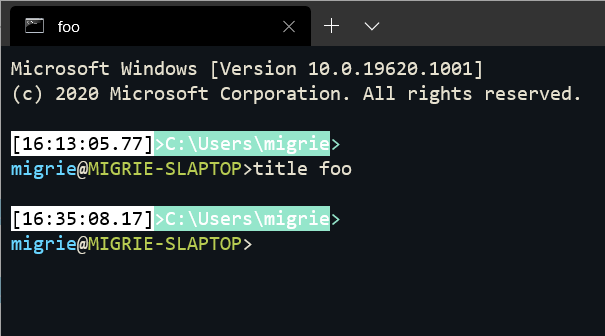
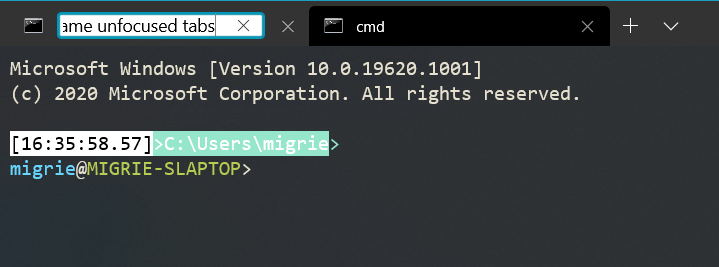
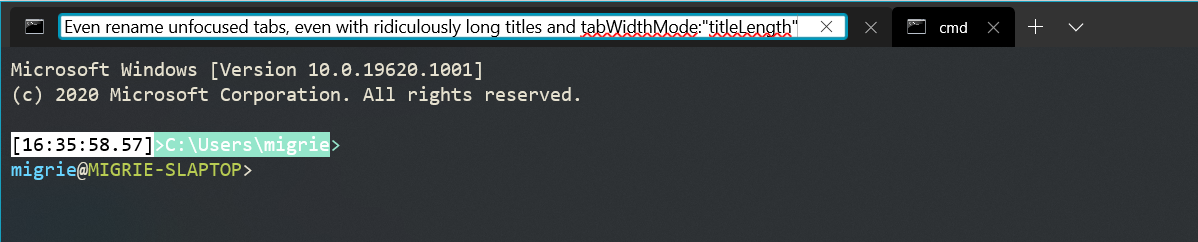
2020-05-28 23:06:17 +02:00
|
|
|
if (!_runtimeTabText.empty())
|
|
|
|
{
|
|
|
|
return _runtimeTabText;
|
|
|
|
}
|
2020-02-04 22:51:11 +01:00
|
|
|
const auto lastFocusedControl = GetActiveTerminalControl();
|
|
|
|
return lastFocusedControl ? lastFocusedControl.Title() : L"";
|
|
|
|
}
|
Converts Dispatcher().RunAsync to WinRT Coroutines (#4051)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
This PR turns all* instances of `Dispatcher().RunAsync` to WinRT coroutines 👌.
This was good coding fodder to fill my plane ride ✈️. Enjoy your holidays everyone!
*With the exception of three functions whose signatures cannot be changed due to inheritance and function overriding in `TermControlAutomationPeer` [`L44`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L44), [`L58`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L58), [`L72`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L72).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3919
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3919
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
My thought pattern here was to minimally disturb the existing code where possible. So where I could, I converted existing functions into coroutine using functions (like in the [core example](https://github.com/microsoft/terminal/issues/3919#issue-536598706)). For ~the most part~ all instances, I used the format where [`this` is accessed safely within a locked scope](https://github.com/microsoft/terminal/issues/3919#issuecomment-564730620). Some function signatures were changed to take objects by value instead of reference, so the coroutines don't crash when the objects are accessed past their original lifetime. The [copy](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1132) and [paste](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1170) event handler entry points were originally set to a high priority; however, the WinRT coroutines don't appear to support a priority scheme so this priority setting was not preserved in the translation.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Compiles and runs, and for every event with a clear trigger repro, I triggered it to ensure crashes weren't introduced.
2020-01-10 04:29:49 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
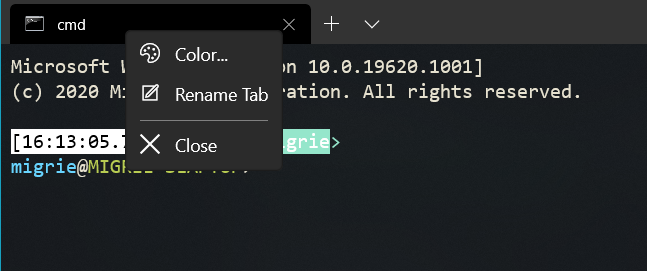
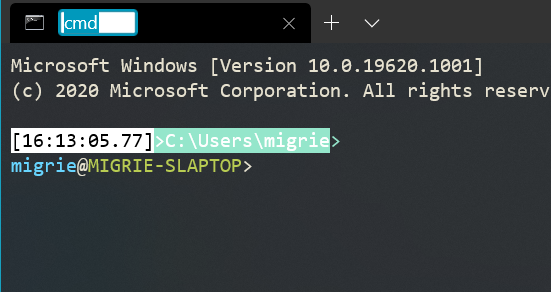
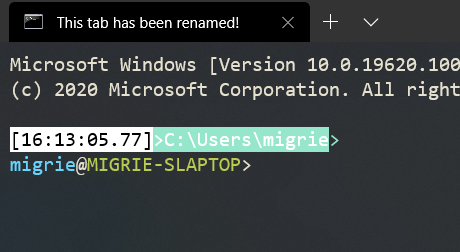
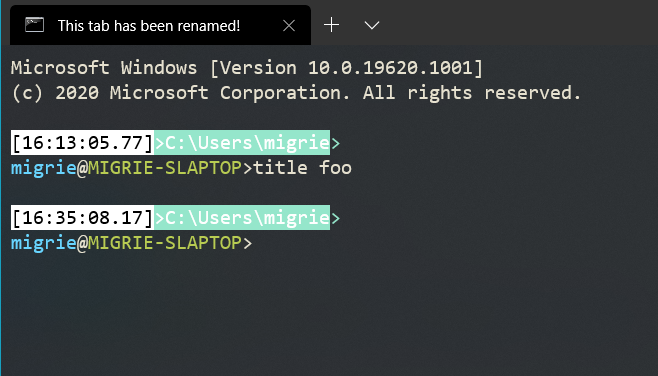
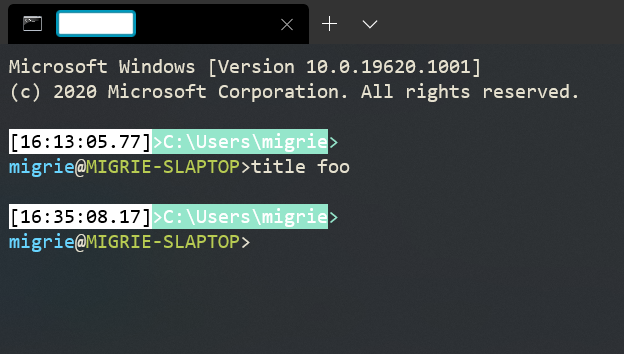
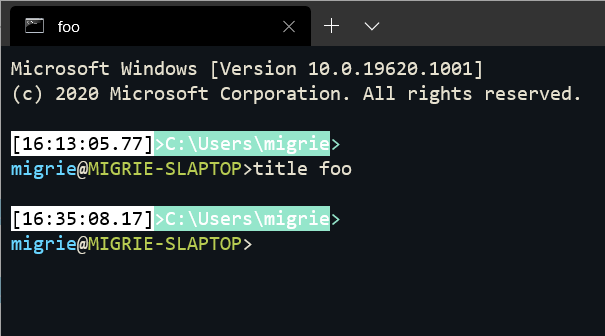
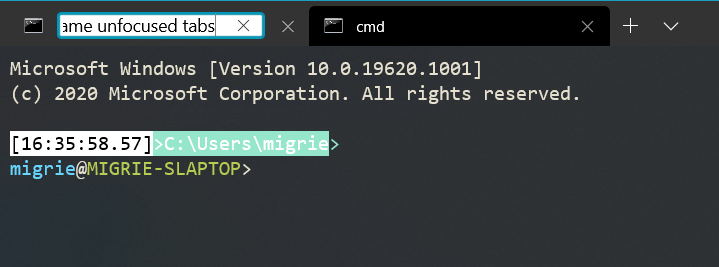
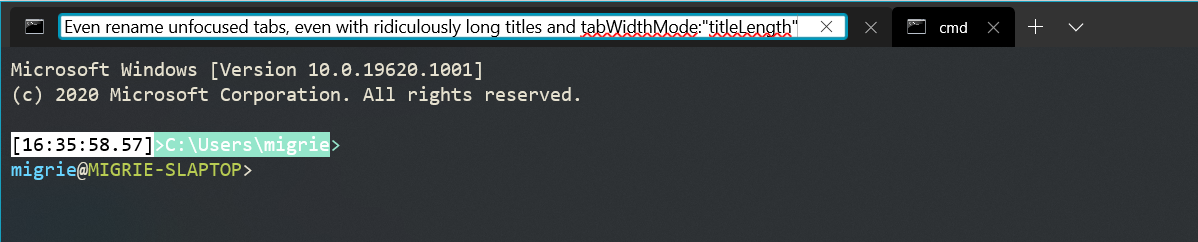
2020-05-28 23:06:17 +02:00
|
|
|
// - Set the text on the TabViewItem for this tab, and bubbles the new title
|
|
|
|
// value up to anyone listening for changes to our title. Callers can
|
|
|
|
// listen for the title change with a PropertyChanged even handler.
|
2020-02-04 22:51:11 +01:00
|
|
|
// Arguments:
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
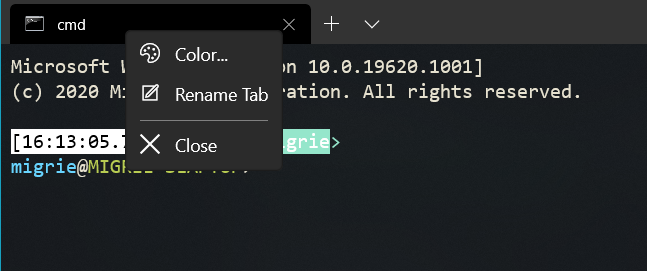
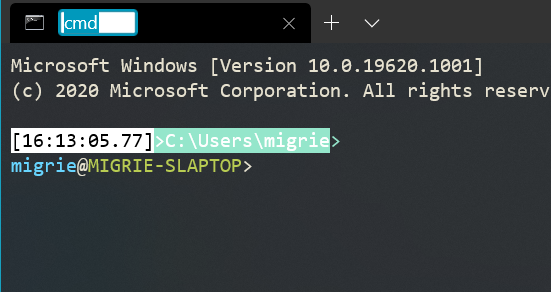
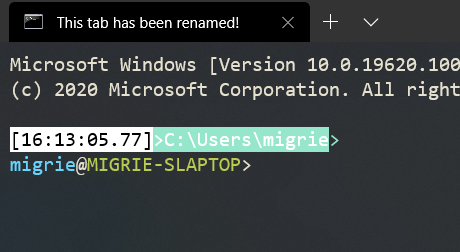
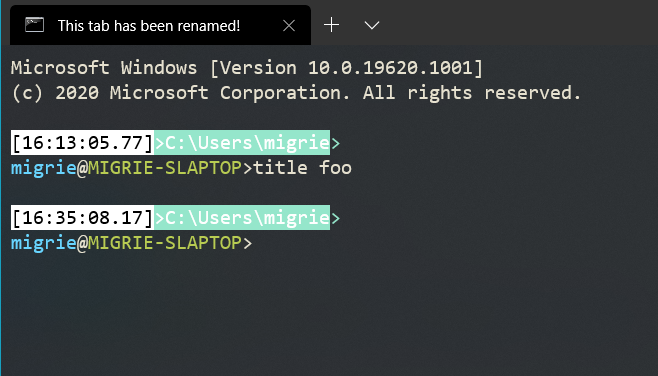
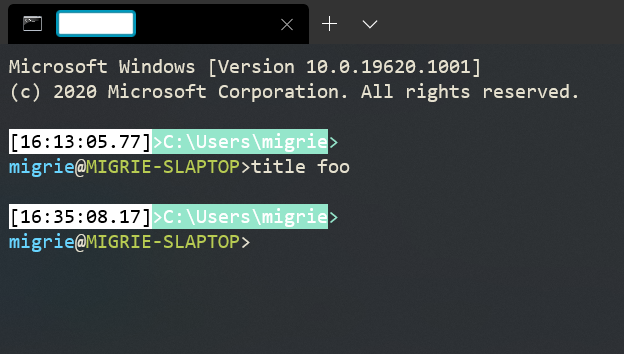
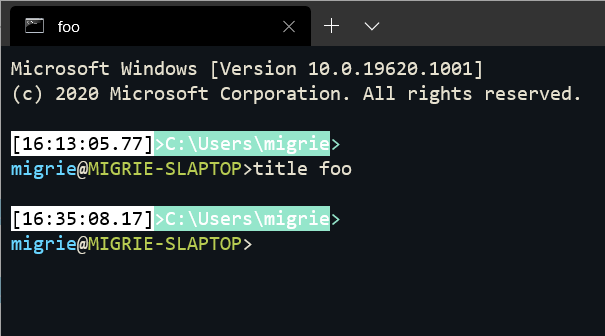
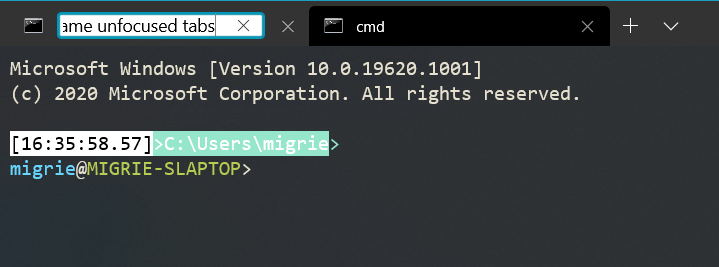
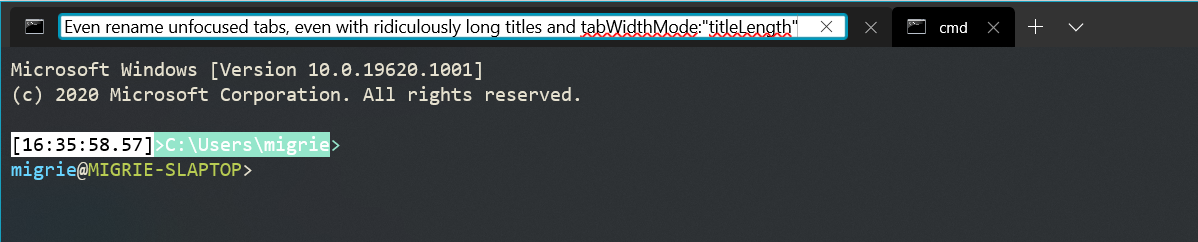
2020-05-28 23:06:17 +02:00
|
|
|
// - <none>
|
2020-02-04 22:51:11 +01:00
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
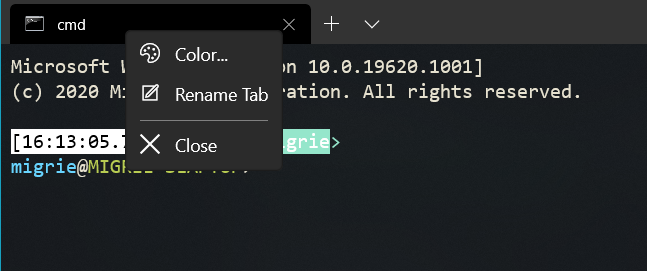
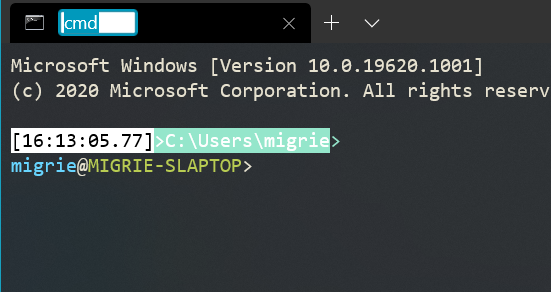
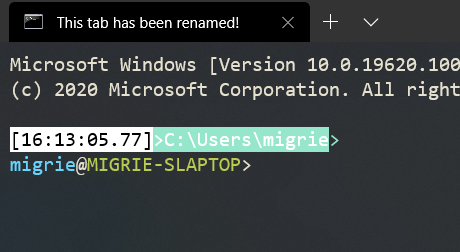
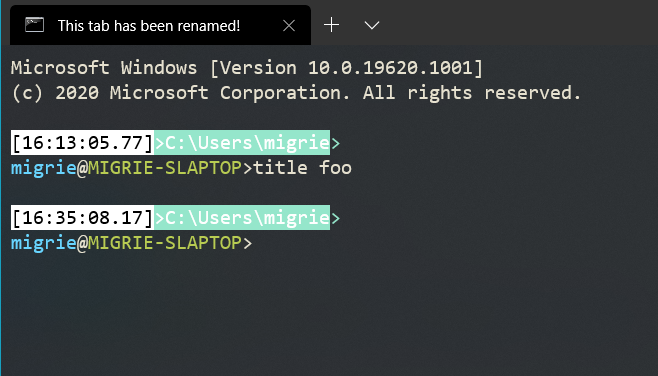
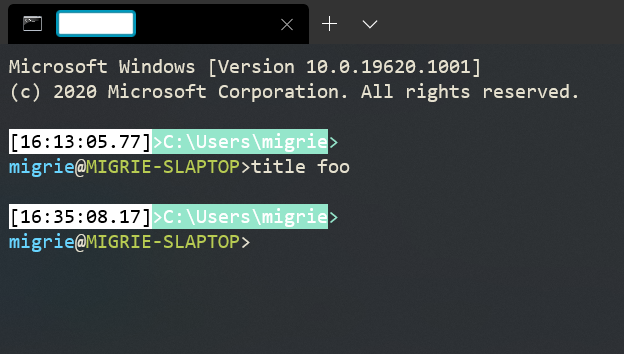
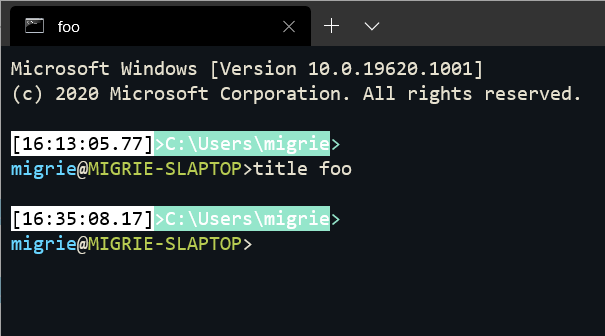
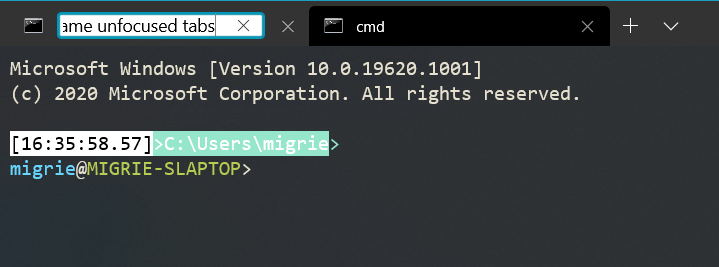
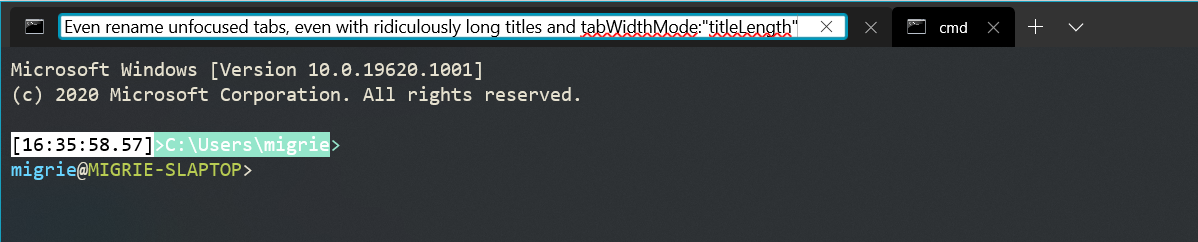
2020-05-28 23:06:17 +02:00
|
|
|
winrt::fire_and_forget Tab::_UpdateTitle()
|
2020-02-04 22:51:11 +01:00
|
|
|
{
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
co_await winrt::resume_foreground(_tabViewItem.Dispatcher());
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
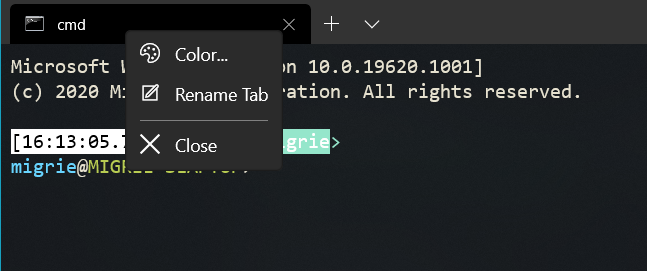
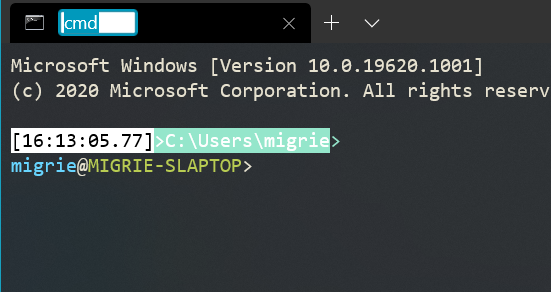
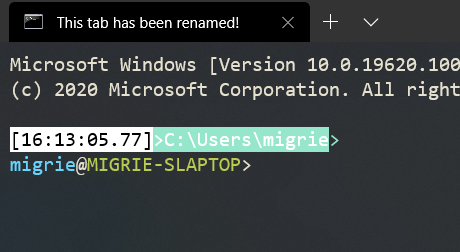
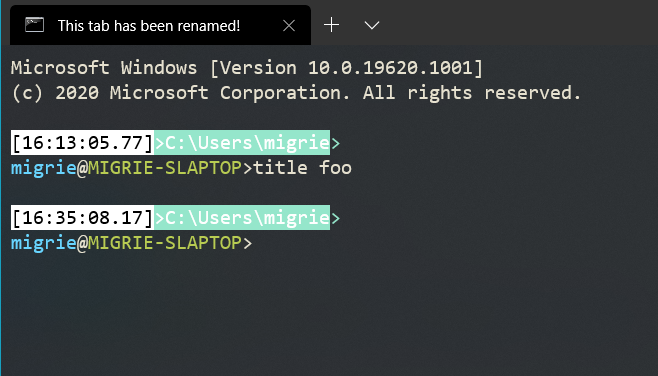
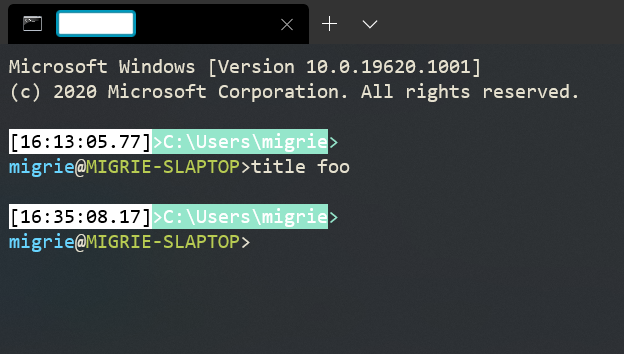
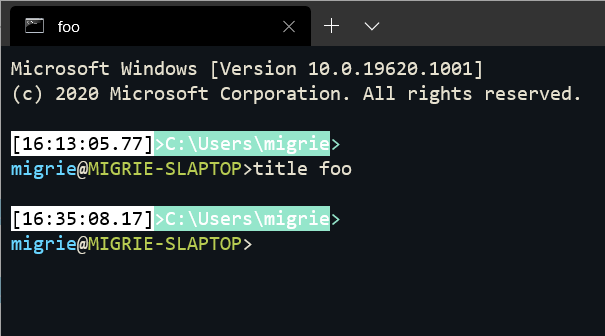
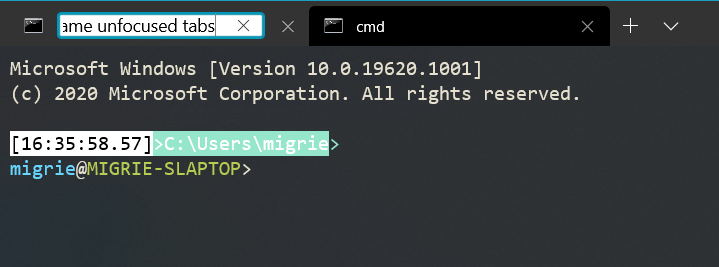
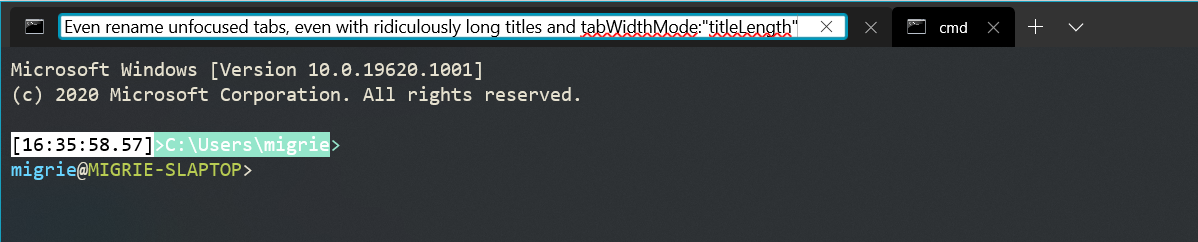
2020-05-28 23:06:17 +02:00
|
|
|
// Bubble our current tab text to anyone who's listening for changes.
|
|
|
|
Title(GetActiveTitle());
|
|
|
|
|
|
|
|
// Update the UI to reflect the changed
|
|
|
|
_UpdateTabHeader();
|
2020-02-04 22:51:11 +01:00
|
|
|
}
|
|
|
|
}
|
2019-08-21 00:38:45 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Move the viewport of the terminal up or down a number of lines. Negative
|
|
|
|
// values of `delta` will move the view up, and positive values will move
|
|
|
|
// the viewport down.
|
|
|
|
// Arguments:
|
|
|
|
// - delta: a number of lines to move the viewport relative to the current viewport.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
winrt::fire_and_forget Tab::Scroll(const int delta)
|
|
|
|
{
|
|
|
|
auto control = GetActiveTerminalControl();
|
2019-06-07 23:56:44 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
co_await winrt::resume_foreground(control.Dispatcher());
|
2020-01-08 22:19:23 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
const auto currentOffset = control.GetScrollOffset();
|
Fix scrollbar doesn't update viewport after window resize (#3344)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
Fixes a bug where scrolling up/down doesn't update the viewport after the window is resized and in other cases. Also changes other things, please read the detailed description.
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #1494
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [ ] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #xxx
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
There are two ways scroll can happen:
- the user scrolls using the scroll bar and the `Terminal` is notified
- the `Terminal` changed the viewport and the scroll bar is updated to reflect the change
The code to notify the `Terminal` that the user scrolled is in the event handler for when the scroll bar's value changes. However this poses a problem because it means that when the `Terminal` changes the viewport, the scroll bar is updated so it would then also notify the `Terminal` that the scroll changed. But it already knows because it's coming from itself!
To fix this, the `TermControl` class had a member called `_lastScrollOffset` that would be set when the `Terminal` decides to change the viewport so that the event handler for the scroll bar could check the new scroll value against `_lastScrollOffset` and if it matches, then everything is fine and there is nothing to update.
This is what happens when the `Terminal` changes the viewport:
1. set `_lastScrollOffset`
2. dispatch job on the UI thread: update the scrollbar which is going to call the event handler which is going to check for `_lastScrollOffset` and clear it
There are two bugs introduced by this approach:
1. (I am not sure about this.) The dispatcher appears to store jobs in a LIFO stack so it sometimes reorders the "update the scrollbar" jobs when there are too many. When I run `1..10000` on PowerShell, then I get this from the event handler (format: `_lastScrollOffset newValue`):
```
8988 8988
8989 8989
8990 8990
8992 8991
8993 8992
...
9001 8997
9001 8998
9001 8999
9001 9000
9001 9001
9001 8985
9001 8968
9001 8953
...
9001 7242
9001 7226
9001 7210
```
This causes the following issues:
1. `_lastScrollOffset` wouldn't be reset because it wouldn't be equal to the current scroll bar value (see example above) so the next scrolls wouldn't do anything as the event handler would still be waiting for an event with the good scroll bar value which would never happen because it happened earlier
2. the `TermControl` would notify the `Terminal` about its own scroll
2. If the `Terminal` didn't actually changed its viewport but still called the `TermControl::_TerminalScrollPositionChanged` method, then it would set the `_lastScrollOffset` member as usual but the scroll bar value change event handler would not be called because it is only called when the value actually changes so the `_lastScrollOffset` member wouldn't be cleared and subsequent scroll bar value change events would be ignored because again the event handler would still be waiting for an event with the good scroll bar value which would never happen. This is actually the reason for #1494: when the window is resized, the `Terminal` will call `TermControl::_TerminalScrollPositionChanged` even if the scroll position didn't actually change (https://github.com/microsoft/terminal/blob/444de5b166d800b17c90510cde8664aefffcb236/src/cascadia/TerminalCore/Terminal.cpp#L183). Maybe this should also be fixed in another PR?
I replaced `_lastScrollOffset` by a flag `_isTerminalInitiatedScroll`. I set the flag just before and unset it just after the terminal changes the scrollbar on the UI thread to eliminate the race conditions and the bug when the scroll bar's value doesn't actually change.
Other changes:
- I also fixed a potential bug where if the user scrolls just after the terminal updates the viewport, it would en up ignoring the user scroll. To do this, when the user scrolls, I cancel any update with `_willUpdateScrollBarToMatchViewport`.
- I also removed the original `ScrollViewport` method because it was not used anywhere and I think it can potentially create confusion (and therefore bugs) because this method updates the viewport but not the scroll bar unlike `KeyboardScrollViewport` which functions as you would expect. I then renamed `KeyboardScrollViewport` into `ScrollViewport`. So, now, there is only one method to scroll the viewport from the `TermControl`. Please, tell me if this shouldn't be in this PR.
- I also removed `_terminal->UserScrollViewport(viewTop);` in the `KeyboardScrollViewport` method because it will be updated later anyways in the scroll bar's value change event handler because of the `_scrollBar.Value(viewTop);`.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
I tested manually by doing this:
- For bug 1:
1. Start the terminal
2. Run the `1..30000` command in PowerShell and wait for it to end (maybe more if you have a fast computer?)
3. Hold left click on the scrollbar slider and start moving it
- For bug 2:
1. Start the terminal
2. Run the `1..100` command in PowerShell and wait for it to end
3. Resize the window horizontally
4. Hold left click on the scrollbar slider and start moving it
Without this patch, the viewport doesn't update.
With the patch, the viewport updates correctly.
2020-02-11 00:15:30 +01:00
|
|
|
control.ScrollViewport(currentOffset + delta);
|
2020-02-04 22:51:11 +01:00
|
|
|
}
|
Enable resizing the panes with the keyboard. (#1207)
Adds the ability to resize panes with the keyboard.
This is accomplished by making the Column/RowDefinitions for a Pane use `GridLengthHelper::FromPixels` to set their size. We store a pair of floats that represents the relative amount that each pane takes out of the parent pane. When the window is resized, we use that percentage to figure out the new size of each child in pixels, and manually size each column.
Then, when the user presses the keybindings for resizePane{Left/Right/Up/Down}, we'll adjust those percentages, and resize the rows/cols as appropriate.
Currently, each pane adjusts the width/height by 5% of the total size at a time. I am not in love with this, but it works for now. I think when we get support for keybindings with arbitrary arg blobs, then we could do either a percent movement, or a number of characters at a time. The number of characters one would be trickier, because we'd have to get the focused control, and get the number of pixels per character, as adjacent panes might not have the same font sizes.
2019-07-10 15:27:12 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Determines whether the focused pane has sufficient space to be split.
|
|
|
|
// Arguments:
|
|
|
|
// - splitType: The type of split we want to create.
|
|
|
|
// Return Value:
|
|
|
|
// - True if the focused pane can be split. False otherwise.
|
|
|
|
bool Tab::CanSplitPane(winrt::TerminalApp::SplitState splitType)
|
|
|
|
{
|
|
|
|
return _activePane->CanSplit(splitType);
|
|
|
|
}
|
Enable resizing the panes with the keyboard. (#1207)
Adds the ability to resize panes with the keyboard.
This is accomplished by making the Column/RowDefinitions for a Pane use `GridLengthHelper::FromPixels` to set their size. We store a pair of floats that represents the relative amount that each pane takes out of the parent pane. When the window is resized, we use that percentage to figure out the new size of each child in pixels, and manually size each column.
Then, when the user presses the keybindings for resizePane{Left/Right/Up/Down}, we'll adjust those percentages, and resize the rows/cols as appropriate.
Currently, each pane adjusts the width/height by 5% of the total size at a time. I am not in love with this, but it works for now. I think when we get support for keybindings with arbitrary arg blobs, then we could do either a percent movement, or a number of characters at a time. The number of characters one would be trickier, because we'd have to get the focused control, and get the number of pixels per character, as adjacent panes might not have the same font sizes.
2019-07-10 15:27:12 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Split the focused pane in our tree of panes, and place the
|
|
|
|
// given TermControl into the newly created pane.
|
|
|
|
// Arguments:
|
|
|
|
// - splitType: The type of split we want to create.
|
|
|
|
// - profile: The profile GUID to associate with the newly created pane.
|
|
|
|
// - control: A TermControl to use in the new pane.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::SplitPane(winrt::TerminalApp::SplitState splitType, const GUID& profile, TermControl& control)
|
|
|
|
{
|
|
|
|
auto [first, second] = _activePane->Split(splitType, profile, control);
|
|
|
|
_activePane = first;
|
|
|
|
_AttachEventHandlersToControl(control);
|
|
|
|
|
|
|
|
// Add a event handlers to the new panes' GotFocus event. When the pane
|
|
|
|
// gains focus, we'll mark it as the new active pane.
|
|
|
|
_AttachEventHandlersToPane(first);
|
|
|
|
_AttachEventHandlersToPane(second);
|
Process actions sync. on startup; don't dupe nonexistent profile (#5090)
This PR has evolved to encapsulate two related fixes that I can't really
untie anymore.
#2455 - Duplicating a tab that doesn't exist anymore
This was the bug I was originally fixing in #4429.
When the user tries to `duplicateTab` with a profile that doesn't exist
anymore (like might happen after a settings reload), don't crash.
As I was going about adding tests for this, got blocked by the fact that
the Terminal couldn't open _any_ panes while the `TerminalPage` was size
0x0. This had two theoretical solutions:
* Fake the `TerminalPage` into thinking it had a real size in the test -
probably possible, though I'm unsure how it would work in practice.
* Change `Pane`s to not require an `ActualWidth`, `ActualHeight` on
initialization.
Fortuately, the second option was something else that was already on my
backlog of bugs.
#4618 - `wt` command-line can't consistently parse more than one arg
Presently, the Terminal just arbitrarily dispatches a bunch of handlers
to try and handle all the commands provided on the commandline. That's
lead to a bunch of reports that not all the commands will always get
executed, nor will they all get executed in the same order.
This PR also changes the `TerminalPage` to be able to dispatch all the
commands sequentially, all at once in the startup. No longer will there
be a hot second where the commands seem to execute themselves in from of
the user - they'll all happen behind the scenes on startup.
This involved a couple other changes areound the `TerminalPage`
* I had to make sure that panes could be opened at a 0x0 size. Now they
use a star sizing based off the percentage of the parent they're
supposed to consume, so that when the parent _does_ get laid out,
they'll take the appropriate size of that parent.
* I had to do some math ahead of time to try and calculate what a
`SplitState::Automatic` would be evaluated as, despite the fact that
we don't actually know how big the pane will be.
* I had to ensure that `focus-tab` commands appropriately mark a single
tab as focused while we're in startup, without roundtripping to the
Dispatcher thread and back
## References
#4429 - the original PR for #2455
#5047 - a follow-up task from discussion in #4429
#4953 - a PR for making panes use star sizing, which was immensly
helpful for this PR.
## Detailed Description of the Pull Request / Additional comments
`CascadiaSettings::BuildSettings` can throw if the GUID doesn't exist.
This wraps those calls up with a try/catch.
It also adds a couple tests - a few `SettingsTests` for try/catching
this state. It also adds a XAML-y test in `TabTests` that creates a
`TerminalPage` and then performs som UI-like actions on it. This test
required a minor change to how we generate the new tab dropdown - in the
tests, `Application::Current()` is _not_ a `TerminalApp::App`, so it
doesn't have a `Logic()` to query. So wrap that in a try/catch as well.
While working on these tests, I found that we'd crash pretty agressively
for mysterious reasons if the TestHostApp became focused while the test
was running. This was due to a call in
`TSFInputControl::NotifyFocusEnter` that would callback to
`TSFInputControl::_layoutRequested`, which would crash on setting the
`MaxSize` of the canvas to a negative value. This PR includes a hotfix
for that bug as well.
## Validation Steps Performed
* Manual testing with a _lot_ of commands in a commandline
* run the tests
* Team tested in selfhost
Closes #2455
Closes #4618
2020-03-26 01:03:32 +01:00
|
|
|
|
|
|
|
// Immediately update our tracker of the focused pane now. If we're
|
|
|
|
// splitting panes during startup (from a commandline), then it's
|
|
|
|
// possible that the focus events won't propagate immediately. Updating
|
|
|
|
// the focus here will give the same effect though.
|
|
|
|
_UpdateActivePane(second);
|
2020-02-04 22:51:11 +01:00
|
|
|
}
|
2019-07-17 16:30:15 +02:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - See Pane::CalcSnappedDimension
|
|
|
|
float Tab::CalcSnappedDimension(const bool widthOrHeight, const float dimension) const
|
|
|
|
{
|
|
|
|
return _rootPane->CalcSnappedDimension(widthOrHeight, dimension);
|
|
|
|
}
|
2020-01-23 23:12:20 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Update the size of our panes to fill the new given size. This happens when
|
|
|
|
// the window is resized.
|
|
|
|
// Arguments:
|
|
|
|
// - newSize: the amount of space that the panes have to fill now.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::ResizeContent(const winrt::Windows::Foundation::Size& newSize)
|
|
|
|
{
|
2020-02-10 21:40:01 +01:00
|
|
|
// NOTE: This _must_ be called on the root pane, so that it can propagate
|
2020-02-04 22:51:11 +01:00
|
|
|
// throughout the entire tree.
|
|
|
|
_rootPane->ResizeContent(newSize);
|
|
|
|
}
|
Decouple "Active Terminal" and "Focused Control" (#3540)
## Summary of the Pull Request
Unties the concept of "focused control" from "active control".
Previously, we were exclusively using the "Focused" state of `TermControl`s to determine which one was active. This was fraught with gotchas - if anything else became focused, then suddenly there was _no_ pane focused in the Tab. This happened especially frequently if the user clicked on a tab to focus the window. Furthermore, in experimental branches with more UI added to the Terminal (such as [dev/migrie/f/2046-command-palette](https://github.com/microsoft/terminal/tree/dev/migrie/f/2046-command-palette)), when these UIs were added to the Terminal, they'd take focus, which again meant that there was no focused pane.
This fixes these issue by having each Tab manually track which Pane is active in that tab. The Tab is now the arbiter of who in the tree is "active". Panes still track this state, for them to be able to MoveFocus appropriately.
It also contains a related fix to prevent the tab separator from stealing focus from the TermControl. This required us to set the color of the un-focused Pane border to some color other that Transparent, so I went with the TabViewBackground. Panes now look like the following:
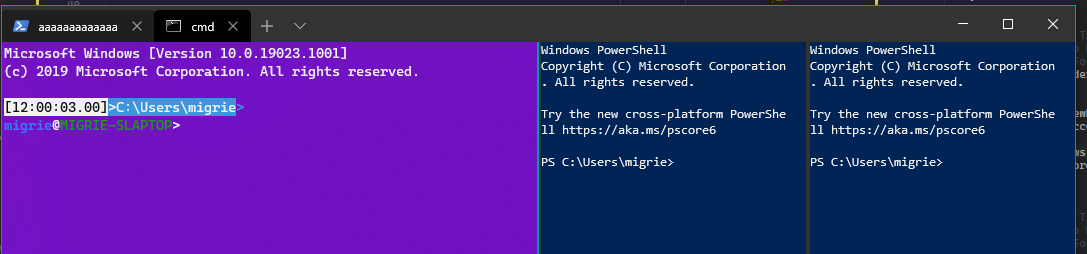
## References
See also: #2046
## PR Checklist
* [x] Closes #1205
* [x] Closes #522
* [x] Closes #999
* [x] I work here
* [😢] Tests added/passed
* [n/a] Requires documentation to be updated
## Validation Steps Performed
Tested manually opening panes, closing panes, clicking around panes, the whole dance.
---------------------------------------------------
* this is janky but is close for some reason?
* This is _almost_ right to solve #1205
If I want to double up and also fix #522 (which I do), then I need to also
* when a tab GetsFocus, send the focus instead to the Pane
* When the border is clicked on, focus that pane's control
And like a lot of cleanup, because this is horrifying
* hey this autorevoker is really nice
* Encapsulate Pane::pfnGotFocus
* Propogate the events back up on close
* Encapsulate Tab::pfnFocusChanged, and clean up TerminalPage a bit
* Mostly just code cleanup, commenting
* This works to hittest on the borders
If the border is `Transparent`, then it can't hittest for Tapped events, and it'll fall through (to someone)
THis at least works, but looks garish
* Match the pane border to the TabViewHeader
* Fix a bit of dead code and a bad copy-pasta
* This _works_ to use a winrt event, but it's dirty
* Clean up everything from the winrt::event debacle.
* This is dead code that shouldn't have been there
* Turn Tab's callback into a winrt::event as well
2019-11-18 22:41:25 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Attempt to move a separator between panes, as to resize each child on
|
|
|
|
// either size of the separator. See Pane::ResizePane for details.
|
|
|
|
// Arguments:
|
|
|
|
// - direction: The direction to move the separator in.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::ResizePane(const winrt::TerminalApp::Direction& direction)
|
|
|
|
{
|
2020-02-10 21:40:01 +01:00
|
|
|
// NOTE: This _must_ be called on the root pane, so that it can propagate
|
2020-02-04 22:51:11 +01:00
|
|
|
// throughout the entire tree.
|
|
|
|
_rootPane->ResizePane(direction);
|
|
|
|
}
|
Fixed self reference capture in Tab and TerminalPage (#3835)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
Every lambda capture in `Tab` and `TerminalPage` has been changed from capturing raw `this` to `std::weak_ptr<Tab>` or `winrt::weak_ref<TerminalPage>`. Lambda bodies have been changed to check the weak reference before use.
Capturing raw `this` in `Tab`'s [title change event handler](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/Tab.cpp#L299) was the root cause of #3776, and is fixed in this PR among other instance of raw `this` capture.
The lambda fixes to `TerminalPage` are unrelated to the core issue addressed in the PR checklist. Because I was already editing `TerminalPage`, figured I'd do a [weak_ref pass](https://github.com/microsoft/terminal/issues/3776#issuecomment-560575575).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3776, potentially #2248, likely closes others
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3776
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
`Tab` now inherits from `enable_shared_from_this`, which enable accessing `Tab` objects as `std::weak_ptr<Tab>` objects. All instances of lambdas capturing `this` now capture `std::weak_ptr<Tab>` instead. `TerminalPage` is a WinRT type which supports `winrt::weak_ref<TerminalPage>`. All previous instance of `TerminalPage` lambdas capturing `this` has been replaced to capture `winrt::weak_ref<TerminalPage>`. These weak pointers/references can only be created after object construction necessitating for `Tab` a new function called after construction to bind lambdas.
Any anomalous crash related to the following functionality during closing a tab or WT may be fixed by this PR:
- Tab icon updating
- Tab text updating
- Tab dragging
- Clicking new tab button
- Changing active pane
- Closing an active tab
- Clicking on a tab
- Creating the new tab flyout menu
Sorry about all the commits. Will fix my fork after this PR! 😅
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Attempted to repro the steps indicated in issue #3776 with the new changes and failed. When before the changes, the issue could consistently be reproed.
2019-12-06 00:18:22 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Attempt to move focus between panes, as to focus the child on
|
|
|
|
// the other side of the separator. See Pane::NavigateFocus for details.
|
|
|
|
// Arguments:
|
|
|
|
// - direction: The direction to move the focus in.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::NavigateFocus(const winrt::TerminalApp::Direction& direction)
|
|
|
|
{
|
2020-02-10 21:40:01 +01:00
|
|
|
// NOTE: This _must_ be called on the root pane, so that it can propagate
|
2020-02-04 22:51:11 +01:00
|
|
|
// throughout the entire tree.
|
|
|
|
_rootPane->NavigateFocus(direction);
|
|
|
|
}
|
Decouple "Active Terminal" and "Focused Control" (#3540)
## Summary of the Pull Request
Unties the concept of "focused control" from "active control".
Previously, we were exclusively using the "Focused" state of `TermControl`s to determine which one was active. This was fraught with gotchas - if anything else became focused, then suddenly there was _no_ pane focused in the Tab. This happened especially frequently if the user clicked on a tab to focus the window. Furthermore, in experimental branches with more UI added to the Terminal (such as [dev/migrie/f/2046-command-palette](https://github.com/microsoft/terminal/tree/dev/migrie/f/2046-command-palette)), when these UIs were added to the Terminal, they'd take focus, which again meant that there was no focused pane.
This fixes these issue by having each Tab manually track which Pane is active in that tab. The Tab is now the arbiter of who in the tree is "active". Panes still track this state, for them to be able to MoveFocus appropriately.
It also contains a related fix to prevent the tab separator from stealing focus from the TermControl. This required us to set the color of the un-focused Pane border to some color other that Transparent, so I went with the TabViewBackground. Panes now look like the following:
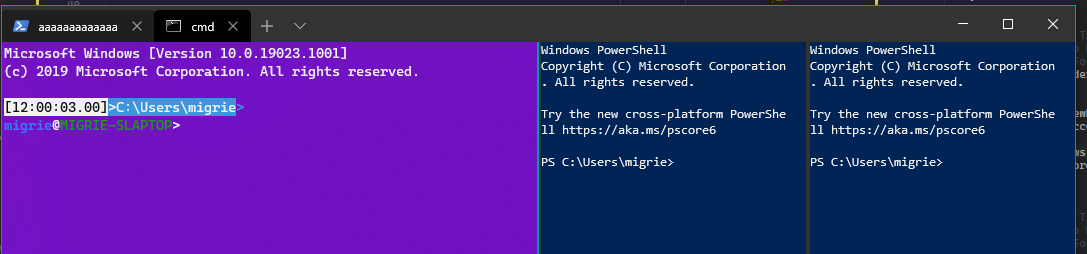
## References
See also: #2046
## PR Checklist
* [x] Closes #1205
* [x] Closes #522
* [x] Closes #999
* [x] I work here
* [😢] Tests added/passed
* [n/a] Requires documentation to be updated
## Validation Steps Performed
Tested manually opening panes, closing panes, clicking around panes, the whole dance.
---------------------------------------------------
* this is janky but is close for some reason?
* This is _almost_ right to solve #1205
If I want to double up and also fix #522 (which I do), then I need to also
* when a tab GetsFocus, send the focus instead to the Pane
* When the border is clicked on, focus that pane's control
And like a lot of cleanup, because this is horrifying
* hey this autorevoker is really nice
* Encapsulate Pane::pfnGotFocus
* Propogate the events back up on close
* Encapsulate Tab::pfnFocusChanged, and clean up TerminalPage a bit
* Mostly just code cleanup, commenting
* This works to hittest on the borders
If the border is `Transparent`, then it can't hittest for Tapped events, and it'll fall through (to someone)
THis at least works, but looks garish
* Match the pane border to the TabViewHeader
* Fix a bit of dead code and a bad copy-pasta
* This _works_ to use a winrt event, but it's dirty
* Clean up everything from the winrt::event debacle.
* This is dead code that shouldn't have been there
* Turn Tab's callback into a winrt::event as well
2019-11-18 22:41:25 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Prepares this tab for being removed from the UI hierarchy by shutting down all active connections.
|
|
|
|
void Tab::Shutdown()
|
|
|
|
{
|
|
|
|
_rootPane->Shutdown();
|
|
|
|
}
|
Fixed self reference capture in Tab and TerminalPage (#3835)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
Every lambda capture in `Tab` and `TerminalPage` has been changed from capturing raw `this` to `std::weak_ptr<Tab>` or `winrt::weak_ref<TerminalPage>`. Lambda bodies have been changed to check the weak reference before use.
Capturing raw `this` in `Tab`'s [title change event handler](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/Tab.cpp#L299) was the root cause of #3776, and is fixed in this PR among other instance of raw `this` capture.
The lambda fixes to `TerminalPage` are unrelated to the core issue addressed in the PR checklist. Because I was already editing `TerminalPage`, figured I'd do a [weak_ref pass](https://github.com/microsoft/terminal/issues/3776#issuecomment-560575575).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3776, potentially #2248, likely closes others
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3776
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
`Tab` now inherits from `enable_shared_from_this`, which enable accessing `Tab` objects as `std::weak_ptr<Tab>` objects. All instances of lambdas capturing `this` now capture `std::weak_ptr<Tab>` instead. `TerminalPage` is a WinRT type which supports `winrt::weak_ref<TerminalPage>`. All previous instance of `TerminalPage` lambdas capturing `this` has been replaced to capture `winrt::weak_ref<TerminalPage>`. These weak pointers/references can only be created after object construction necessitating for `Tab` a new function called after construction to bind lambdas.
Any anomalous crash related to the following functionality during closing a tab or WT may be fixed by this PR:
- Tab icon updating
- Tab text updating
- Tab dragging
- Clicking new tab button
- Changing active pane
- Closing an active tab
- Clicking on a tab
- Creating the new tab flyout menu
Sorry about all the commits. Will fix my fork after this PR! 😅
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Attempted to repro the steps indicated in issue #3776 with the new changes and failed. When before the changes, the issue could consistently be reproed.
2019-12-06 00:18:22 +01:00
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Closes the currently focused pane in this tab. If it's the last pane in
|
|
|
|
// this tab, our Closed event will be fired (at a later time) for anyone
|
|
|
|
// registered as a handler of our close event.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::ClosePane()
|
|
|
|
{
|
|
|
|
_activePane->Close();
|
|
|
|
}
|
Converts Dispatcher().RunAsync to WinRT Coroutines (#4051)
<!-- Enter a brief description/summary of your PR here. What does it fix/what does it change/how was it tested (even manually, if necessary)? -->
## Summary of the Pull Request
This PR turns all* instances of `Dispatcher().RunAsync` to WinRT coroutines 👌.
This was good coding fodder to fill my plane ride ✈️. Enjoy your holidays everyone!
*With the exception of three functions whose signatures cannot be changed due to inheritance and function overriding in `TermControlAutomationPeer` [`L44`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L44), [`L58`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L58), [`L72`](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalControl/TermControlAutomationPeer.cpp#L72).
<!-- Other than the issue solved, is this relevant to any other issues/existing PRs? -->
## References
<!-- Please review the items on the PR checklist before submitting-->
## PR Checklist
* [x] Closes #3919
* [x] CLA signed. If not, go over [here](https://cla.opensource.microsoft.com/microsoft/Terminal) and sign the CLA
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
* [x] I've discussed this with core contributors already. If not checked, I'm ready to accept this work might be rejected in favor of a different grand plan. Issue number where discussion took place: #3919
<!-- Provide a more detailed description of the PR, other things fixed or any additional comments/features here -->
## Detailed Description of the Pull Request / Additional comments
My thought pattern here was to minimally disturb the existing code where possible. So where I could, I converted existing functions into coroutine using functions (like in the [core example](https://github.com/microsoft/terminal/issues/3919#issue-536598706)). For ~the most part~ all instances, I used the format where [`this` is accessed safely within a locked scope](https://github.com/microsoft/terminal/issues/3919#issuecomment-564730620). Some function signatures were changed to take objects by value instead of reference, so the coroutines don't crash when the objects are accessed past their original lifetime. The [copy](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1132) and [paste](https://github.com/microsoft/terminal/blob/master/src/cascadia/TerminalApp/TerminalPage.cpp#L1170) event handler entry points were originally set to a high priority; however, the WinRT coroutines don't appear to support a priority scheme so this priority setting was not preserved in the translation.
<!-- Describe how you validated the behavior. Add automated tests wherever possible, but list manual validation steps taken as well -->
## Validation Steps Performed
Compiles and runs, and for every event with a clear trigger repro, I triggered it to ensure crashes weren't introduced.
2020-01-10 04:29:49 +01:00
|
|
|
|
2020-06-24 22:07:41 +02:00
|
|
|
void Tab::SetTabText(winrt::hstring title)
|
|
|
|
{
|
|
|
|
_runtimeTabText = title;
|
|
|
|
_UpdateTitle();
|
|
|
|
}
|
|
|
|
|
|
|
|
void Tab::ResetTabText()
|
|
|
|
{
|
|
|
|
_runtimeTabText = L"";
|
|
|
|
_UpdateTitle();
|
|
|
|
}
|
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Register any event handlers that we may need with the given TermControl.
|
|
|
|
// This should be called on each and every TermControl that we add to the tree
|
|
|
|
// of Panes in this tab. We'll add events too:
|
|
|
|
// * notify us when the control's title changed, so we can update our own
|
|
|
|
// title (if necessary)
|
|
|
|
// Arguments:
|
|
|
|
// - control: the TermControl to add events to.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_AttachEventHandlersToControl(const TermControl& control)
|
|
|
|
{
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
|
|
|
|
control.TitleChanged([weakThis](auto newTitle) {
|
|
|
|
// Check if Tab's lifetime has expired
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
|
|
|
// The title of the control changed, but not necessarily the title of the tab.
|
|
|
|
// Set the tab's text to the active panes' text.
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
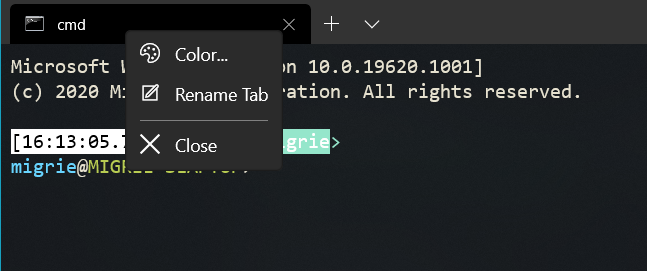
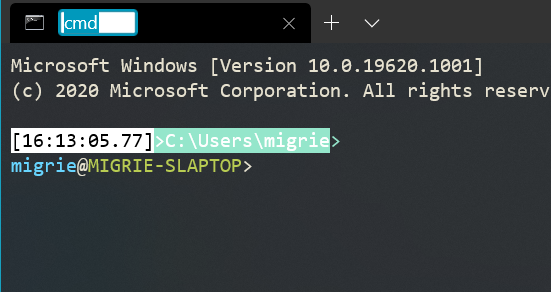
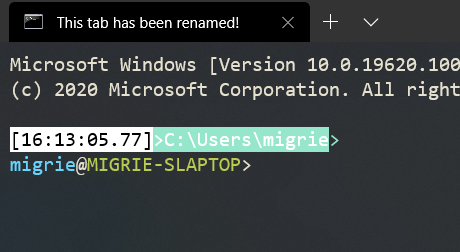
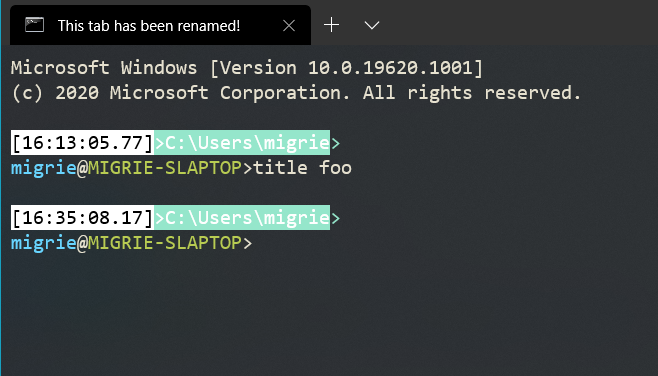
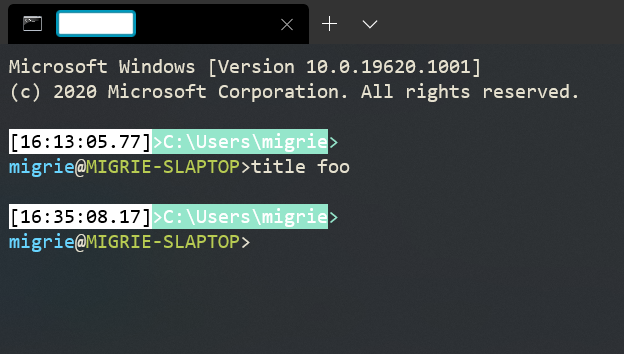
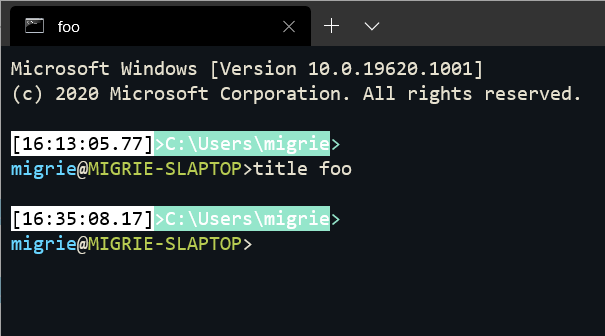
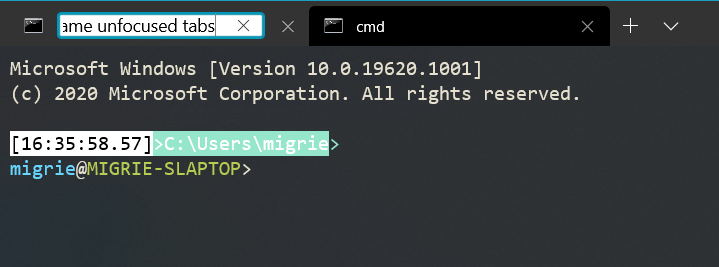
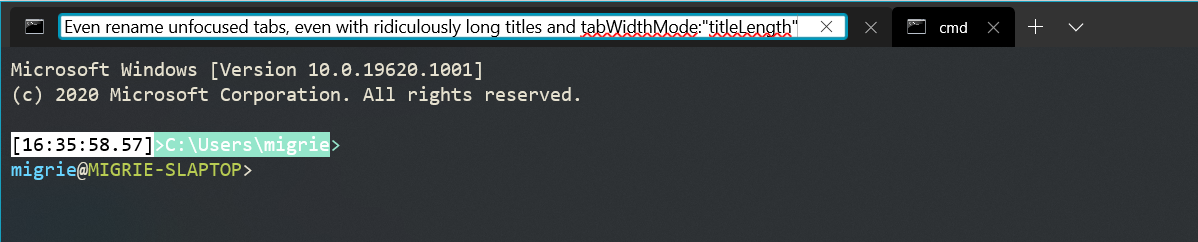
2020-05-28 23:06:17 +02:00
|
|
|
tab->_UpdateTitle();
|
2020-02-04 22:51:11 +01:00
|
|
|
}
|
|
|
|
});
|
|
|
|
|
|
|
|
// This is called when the terminal changes its font size or sets it for the first
|
|
|
|
// time (because when we just create terminal via its ctor it has invalid font size).
|
|
|
|
// On the latter event, we tell the root pane to resize itself so that its descendants
|
|
|
|
// (including ourself) can properly snap to character grids. In future, we may also
|
|
|
|
// want to do that on regular font changes.
|
|
|
|
control.FontSizeChanged([this](const int /* fontWidth */,
|
|
|
|
const int /* fontHeight */,
|
|
|
|
const bool isInitialChange) {
|
|
|
|
if (isInitialChange)
|
|
|
|
{
|
|
|
|
_rootPane->Relayout();
|
|
|
|
}
|
|
|
|
});
|
|
|
|
}
|
Decouple "Active Terminal" and "Focused Control" (#3540)
## Summary of the Pull Request
Unties the concept of "focused control" from "active control".
Previously, we were exclusively using the "Focused" state of `TermControl`s to determine which one was active. This was fraught with gotchas - if anything else became focused, then suddenly there was _no_ pane focused in the Tab. This happened especially frequently if the user clicked on a tab to focus the window. Furthermore, in experimental branches with more UI added to the Terminal (such as [dev/migrie/f/2046-command-palette](https://github.com/microsoft/terminal/tree/dev/migrie/f/2046-command-palette)), when these UIs were added to the Terminal, they'd take focus, which again meant that there was no focused pane.
This fixes these issue by having each Tab manually track which Pane is active in that tab. The Tab is now the arbiter of who in the tree is "active". Panes still track this state, for them to be able to MoveFocus appropriately.
It also contains a related fix to prevent the tab separator from stealing focus from the TermControl. This required us to set the color of the un-focused Pane border to some color other that Transparent, so I went with the TabViewBackground. Panes now look like the following:
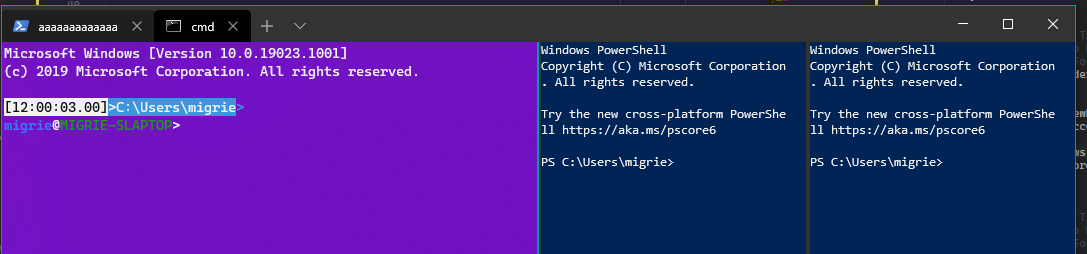
## References
See also: #2046
## PR Checklist
* [x] Closes #1205
* [x] Closes #522
* [x] Closes #999
* [x] I work here
* [😢] Tests added/passed
* [n/a] Requires documentation to be updated
## Validation Steps Performed
Tested manually opening panes, closing panes, clicking around panes, the whole dance.
---------------------------------------------------
* this is janky but is close for some reason?
* This is _almost_ right to solve #1205
If I want to double up and also fix #522 (which I do), then I need to also
* when a tab GetsFocus, send the focus instead to the Pane
* When the border is clicked on, focus that pane's control
And like a lot of cleanup, because this is horrifying
* hey this autorevoker is really nice
* Encapsulate Pane::pfnGotFocus
* Propogate the events back up on close
* Encapsulate Tab::pfnFocusChanged, and clean up TerminalPage a bit
* Mostly just code cleanup, commenting
* This works to hittest on the borders
If the border is `Transparent`, then it can't hittest for Tapped events, and it'll fall through (to someone)
THis at least works, but looks garish
* Match the pane border to the TabViewHeader
* Fix a bit of dead code and a bad copy-pasta
* This _works_ to use a winrt event, but it's dirty
* Clean up everything from the winrt::event debacle.
* This is dead code that shouldn't have been there
* Turn Tab's callback into a winrt::event as well
2019-11-18 22:41:25 +01:00
|
|
|
|
Process actions sync. on startup; don't dupe nonexistent profile (#5090)
This PR has evolved to encapsulate two related fixes that I can't really
untie anymore.
#2455 - Duplicating a tab that doesn't exist anymore
This was the bug I was originally fixing in #4429.
When the user tries to `duplicateTab` with a profile that doesn't exist
anymore (like might happen after a settings reload), don't crash.
As I was going about adding tests for this, got blocked by the fact that
the Terminal couldn't open _any_ panes while the `TerminalPage` was size
0x0. This had two theoretical solutions:
* Fake the `TerminalPage` into thinking it had a real size in the test -
probably possible, though I'm unsure how it would work in practice.
* Change `Pane`s to not require an `ActualWidth`, `ActualHeight` on
initialization.
Fortuately, the second option was something else that was already on my
backlog of bugs.
#4618 - `wt` command-line can't consistently parse more than one arg
Presently, the Terminal just arbitrarily dispatches a bunch of handlers
to try and handle all the commands provided on the commandline. That's
lead to a bunch of reports that not all the commands will always get
executed, nor will they all get executed in the same order.
This PR also changes the `TerminalPage` to be able to dispatch all the
commands sequentially, all at once in the startup. No longer will there
be a hot second where the commands seem to execute themselves in from of
the user - they'll all happen behind the scenes on startup.
This involved a couple other changes areound the `TerminalPage`
* I had to make sure that panes could be opened at a 0x0 size. Now they
use a star sizing based off the percentage of the parent they're
supposed to consume, so that when the parent _does_ get laid out,
they'll take the appropriate size of that parent.
* I had to do some math ahead of time to try and calculate what a
`SplitState::Automatic` would be evaluated as, despite the fact that
we don't actually know how big the pane will be.
* I had to ensure that `focus-tab` commands appropriately mark a single
tab as focused while we're in startup, without roundtripping to the
Dispatcher thread and back
## References
#4429 - the original PR for #2455
#5047 - a follow-up task from discussion in #4429
#4953 - a PR for making panes use star sizing, which was immensly
helpful for this PR.
## Detailed Description of the Pull Request / Additional comments
`CascadiaSettings::BuildSettings` can throw if the GUID doesn't exist.
This wraps those calls up with a try/catch.
It also adds a couple tests - a few `SettingsTests` for try/catching
this state. It also adds a XAML-y test in `TabTests` that creates a
`TerminalPage` and then performs som UI-like actions on it. This test
required a minor change to how we generate the new tab dropdown - in the
tests, `Application::Current()` is _not_ a `TerminalApp::App`, so it
doesn't have a `Logic()` to query. So wrap that in a try/catch as well.
While working on these tests, I found that we'd crash pretty agressively
for mysterious reasons if the TestHostApp became focused while the test
was running. This was due to a call in
`TSFInputControl::NotifyFocusEnter` that would callback to
`TSFInputControl::_layoutRequested`, which would crash on setting the
`MaxSize` of the canvas to a negative value. This PR includes a hotfix
for that bug as well.
## Validation Steps Performed
* Manual testing with a _lot_ of commands in a commandline
* run the tests
* Team tested in selfhost
Closes #2455
Closes #4618
2020-03-26 01:03:32 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Mark the given pane as the active pane in this tab. All other panes
|
|
|
|
// will be marked as inactive. We'll also update our own UI state to
|
|
|
|
// reflect this newly active pane.
|
|
|
|
// Arguments:
|
|
|
|
// - pane: a Pane to mark as active.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_UpdateActivePane(std::shared_ptr<Pane> pane)
|
|
|
|
{
|
|
|
|
// Clear the active state of the entire tree, and mark only the pane as active.
|
|
|
|
_rootPane->ClearActive();
|
|
|
|
_activePane = pane;
|
|
|
|
_activePane->SetActive();
|
|
|
|
|
|
|
|
// Update our own title text to match the newly-active pane.
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
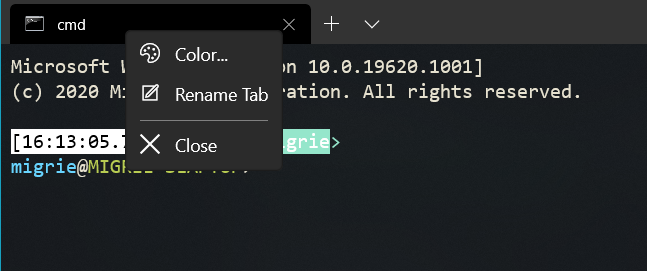
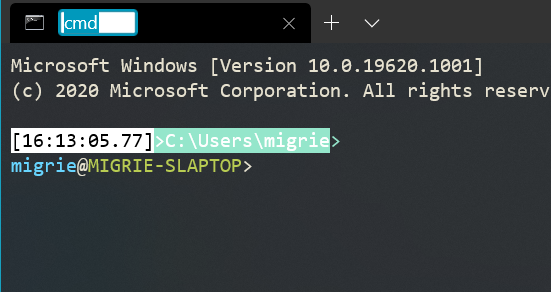
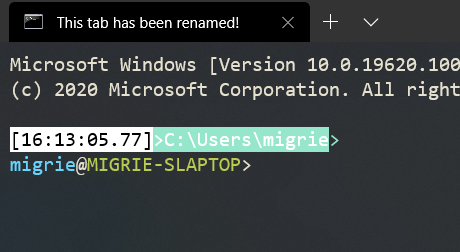
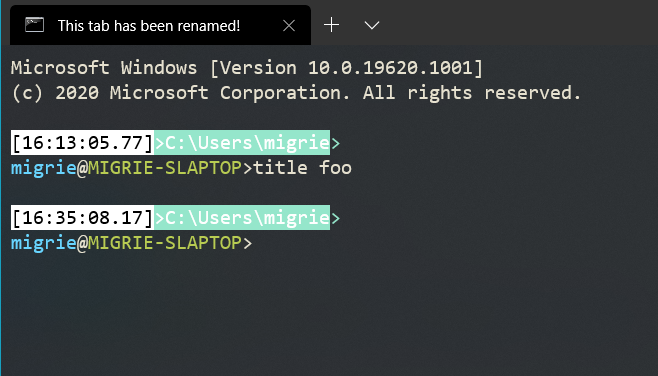
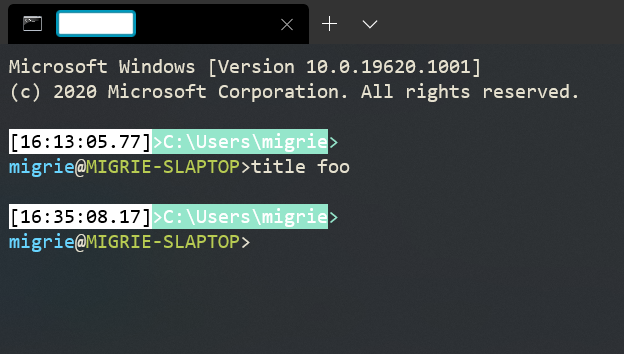
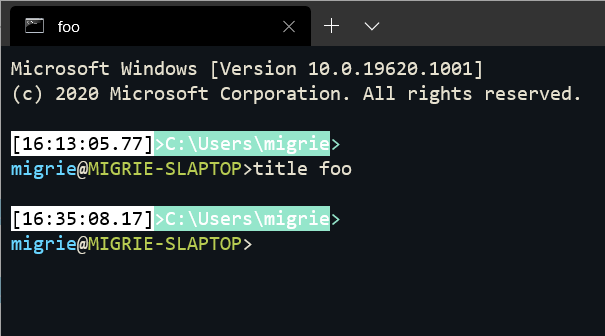
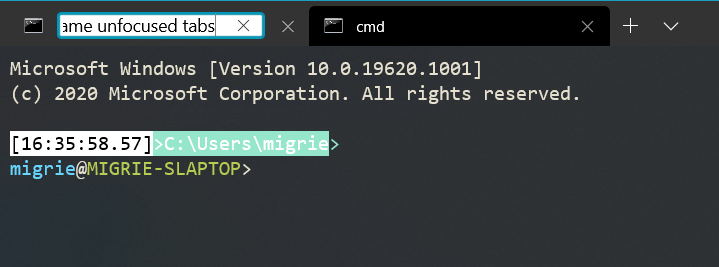
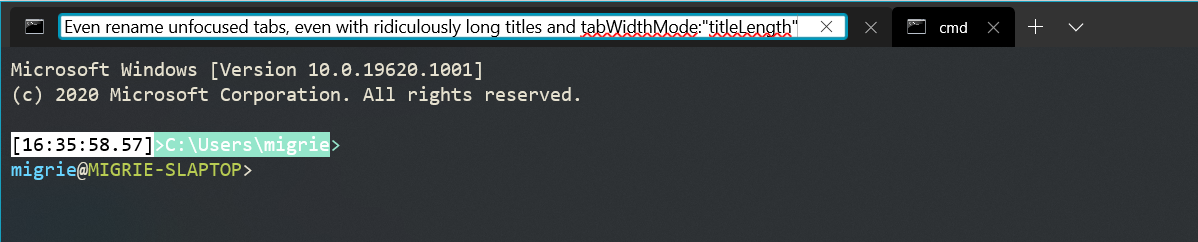
2020-05-28 23:06:17 +02:00
|
|
|
_UpdateTitle();
|
Process actions sync. on startup; don't dupe nonexistent profile (#5090)
This PR has evolved to encapsulate two related fixes that I can't really
untie anymore.
#2455 - Duplicating a tab that doesn't exist anymore
This was the bug I was originally fixing in #4429.
When the user tries to `duplicateTab` with a profile that doesn't exist
anymore (like might happen after a settings reload), don't crash.
As I was going about adding tests for this, got blocked by the fact that
the Terminal couldn't open _any_ panes while the `TerminalPage` was size
0x0. This had two theoretical solutions:
* Fake the `TerminalPage` into thinking it had a real size in the test -
probably possible, though I'm unsure how it would work in practice.
* Change `Pane`s to not require an `ActualWidth`, `ActualHeight` on
initialization.
Fortuately, the second option was something else that was already on my
backlog of bugs.
#4618 - `wt` command-line can't consistently parse more than one arg
Presently, the Terminal just arbitrarily dispatches a bunch of handlers
to try and handle all the commands provided on the commandline. That's
lead to a bunch of reports that not all the commands will always get
executed, nor will they all get executed in the same order.
This PR also changes the `TerminalPage` to be able to dispatch all the
commands sequentially, all at once in the startup. No longer will there
be a hot second where the commands seem to execute themselves in from of
the user - they'll all happen behind the scenes on startup.
This involved a couple other changes areound the `TerminalPage`
* I had to make sure that panes could be opened at a 0x0 size. Now they
use a star sizing based off the percentage of the parent they're
supposed to consume, so that when the parent _does_ get laid out,
they'll take the appropriate size of that parent.
* I had to do some math ahead of time to try and calculate what a
`SplitState::Automatic` would be evaluated as, despite the fact that
we don't actually know how big the pane will be.
* I had to ensure that `focus-tab` commands appropriately mark a single
tab as focused while we're in startup, without roundtripping to the
Dispatcher thread and back
## References
#4429 - the original PR for #2455
#5047 - a follow-up task from discussion in #4429
#4953 - a PR for making panes use star sizing, which was immensly
helpful for this PR.
## Detailed Description of the Pull Request / Additional comments
`CascadiaSettings::BuildSettings` can throw if the GUID doesn't exist.
This wraps those calls up with a try/catch.
It also adds a couple tests - a few `SettingsTests` for try/catching
this state. It also adds a XAML-y test in `TabTests` that creates a
`TerminalPage` and then performs som UI-like actions on it. This test
required a minor change to how we generate the new tab dropdown - in the
tests, `Application::Current()` is _not_ a `TerminalApp::App`, so it
doesn't have a `Logic()` to query. So wrap that in a try/catch as well.
While working on these tests, I found that we'd crash pretty agressively
for mysterious reasons if the TestHostApp became focused while the test
was running. This was due to a call in
`TSFInputControl::NotifyFocusEnter` that would callback to
`TSFInputControl::_layoutRequested`, which would crash on setting the
`MaxSize` of the canvas to a negative value. This PR includes a hotfix
for that bug as well.
## Validation Steps Performed
* Manual testing with a _lot_ of commands in a commandline
* run the tests
* Team tested in selfhost
Closes #2455
Closes #4618
2020-03-26 01:03:32 +01:00
|
|
|
|
|
|
|
// Raise our own ActivePaneChanged event.
|
|
|
|
_ActivePaneChangedHandlers();
|
|
|
|
}
|
|
|
|
|
2020-02-04 22:51:11 +01:00
|
|
|
// Method Description:
|
|
|
|
// - Add an event handler to this pane's GotFocus event. When that pane gains
|
|
|
|
// focus, we'll mark it as the new active pane. We'll also query the title of
|
|
|
|
// that pane when it's focused to set our own text, and finally, we'll trigger
|
|
|
|
// our own ActivePaneChanged event.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_AttachEventHandlersToPane(std::shared_ptr<Pane> pane)
|
|
|
|
{
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
|
|
|
|
pane->GotFocus([weakThis](std::shared_ptr<Pane> sender) {
|
|
|
|
// Do nothing if the Tab's lifetime is expired or pane isn't new.
|
|
|
|
auto tab{ weakThis.get() };
|
|
|
|
|
|
|
|
if (tab && sender != tab->_activePane)
|
|
|
|
{
|
Process actions sync. on startup; don't dupe nonexistent profile (#5090)
This PR has evolved to encapsulate two related fixes that I can't really
untie anymore.
#2455 - Duplicating a tab that doesn't exist anymore
This was the bug I was originally fixing in #4429.
When the user tries to `duplicateTab` with a profile that doesn't exist
anymore (like might happen after a settings reload), don't crash.
As I was going about adding tests for this, got blocked by the fact that
the Terminal couldn't open _any_ panes while the `TerminalPage` was size
0x0. This had two theoretical solutions:
* Fake the `TerminalPage` into thinking it had a real size in the test -
probably possible, though I'm unsure how it would work in practice.
* Change `Pane`s to not require an `ActualWidth`, `ActualHeight` on
initialization.
Fortuately, the second option was something else that was already on my
backlog of bugs.
#4618 - `wt` command-line can't consistently parse more than one arg
Presently, the Terminal just arbitrarily dispatches a bunch of handlers
to try and handle all the commands provided on the commandline. That's
lead to a bunch of reports that not all the commands will always get
executed, nor will they all get executed in the same order.
This PR also changes the `TerminalPage` to be able to dispatch all the
commands sequentially, all at once in the startup. No longer will there
be a hot second where the commands seem to execute themselves in from of
the user - they'll all happen behind the scenes on startup.
This involved a couple other changes areound the `TerminalPage`
* I had to make sure that panes could be opened at a 0x0 size. Now they
use a star sizing based off the percentage of the parent they're
supposed to consume, so that when the parent _does_ get laid out,
they'll take the appropriate size of that parent.
* I had to do some math ahead of time to try and calculate what a
`SplitState::Automatic` would be evaluated as, despite the fact that
we don't actually know how big the pane will be.
* I had to ensure that `focus-tab` commands appropriately mark a single
tab as focused while we're in startup, without roundtripping to the
Dispatcher thread and back
## References
#4429 - the original PR for #2455
#5047 - a follow-up task from discussion in #4429
#4953 - a PR for making panes use star sizing, which was immensly
helpful for this PR.
## Detailed Description of the Pull Request / Additional comments
`CascadiaSettings::BuildSettings` can throw if the GUID doesn't exist.
This wraps those calls up with a try/catch.
It also adds a couple tests - a few `SettingsTests` for try/catching
this state. It also adds a XAML-y test in `TabTests` that creates a
`TerminalPage` and then performs som UI-like actions on it. This test
required a minor change to how we generate the new tab dropdown - in the
tests, `Application::Current()` is _not_ a `TerminalApp::App`, so it
doesn't have a `Logic()` to query. So wrap that in a try/catch as well.
While working on these tests, I found that we'd crash pretty agressively
for mysterious reasons if the TestHostApp became focused while the test
was running. This was due to a call in
`TSFInputControl::NotifyFocusEnter` that would callback to
`TSFInputControl::_layoutRequested`, which would crash on setting the
`MaxSize` of the canvas to a negative value. This PR includes a hotfix
for that bug as well.
## Validation Steps Performed
* Manual testing with a _lot_ of commands in a commandline
* run the tests
* Team tested in selfhost
Closes #2455
Closes #4618
2020-03-26 01:03:32 +01:00
|
|
|
tab->_UpdateActivePane(sender);
|
2020-02-04 22:51:11 +01:00
|
|
|
}
|
|
|
|
});
|
|
|
|
}
|
Decouple "Active Terminal" and "Focused Control" (#3540)
## Summary of the Pull Request
Unties the concept of "focused control" from "active control".
Previously, we were exclusively using the "Focused" state of `TermControl`s to determine which one was active. This was fraught with gotchas - if anything else became focused, then suddenly there was _no_ pane focused in the Tab. This happened especially frequently if the user clicked on a tab to focus the window. Furthermore, in experimental branches with more UI added to the Terminal (such as [dev/migrie/f/2046-command-palette](https://github.com/microsoft/terminal/tree/dev/migrie/f/2046-command-palette)), when these UIs were added to the Terminal, they'd take focus, which again meant that there was no focused pane.
This fixes these issue by having each Tab manually track which Pane is active in that tab. The Tab is now the arbiter of who in the tree is "active". Panes still track this state, for them to be able to MoveFocus appropriately.
It also contains a related fix to prevent the tab separator from stealing focus from the TermControl. This required us to set the color of the un-focused Pane border to some color other that Transparent, so I went with the TabViewBackground. Panes now look like the following:
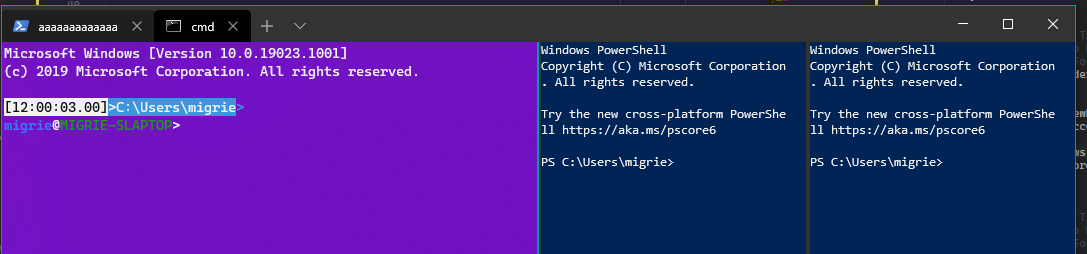
## References
See also: #2046
## PR Checklist
* [x] Closes #1205
* [x] Closes #522
* [x] Closes #999
* [x] I work here
* [😢] Tests added/passed
* [n/a] Requires documentation to be updated
## Validation Steps Performed
Tested manually opening panes, closing panes, clicking around panes, the whole dance.
---------------------------------------------------
* this is janky but is close for some reason?
* This is _almost_ right to solve #1205
If I want to double up and also fix #522 (which I do), then I need to also
* when a tab GetsFocus, send the focus instead to the Pane
* When the border is clicked on, focus that pane's control
And like a lot of cleanup, because this is horrifying
* hey this autorevoker is really nice
* Encapsulate Pane::pfnGotFocus
* Propogate the events back up on close
* Encapsulate Tab::pfnFocusChanged, and clean up TerminalPage a bit
* Mostly just code cleanup, commenting
* This works to hittest on the borders
If the border is `Transparent`, then it can't hittest for Tapped events, and it'll fall through (to someone)
THis at least works, but looks garish
* Match the pane border to the TabViewHeader
* Fix a bit of dead code and a bad copy-pasta
* This _works_ to use a winrt event, but it's dirty
* Clean up everything from the winrt::event debacle.
* This is dead code that shouldn't have been there
* Turn Tab's callback into a winrt::event as well
2019-11-18 22:41:25 +01:00
|
|
|
|
Process actions sync. on startup; don't dupe nonexistent profile (#5090)
This PR has evolved to encapsulate two related fixes that I can't really
untie anymore.
#2455 - Duplicating a tab that doesn't exist anymore
This was the bug I was originally fixing in #4429.
When the user tries to `duplicateTab` with a profile that doesn't exist
anymore (like might happen after a settings reload), don't crash.
As I was going about adding tests for this, got blocked by the fact that
the Terminal couldn't open _any_ panes while the `TerminalPage` was size
0x0. This had two theoretical solutions:
* Fake the `TerminalPage` into thinking it had a real size in the test -
probably possible, though I'm unsure how it would work in practice.
* Change `Pane`s to not require an `ActualWidth`, `ActualHeight` on
initialization.
Fortuately, the second option was something else that was already on my
backlog of bugs.
#4618 - `wt` command-line can't consistently parse more than one arg
Presently, the Terminal just arbitrarily dispatches a bunch of handlers
to try and handle all the commands provided on the commandline. That's
lead to a bunch of reports that not all the commands will always get
executed, nor will they all get executed in the same order.
This PR also changes the `TerminalPage` to be able to dispatch all the
commands sequentially, all at once in the startup. No longer will there
be a hot second where the commands seem to execute themselves in from of
the user - they'll all happen behind the scenes on startup.
This involved a couple other changes areound the `TerminalPage`
* I had to make sure that panes could be opened at a 0x0 size. Now they
use a star sizing based off the percentage of the parent they're
supposed to consume, so that when the parent _does_ get laid out,
they'll take the appropriate size of that parent.
* I had to do some math ahead of time to try and calculate what a
`SplitState::Automatic` would be evaluated as, despite the fact that
we don't actually know how big the pane will be.
* I had to ensure that `focus-tab` commands appropriately mark a single
tab as focused while we're in startup, without roundtripping to the
Dispatcher thread and back
## References
#4429 - the original PR for #2455
#5047 - a follow-up task from discussion in #4429
#4953 - a PR for making panes use star sizing, which was immensly
helpful for this PR.
## Detailed Description of the Pull Request / Additional comments
`CascadiaSettings::BuildSettings` can throw if the GUID doesn't exist.
This wraps those calls up with a try/catch.
It also adds a couple tests - a few `SettingsTests` for try/catching
this state. It also adds a XAML-y test in `TabTests` that creates a
`TerminalPage` and then performs som UI-like actions on it. This test
required a minor change to how we generate the new tab dropdown - in the
tests, `Application::Current()` is _not_ a `TerminalApp::App`, so it
doesn't have a `Logic()` to query. So wrap that in a try/catch as well.
While working on these tests, I found that we'd crash pretty agressively
for mysterious reasons if the TestHostApp became focused while the test
was running. This was due to a call in
`TSFInputControl::NotifyFocusEnter` that would callback to
`TSFInputControl::_layoutRequested`, which would crash on setting the
`MaxSize` of the canvas to a negative value. This PR includes a hotfix
for that bug as well.
## Validation Steps Performed
* Manual testing with a _lot_ of commands in a commandline
* run the tests
* Team tested in selfhost
Closes #2455
Closes #4618
2020-03-26 01:03:32 +01:00
|
|
|
// Method Description:
|
2020-05-04 22:57:12 +02:00
|
|
|
// - Creates a context menu attached to the tab.
|
|
|
|
// Currently contains elements allowing to select or
|
|
|
|
// to close the current tab
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_CreateContextMenu()
|
|
|
|
{
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
|
|
|
|
// Close
|
|
|
|
Controls::MenuFlyoutItem closeTabMenuItem;
|
|
|
|
Controls::FontIcon closeSymbol;
|
|
|
|
closeSymbol.FontFamily(Media::FontFamily{ L"Segoe MDL2 Assets" });
|
|
|
|
closeSymbol.Glyph(L"\xE8BB");
|
|
|
|
|
|
|
|
closeTabMenuItem.Click([weakThis](auto&&, auto&&) {
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
|
|
|
tab->_rootPane->Close();
|
|
|
|
}
|
|
|
|
});
|
|
|
|
closeTabMenuItem.Text(RS_(L"TabClose"));
|
|
|
|
closeTabMenuItem.Icon(closeSymbol);
|
|
|
|
|
|
|
|
// "Color..."
|
|
|
|
Controls::MenuFlyoutItem chooseColorMenuItem;
|
|
|
|
Controls::FontIcon colorPickSymbol;
|
|
|
|
colorPickSymbol.FontFamily(Media::FontFamily{ L"Segoe MDL2 Assets" });
|
|
|
|
colorPickSymbol.Glyph(L"\xE790");
|
|
|
|
|
|
|
|
chooseColorMenuItem.Click([weakThis](auto&&, auto&&) {
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
Add `setTabColor` and `openTabColorPicker` actions (#6567)
## Summary of the Pull Request
Adds a pair of `ShortcutAction`s for setting the tab color.
* `setTabColor`: This changes the color of the current tab to the provided color, or can be used to clear the color.
* `openTabColorPicker`: This keybinding immediately activates the tab color picker for the currently focused tab.
## References
## PR Checklist
* [x] scratches my own itch
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/69
## Detailed Description of the Pull Request / Additional comments
## Validation Steps Performed
* hey look there are tests
* Tested with the following:
```json
// { "command": "setTabColor", "keys": [ "alt+c" ] },
{ "keys": "ctrl+alt+c", "command": { "action": "setTabColor", "color": "#123456" } },
{ "keys": "alt+shift+c", "command": { "action": "setTabColor", "color": null} },
{ "keys": "alt+c", "command": "openTabColorPicker" },
```
2020-06-25 15:06:21 +02:00
|
|
|
tab->ActivateColorPicker();
|
2020-05-04 22:57:12 +02:00
|
|
|
}
|
|
|
|
});
|
|
|
|
chooseColorMenuItem.Text(RS_(L"TabColorChoose"));
|
|
|
|
chooseColorMenuItem.Icon(colorPickSymbol);
|
|
|
|
|
|
|
|
// Color Picker (it's convenient to have it here)
|
|
|
|
_tabColorPickup.ColorSelected([weakThis](auto newTabColor) {
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
Add `setTabColor` and `openTabColorPicker` actions (#6567)
## Summary of the Pull Request
Adds a pair of `ShortcutAction`s for setting the tab color.
* `setTabColor`: This changes the color of the current tab to the provided color, or can be used to clear the color.
* `openTabColorPicker`: This keybinding immediately activates the tab color picker for the currently focused tab.
## References
## PR Checklist
* [x] scratches my own itch
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/69
## Detailed Description of the Pull Request / Additional comments
## Validation Steps Performed
* hey look there are tests
* Tested with the following:
```json
// { "command": "setTabColor", "keys": [ "alt+c" ] },
{ "keys": "ctrl+alt+c", "command": { "action": "setTabColor", "color": "#123456" } },
{ "keys": "alt+shift+c", "command": { "action": "setTabColor", "color": null} },
{ "keys": "alt+c", "command": "openTabColorPicker" },
```
2020-06-25 15:06:21 +02:00
|
|
|
tab->SetTabColor(newTabColor);
|
2020-05-04 22:57:12 +02:00
|
|
|
}
|
|
|
|
});
|
|
|
|
|
|
|
|
_tabColorPickup.ColorCleared([weakThis]() {
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
Add `setTabColor` and `openTabColorPicker` actions (#6567)
## Summary of the Pull Request
Adds a pair of `ShortcutAction`s for setting the tab color.
* `setTabColor`: This changes the color of the current tab to the provided color, or can be used to clear the color.
* `openTabColorPicker`: This keybinding immediately activates the tab color picker for the currently focused tab.
## References
## PR Checklist
* [x] scratches my own itch
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/69
## Detailed Description of the Pull Request / Additional comments
## Validation Steps Performed
* hey look there are tests
* Tested with the following:
```json
// { "command": "setTabColor", "keys": [ "alt+c" ] },
{ "keys": "ctrl+alt+c", "command": { "action": "setTabColor", "color": "#123456" } },
{ "keys": "alt+shift+c", "command": { "action": "setTabColor", "color": null} },
{ "keys": "alt+c", "command": "openTabColorPicker" },
```
2020-06-25 15:06:21 +02:00
|
|
|
tab->ResetTabColor();
|
2020-05-04 22:57:12 +02:00
|
|
|
}
|
|
|
|
});
|
|
|
|
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
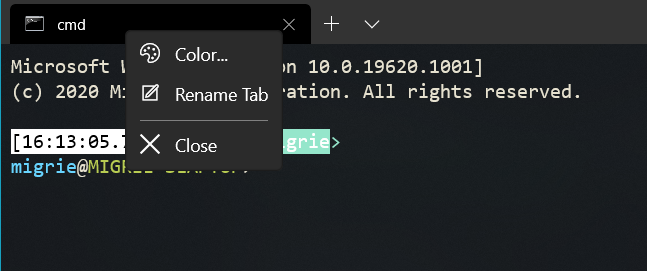
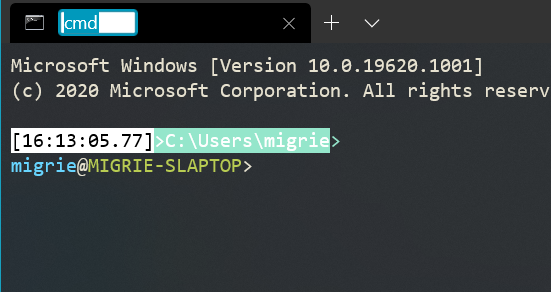
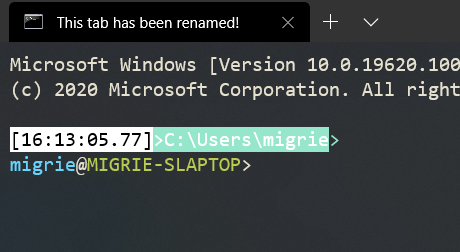
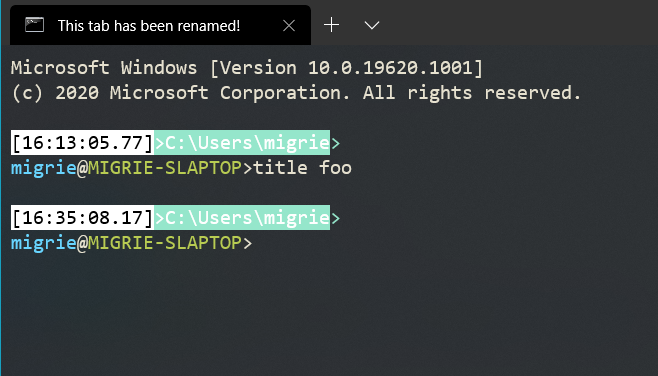
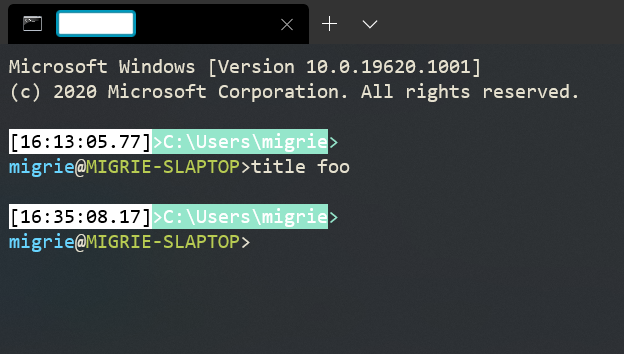
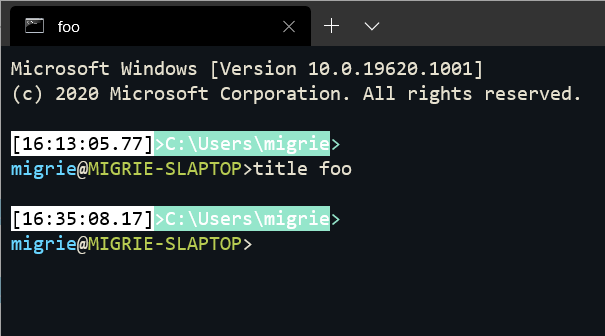
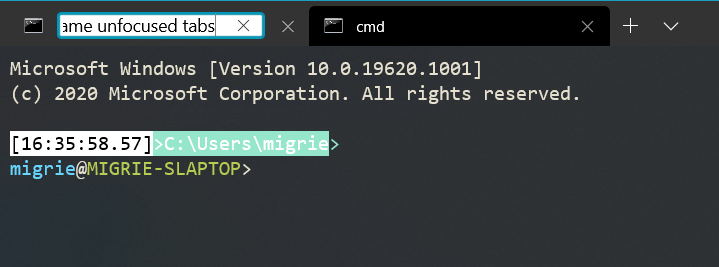
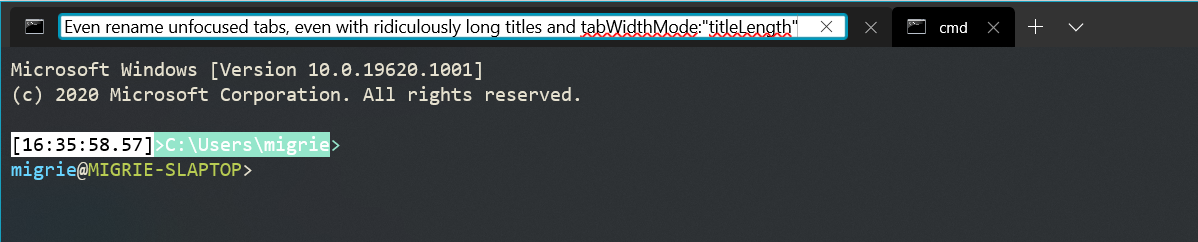
2020-05-28 23:06:17 +02:00
|
|
|
Controls::MenuFlyoutItem renameTabMenuItem;
|
|
|
|
{
|
|
|
|
// "Rename Tab"
|
|
|
|
Controls::FontIcon renameTabSymbol;
|
|
|
|
renameTabSymbol.FontFamily(Media::FontFamily{ L"Segoe MDL2 Assets" });
|
|
|
|
renameTabSymbol.Glyph(L"\xE932"); // Label
|
|
|
|
|
|
|
|
renameTabMenuItem.Click([weakThis](auto&&, auto&&) {
|
|
|
|
if (auto tab{ weakThis.get() })
|
|
|
|
{
|
|
|
|
tab->_inRename = true;
|
|
|
|
tab->_UpdateTabHeader();
|
|
|
|
}
|
|
|
|
});
|
|
|
|
renameTabMenuItem.Text(RS_(L"RenameTabText"));
|
|
|
|
renameTabMenuItem.Icon(renameTabSymbol);
|
|
|
|
}
|
|
|
|
|
2020-05-04 22:57:12 +02:00
|
|
|
// Build the menu
|
|
|
|
Controls::MenuFlyout newTabFlyout;
|
|
|
|
Controls::MenuFlyoutSeparator menuSeparator;
|
|
|
|
newTabFlyout.Items().Append(chooseColorMenuItem);
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
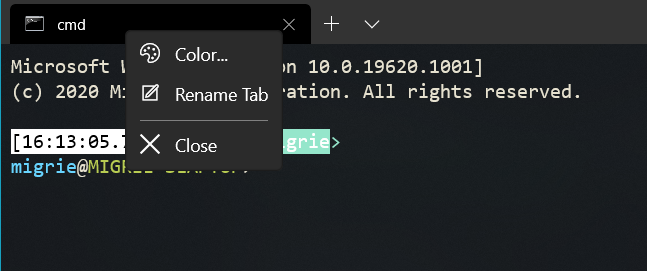
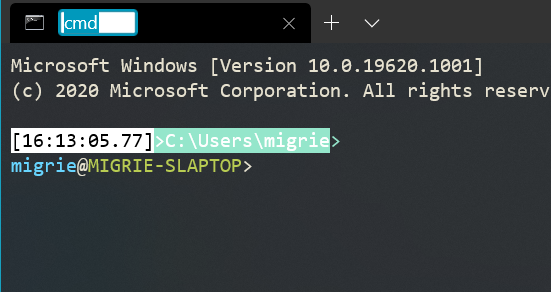
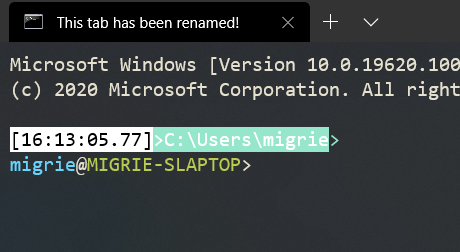
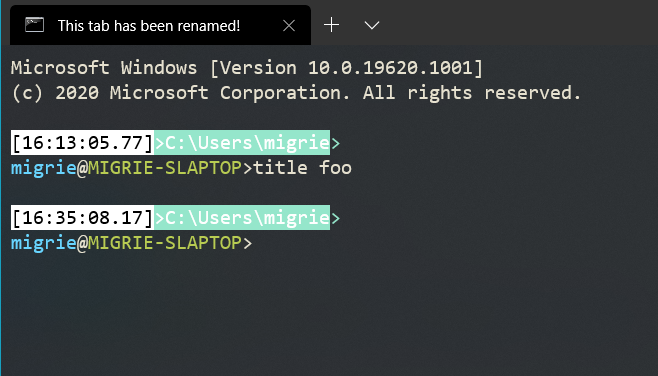
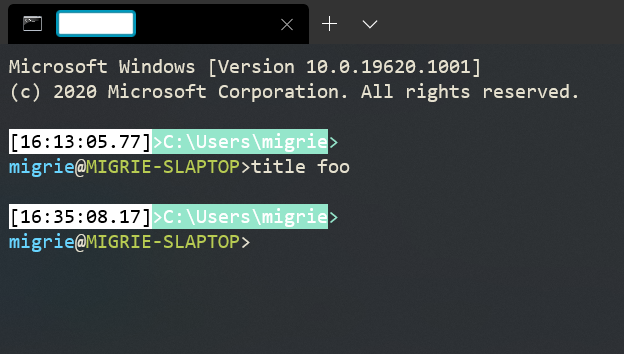
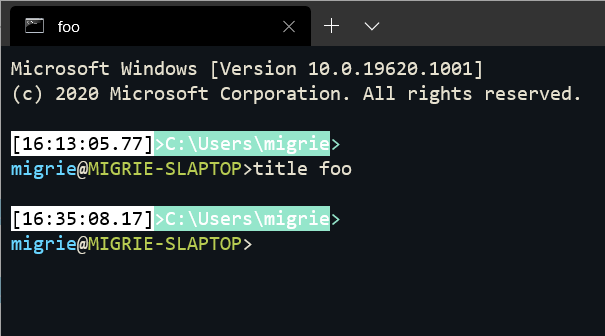
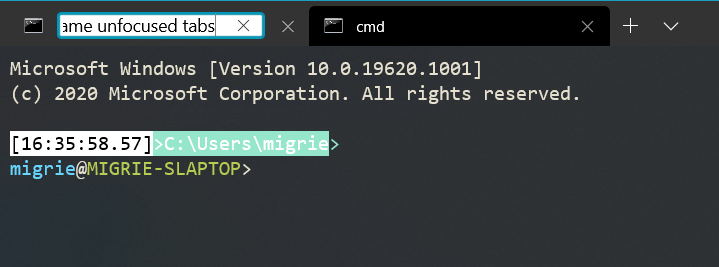
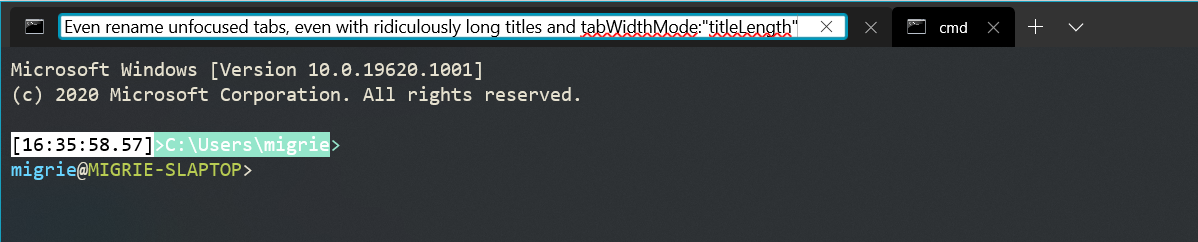
2020-05-28 23:06:17 +02:00
|
|
|
newTabFlyout.Items().Append(renameTabMenuItem);
|
2020-05-04 22:57:12 +02:00
|
|
|
newTabFlyout.Items().Append(menuSeparator);
|
|
|
|
newTabFlyout.Items().Append(closeTabMenuItem);
|
|
|
|
_tabViewItem.ContextFlyout(newTabFlyout);
|
|
|
|
}
|
|
|
|
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
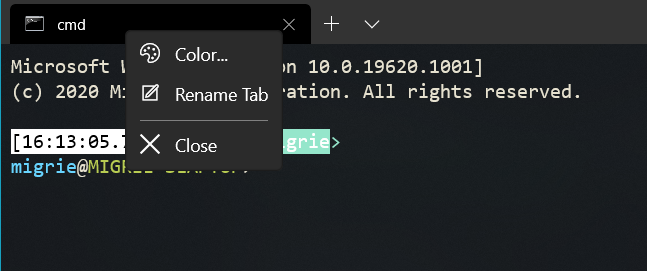
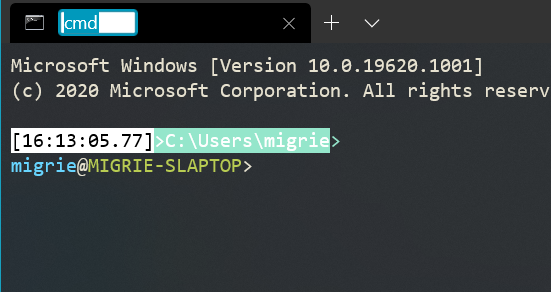
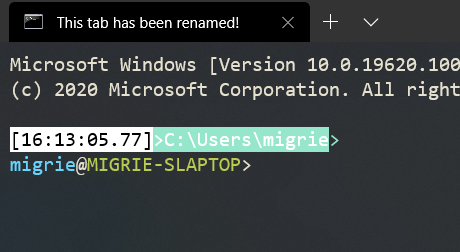
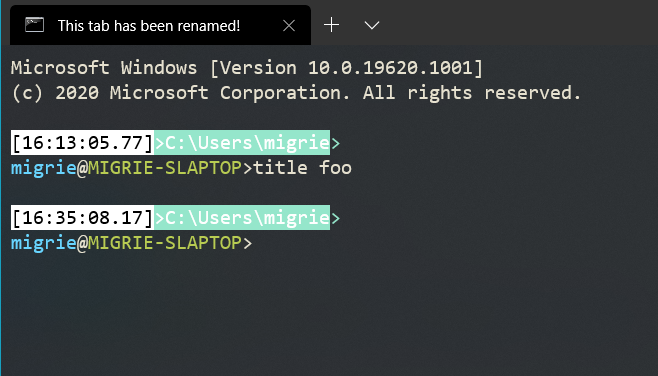
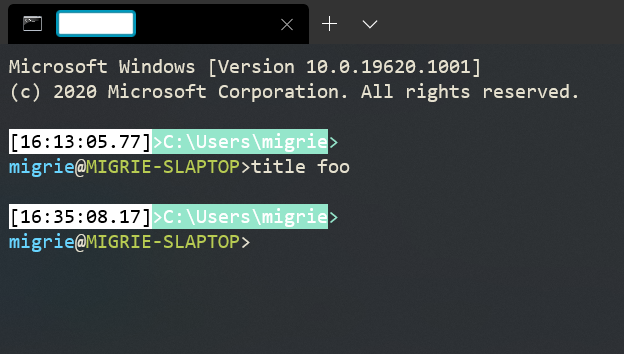
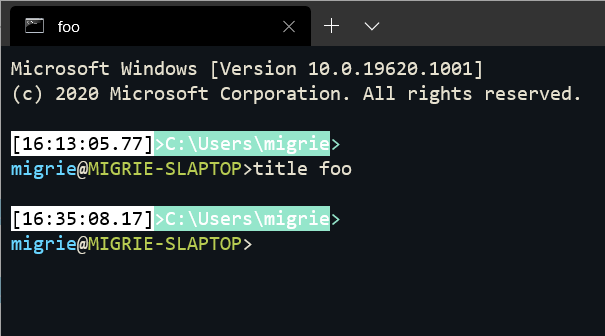
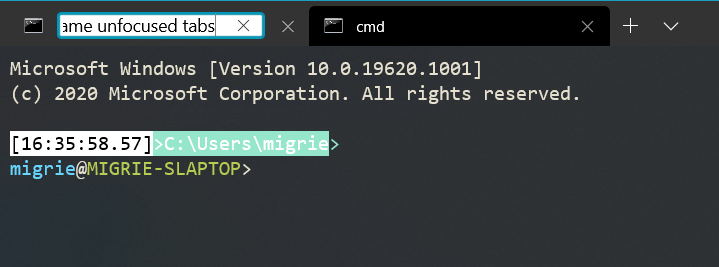
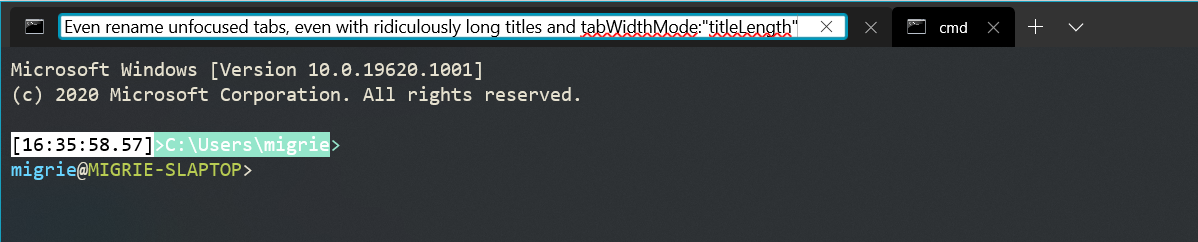
2020-05-28 23:06:17 +02:00
|
|
|
// Method Description:
|
|
|
|
// - This will update the contents of our TabViewItem for our current state.
|
|
|
|
// - If we're not in a rename, we'll set the Header of the TabViewItem to
|
|
|
|
// simply our current tab text (either the runtime tab text or the
|
|
|
|
// active terminal's text).
|
|
|
|
// - If we're in a rename, then we'll set the Header to a TextBox with the
|
|
|
|
// current tab text. The user can then use that TextBox to set a string
|
|
|
|
// to use as an override for the tab's text.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_UpdateTabHeader()
|
|
|
|
{
|
|
|
|
winrt::hstring tabText{ GetActiveTitle() };
|
|
|
|
|
|
|
|
if (!_inRename)
|
|
|
|
{
|
|
|
|
// If we're not currently in the process of renaming the tab, then just set the tab's text to whatever our active title is.
|
|
|
|
_tabViewItem.Header(winrt::box_value(tabText));
|
|
|
|
}
|
|
|
|
else
|
|
|
|
{
|
|
|
|
_ConstructTabRenameBox(tabText);
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
// - Create a new TextBox to use as the control for renaming the tab text.
|
|
|
|
// If the text box is already created, then this will do nothing, and
|
|
|
|
// leave the current box unmodified.
|
|
|
|
// Arguments:
|
|
|
|
// - tabText: This should be the text to initialize the rename text box with.
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_ConstructTabRenameBox(const winrt::hstring& tabText)
|
|
|
|
{
|
|
|
|
if (_tabViewItem.Header().try_as<Controls::TextBox>())
|
|
|
|
{
|
|
|
|
return;
|
|
|
|
}
|
|
|
|
|
|
|
|
Controls::TextBox tabTextBox;
|
|
|
|
tabTextBox.Text(tabText);
|
|
|
|
|
|
|
|
// The TextBox has a MinHeight already set by default, which is
|
|
|
|
// larger than we want. Get rid of it.
|
|
|
|
tabTextBox.MinHeight(0);
|
|
|
|
// Also get rid of the internal padding on the text box, between the
|
|
|
|
// border and the text content, on the top and bottom. This will
|
|
|
|
// help the box fit within the bounds of the tab.
|
|
|
|
Thickness internalPadding = ThicknessHelper::FromLengths(4, 0, 4, 0);
|
|
|
|
tabTextBox.Padding(internalPadding);
|
|
|
|
|
|
|
|
// Make the margin (0, -8, 0, -8), to counteract the padding that
|
|
|
|
// the TabViewItem has.
|
|
|
|
//
|
|
|
|
// This is maybe a bit fragile, as the actual value might not be exactly
|
|
|
|
// (0, 8, 0, 8), but using TabViewItemHeaderPadding to look up the real
|
|
|
|
// value at runtime didn't work. So this is good enough for now.
|
|
|
|
Thickness negativeMargins = ThicknessHelper::FromLengths(0, -8, 0, -8);
|
|
|
|
tabTextBox.Margin(negativeMargins);
|
|
|
|
|
|
|
|
// Set up some event handlers on the text box. We need three of them:
|
|
|
|
// * A LostFocus event, so when the TextBox loses focus, we'll
|
|
|
|
// remove it and return to just the text on the tab.
|
|
|
|
// * A KeyUp event, to be able to submit the tab text on Enter or
|
|
|
|
// dismiss the text box on Escape
|
|
|
|
// * A LayoutUpdated event, so that we can auto-focus the text box
|
|
|
|
// when it's added to the tree.
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
|
|
|
|
// When the text box loses focus, update the tab title of our tab.
|
|
|
|
// - If there are any contents in the box, we'll use that value as
|
|
|
|
// the new "runtime text", which will override any text set by the
|
|
|
|
// application.
|
|
|
|
// - If the text box is empty, we'll reset the "runtime text", and
|
|
|
|
// return to using the active terminal's title.
|
|
|
|
tabTextBox.LostFocus([weakThis](const IInspectable& sender, auto&&) {
|
|
|
|
auto tab{ weakThis.get() };
|
|
|
|
auto textBox{ sender.try_as<Controls::TextBox>() };
|
|
|
|
if (tab && textBox)
|
|
|
|
{
|
|
|
|
tab->_runtimeTabText = textBox.Text();
|
|
|
|
tab->_inRename = false;
|
|
|
|
tab->_UpdateTitle();
|
|
|
|
}
|
|
|
|
});
|
|
|
|
|
|
|
|
// NOTE: (Preview)KeyDown does not work here. If you use that, we'll
|
|
|
|
// remove the TextBox from the UI tree, then the following KeyUp
|
|
|
|
// will bubble to the NewTabButton, which we don't want to have
|
|
|
|
// happen.
|
|
|
|
tabTextBox.KeyUp([weakThis](const IInspectable& sender, Input::KeyRoutedEventArgs const& e) {
|
|
|
|
auto tab{ weakThis.get() };
|
|
|
|
auto textBox{ sender.try_as<Controls::TextBox>() };
|
|
|
|
if (tab && textBox)
|
|
|
|
{
|
|
|
|
switch (e.OriginalKey())
|
|
|
|
{
|
|
|
|
case VirtualKey::Enter:
|
|
|
|
tab->_runtimeTabText = textBox.Text();
|
|
|
|
[[fallthrough]];
|
|
|
|
case VirtualKey::Escape:
|
|
|
|
e.Handled(true);
|
|
|
|
textBox.Text(tab->_runtimeTabText);
|
|
|
|
tab->_inRename = false;
|
|
|
|
tab->_UpdateTitle();
|
|
|
|
break;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
});
|
|
|
|
|
|
|
|
// As soon as the text box is added to the UI tree, focus it. We can't focus it till it's in the tree.
|
|
|
|
_tabRenameBoxLayoutUpdatedRevoker = tabTextBox.LayoutUpdated(winrt::auto_revoke, [this](auto&&, auto&&) {
|
|
|
|
// Curiously, the sender for this event is null, so we have to
|
|
|
|
// get the TextBox from the Tab's Header().
|
|
|
|
auto textBox{ _tabViewItem.Header().try_as<Controls::TextBox>() };
|
|
|
|
if (textBox)
|
|
|
|
{
|
|
|
|
textBox.SelectAll();
|
|
|
|
textBox.Focus(FocusState::Programmatic);
|
|
|
|
}
|
|
|
|
// Only let this succeed once.
|
|
|
|
_tabRenameBoxLayoutUpdatedRevoker.revoke();
|
|
|
|
});
|
|
|
|
|
|
|
|
_tabViewItem.Header(tabTextBox);
|
|
|
|
}
|
|
|
|
|
2020-05-04 22:57:12 +02:00
|
|
|
// Method Description:
|
|
|
|
// Returns the tab color, if any
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - The tab's color, if any
|
|
|
|
std::optional<winrt::Windows::UI::Color> Tab::GetTabColor()
|
|
|
|
{
|
|
|
|
return _tabColor;
|
|
|
|
}
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
// - Sets the tab background color to the color chosen by the user
|
|
|
|
// - Sets the tab foreground color depending on the luminance of
|
|
|
|
// the background color
|
|
|
|
// Arguments:
|
|
|
|
// - color: the shiny color the user picked for their tab
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
Add `setTabColor` and `openTabColorPicker` actions (#6567)
## Summary of the Pull Request
Adds a pair of `ShortcutAction`s for setting the tab color.
* `setTabColor`: This changes the color of the current tab to the provided color, or can be used to clear the color.
* `openTabColorPicker`: This keybinding immediately activates the tab color picker for the currently focused tab.
## References
## PR Checklist
* [x] scratches my own itch
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/69
## Detailed Description of the Pull Request / Additional comments
## Validation Steps Performed
* hey look there are tests
* Tested with the following:
```json
// { "command": "setTabColor", "keys": [ "alt+c" ] },
{ "keys": "ctrl+alt+c", "command": { "action": "setTabColor", "color": "#123456" } },
{ "keys": "alt+shift+c", "command": { "action": "setTabColor", "color": null} },
{ "keys": "alt+c", "command": "openTabColorPicker" },
```
2020-06-25 15:06:21 +02:00
|
|
|
void Tab::SetTabColor(const winrt::Windows::UI::Color& color)
|
2020-05-04 22:57:12 +02:00
|
|
|
{
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
|
|
|
|
_tabViewItem.Dispatcher().RunAsync(CoreDispatcherPriority::Normal, [weakThis, color]() {
|
|
|
|
auto ptrTab = weakThis.get();
|
|
|
|
if (!ptrTab)
|
|
|
|
return;
|
|
|
|
|
|
|
|
auto tab{ ptrTab };
|
|
|
|
Media::SolidColorBrush selectedTabBrush{};
|
|
|
|
Media::SolidColorBrush deselectedTabBrush{};
|
|
|
|
Media::SolidColorBrush fontBrush{};
|
|
|
|
Media::SolidColorBrush hoverTabBrush{};
|
|
|
|
// calculate the luminance of the current color and select a font
|
|
|
|
// color based on that
|
|
|
|
// see https://www.w3.org/TR/WCAG20/#relativeluminancedef
|
|
|
|
if (TerminalApp::ColorHelper::IsBrightColor(color))
|
|
|
|
{
|
|
|
|
fontBrush.Color(winrt::Windows::UI::Colors::Black());
|
|
|
|
}
|
|
|
|
else
|
|
|
|
{
|
|
|
|
fontBrush.Color(winrt::Windows::UI::Colors::White());
|
|
|
|
}
|
|
|
|
|
|
|
|
hoverTabBrush.Color(TerminalApp::ColorHelper::GetAccentColor(color));
|
|
|
|
selectedTabBrush.Color(color);
|
|
|
|
|
|
|
|
// currently if a tab has a custom color, a deselected state is
|
|
|
|
// signified by using the same color with a bit ot transparency
|
|
|
|
auto deselectedTabColor = color;
|
|
|
|
deselectedTabColor.A = 64;
|
|
|
|
deselectedTabBrush.Color(deselectedTabColor);
|
|
|
|
|
|
|
|
// currently if a tab has a custom color, a deselected state is
|
|
|
|
// signified by using the same color with a bit ot transparency
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderBackgroundSelected"), selectedTabBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderBackground"), deselectedTabBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderBackgroundPointerOver"), hoverTabBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderBackgroundPressed"), selectedTabBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderForeground"), fontBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderForegroundSelected"), fontBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderForegroundPointerOver"), fontBrush);
|
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewItemHeaderForegroundPressed"), fontBrush);
|
2020-06-05 21:12:28 +02:00
|
|
|
tab->_tabViewItem.Resources().Insert(winrt::box_value(L"TabViewButtonForegroundActiveTab"), fontBrush);
|
2020-05-04 22:57:12 +02:00
|
|
|
|
|
|
|
tab->_RefreshVisualState();
|
|
|
|
|
|
|
|
tab->_tabColor.emplace(color);
|
|
|
|
tab->_colorSelected(color);
|
|
|
|
});
|
|
|
|
}
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
// Clear the custom color of the tab, if any
|
|
|
|
// the background color
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
Add `setTabColor` and `openTabColorPicker` actions (#6567)
## Summary of the Pull Request
Adds a pair of `ShortcutAction`s for setting the tab color.
* `setTabColor`: This changes the color of the current tab to the provided color, or can be used to clear the color.
* `openTabColorPicker`: This keybinding immediately activates the tab color picker for the currently focused tab.
## References
## PR Checklist
* [x] scratches my own itch
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/69
## Detailed Description of the Pull Request / Additional comments
## Validation Steps Performed
* hey look there are tests
* Tested with the following:
```json
// { "command": "setTabColor", "keys": [ "alt+c" ] },
{ "keys": "ctrl+alt+c", "command": { "action": "setTabColor", "color": "#123456" } },
{ "keys": "alt+shift+c", "command": { "action": "setTabColor", "color": null} },
{ "keys": "alt+c", "command": "openTabColorPicker" },
```
2020-06-25 15:06:21 +02:00
|
|
|
void Tab::ResetTabColor()
|
2020-05-04 22:57:12 +02:00
|
|
|
{
|
|
|
|
auto weakThis{ get_weak() };
|
|
|
|
|
|
|
|
_tabViewItem.Dispatcher().RunAsync(CoreDispatcherPriority::Normal, [weakThis]() {
|
|
|
|
auto ptrTab = weakThis.get();
|
|
|
|
if (!ptrTab)
|
|
|
|
return;
|
|
|
|
|
|
|
|
auto tab{ ptrTab };
|
|
|
|
winrt::hstring keys[] = {
|
|
|
|
L"TabViewItemHeaderBackground",
|
|
|
|
L"TabViewItemHeaderBackgroundSelected",
|
|
|
|
L"TabViewItemHeaderBackgroundPointerOver",
|
|
|
|
L"TabViewItemHeaderForeground",
|
|
|
|
L"TabViewItemHeaderForegroundSelected",
|
|
|
|
L"TabViewItemHeaderForegroundPointerOver",
|
|
|
|
L"TabViewItemHeaderBackgroundPressed",
|
2020-06-05 21:12:28 +02:00
|
|
|
L"TabViewItemHeaderForegroundPressed",
|
|
|
|
L"TabViewButtonForegroundActiveTab"
|
2020-05-04 22:57:12 +02:00
|
|
|
};
|
|
|
|
|
|
|
|
// simply clear any of the colors in the tab's dict
|
|
|
|
for (auto keyString : keys)
|
|
|
|
{
|
|
|
|
auto key = winrt::box_value(keyString);
|
|
|
|
if (tab->_tabViewItem.Resources().HasKey(key))
|
|
|
|
{
|
|
|
|
tab->_tabViewItem.Resources().Remove(key);
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
tab->_RefreshVisualState();
|
|
|
|
tab->_tabColor.reset();
|
|
|
|
tab->_colorCleared();
|
|
|
|
});
|
|
|
|
}
|
|
|
|
|
Add `setTabColor` and `openTabColorPicker` actions (#6567)
## Summary of the Pull Request
Adds a pair of `ShortcutAction`s for setting the tab color.
* `setTabColor`: This changes the color of the current tab to the provided color, or can be used to clear the color.
* `openTabColorPicker`: This keybinding immediately activates the tab color picker for the currently focused tab.
## References
## PR Checklist
* [x] scratches my own itch
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/69
## Detailed Description of the Pull Request / Additional comments
## Validation Steps Performed
* hey look there are tests
* Tested with the following:
```json
// { "command": "setTabColor", "keys": [ "alt+c" ] },
{ "keys": "ctrl+alt+c", "command": { "action": "setTabColor", "color": "#123456" } },
{ "keys": "alt+shift+c", "command": { "action": "setTabColor", "color": null} },
{ "keys": "alt+c", "command": "openTabColorPicker" },
```
2020-06-25 15:06:21 +02:00
|
|
|
// Method Description:
|
|
|
|
// - Display the tab color picker at the location of the TabViewItem for this tab.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::ActivateColorPicker()
|
|
|
|
{
|
|
|
|
_tabColorPickup.ShowAt(_tabViewItem);
|
|
|
|
}
|
|
|
|
|
2020-05-04 22:57:12 +02:00
|
|
|
// Method Description:
|
|
|
|
// Toggles the visual state of the tab view item,
|
|
|
|
// so that changes to the tab color are reflected immediately
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - <none>
|
|
|
|
void Tab::_RefreshVisualState()
|
|
|
|
{
|
|
|
|
if (_focused)
|
|
|
|
{
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
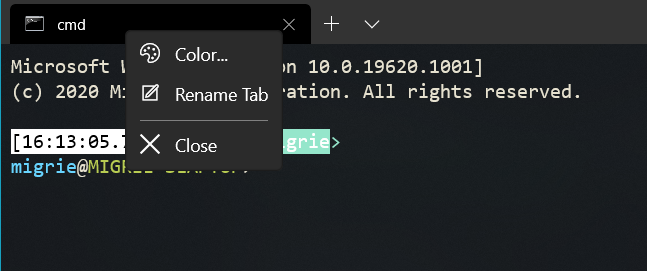
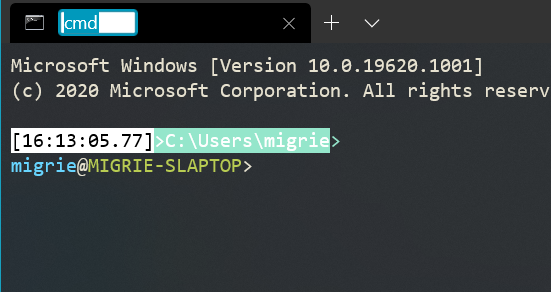
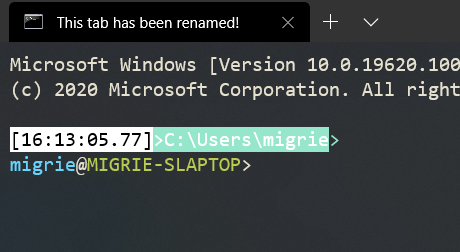
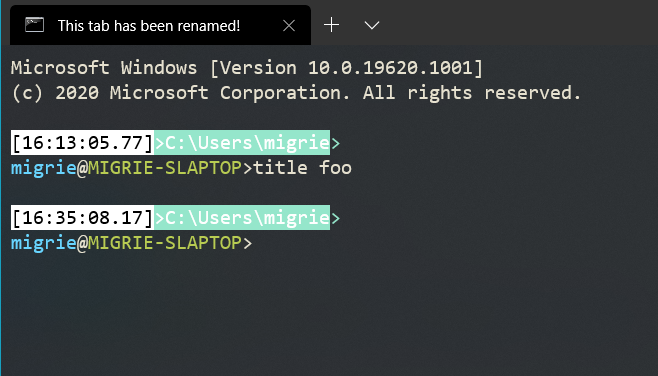
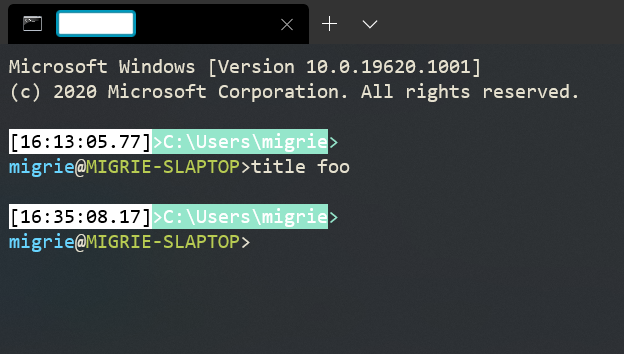
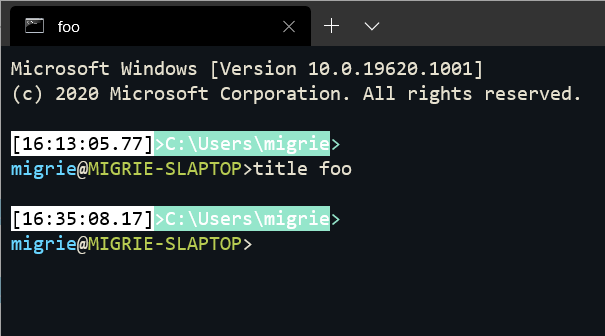
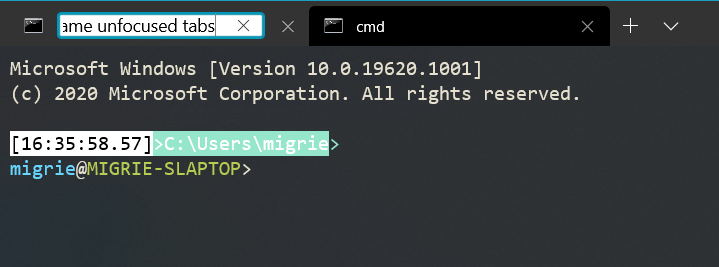
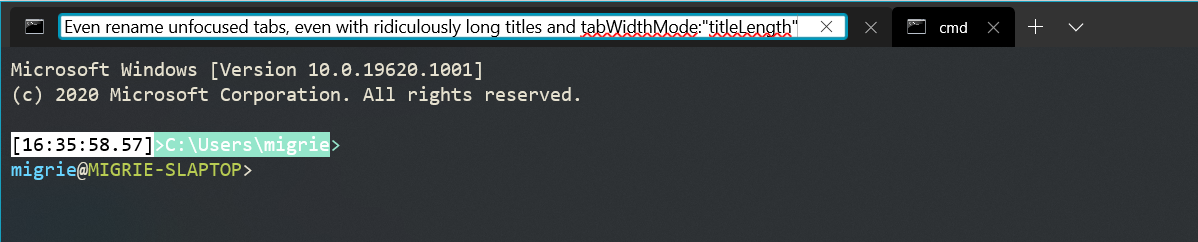
2020-05-28 23:06:17 +02:00
|
|
|
VisualStateManager::GoToState(_tabViewItem, L"Normal", true);
|
|
|
|
VisualStateManager::GoToState(_tabViewItem, L"Selected", true);
|
2020-05-04 22:57:12 +02:00
|
|
|
}
|
|
|
|
else
|
|
|
|
{
|
Enable tab renaming at runtime from the UI (#5775)
## Summary of the Pull Request
Adds support for setting, from the UI, a runtime override for the tab title text. The user can use this to effectively "rename" a tab.
If set, the tab will _always_ use the runtime override string. If the user has multiple panes with different titles in a pane, then the tab's override text will be used _regardless_ of which pane was focused when the tab was renamed.
The override text can be removed by just deleting the entire contents of the box. Then, the tab will revert to using the terminal's usual title.
## References
* Wouldn't be possible without the context menu from #3789
* Focus doesn't return to the active terminal after hitting <kbd>enter</kbd>/<kbd>esc</kbd>, but that's tracked by #5750
## PR Checklist
* [x] Closes #1079
* [x] I work here
* [ ] Tests added/passed
* [ ] Requires documentation to be updated
## TODO
* [x] `Tab::SetTabText` might be able to be greatly simplified/removed?
* [x] I'm _pretty sure_ if they set an override title, we won't bubble that up to set the window title.
* [x] I'm unsure how this behaves when the terminal's title changes _while_ the TextBox is visible. I don't think it should change the current contents of the box, but it might currently.
* [ ] **for discussion**: If the user doesn't actually change the text of the tab, then we probably shouldn't set the override text, right?
- EX: if they open the box and the text is "cmd", and immediately hit <kbd>enter</kbd>, then run `title foo`, should the text change to "foo" or stay "cmd"?
## Detailed Description of the Pull Request / Additional comments
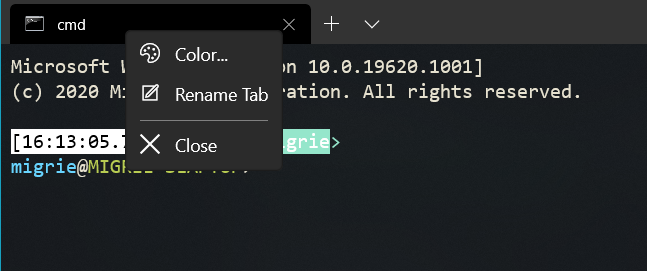
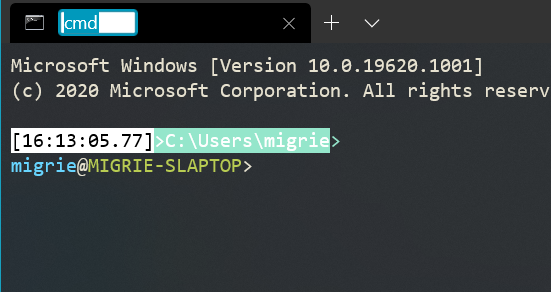
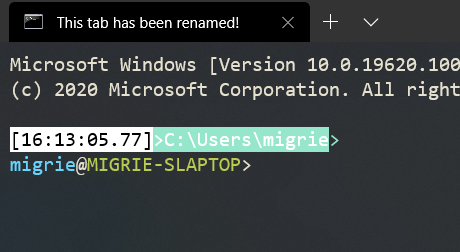
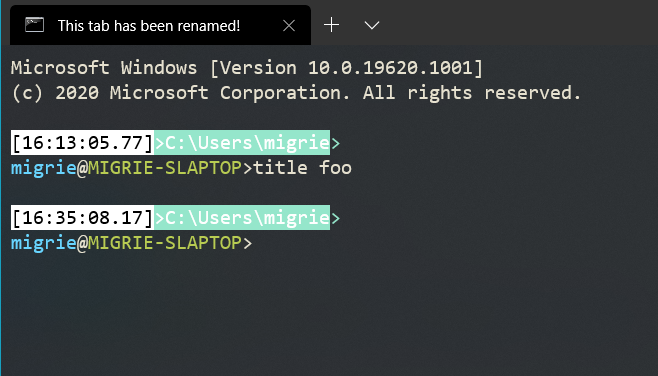
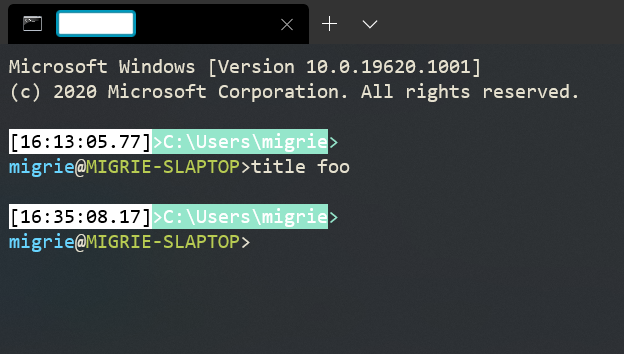
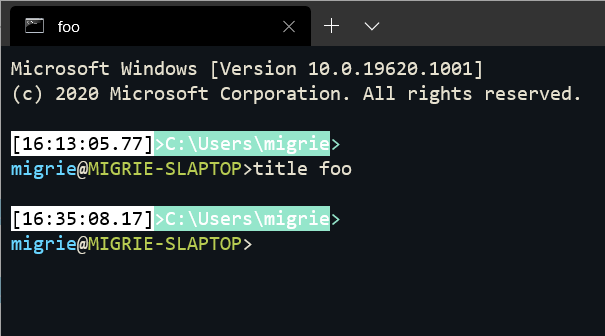
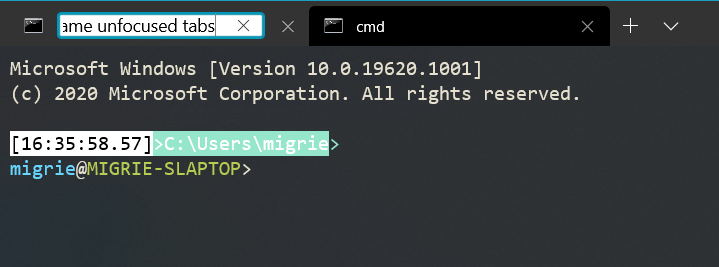
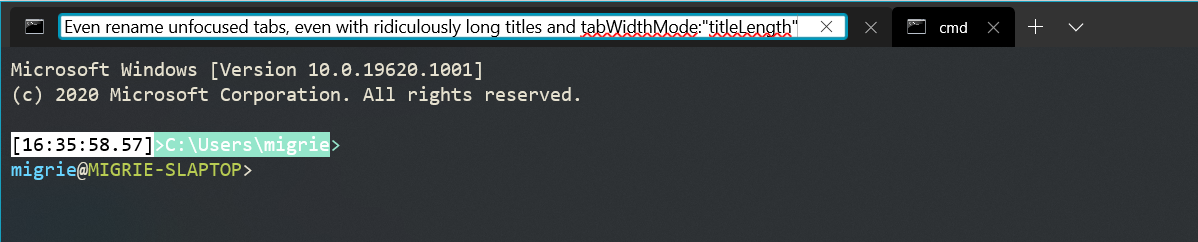
2020-05-28 23:06:17 +02:00
|
|
|
VisualStateManager::GoToState(_tabViewItem, L"Selected", true);
|
|
|
|
VisualStateManager::GoToState(_tabViewItem, L"Normal", true);
|
2020-05-04 22:57:12 +02:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
Process actions sync. on startup; don't dupe nonexistent profile (#5090)
This PR has evolved to encapsulate two related fixes that I can't really
untie anymore.
#2455 - Duplicating a tab that doesn't exist anymore
This was the bug I was originally fixing in #4429.
When the user tries to `duplicateTab` with a profile that doesn't exist
anymore (like might happen after a settings reload), don't crash.
As I was going about adding tests for this, got blocked by the fact that
the Terminal couldn't open _any_ panes while the `TerminalPage` was size
0x0. This had two theoretical solutions:
* Fake the `TerminalPage` into thinking it had a real size in the test -
probably possible, though I'm unsure how it would work in practice.
* Change `Pane`s to not require an `ActualWidth`, `ActualHeight` on
initialization.
Fortuately, the second option was something else that was already on my
backlog of bugs.
#4618 - `wt` command-line can't consistently parse more than one arg
Presently, the Terminal just arbitrarily dispatches a bunch of handlers
to try and handle all the commands provided on the commandline. That's
lead to a bunch of reports that not all the commands will always get
executed, nor will they all get executed in the same order.
This PR also changes the `TerminalPage` to be able to dispatch all the
commands sequentially, all at once in the startup. No longer will there
be a hot second where the commands seem to execute themselves in from of
the user - they'll all happen behind the scenes on startup.
This involved a couple other changes areound the `TerminalPage`
* I had to make sure that panes could be opened at a 0x0 size. Now they
use a star sizing based off the percentage of the parent they're
supposed to consume, so that when the parent _does_ get laid out,
they'll take the appropriate size of that parent.
* I had to do some math ahead of time to try and calculate what a
`SplitState::Automatic` would be evaluated as, despite the fact that
we don't actually know how big the pane will be.
* I had to ensure that `focus-tab` commands appropriately mark a single
tab as focused while we're in startup, without roundtripping to the
Dispatcher thread and back
## References
#4429 - the original PR for #2455
#5047 - a follow-up task from discussion in #4429
#4953 - a PR for making panes use star sizing, which was immensly
helpful for this PR.
## Detailed Description of the Pull Request / Additional comments
`CascadiaSettings::BuildSettings` can throw if the GUID doesn't exist.
This wraps those calls up with a try/catch.
It also adds a couple tests - a few `SettingsTests` for try/catching
this state. It also adds a XAML-y test in `TabTests` that creates a
`TerminalPage` and then performs som UI-like actions on it. This test
required a minor change to how we generate the new tab dropdown - in the
tests, `Application::Current()` is _not_ a `TerminalApp::App`, so it
doesn't have a `Logic()` to query. So wrap that in a try/catch as well.
While working on these tests, I found that we'd crash pretty agressively
for mysterious reasons if the TestHostApp became focused while the test
was running. This was due to a call in
`TSFInputControl::NotifyFocusEnter` that would callback to
`TSFInputControl::_layoutRequested`, which would crash on setting the
`MaxSize` of the canvas to a negative value. This PR includes a hotfix
for that bug as well.
## Validation Steps Performed
* Manual testing with a _lot_ of commands in a commandline
* run the tests
* Team tested in selfhost
Closes #2455
Closes #4618
2020-03-26 01:03:32 +01:00
|
|
|
// - Get the total number of leaf panes in this tab. This will be the number
|
|
|
|
// of actual controls hosted by this tab.
|
|
|
|
// Arguments:
|
|
|
|
// - <none>
|
|
|
|
// Return Value:
|
|
|
|
// - The total number of leaf panes hosted by this tab.
|
|
|
|
int Tab::_GetLeafPaneCount() const noexcept
|
|
|
|
{
|
|
|
|
return _rootPane->GetLeafPaneCount();
|
|
|
|
}
|
|
|
|
|
|
|
|
// Method Description:
|
|
|
|
// - This is a helper to determine which direction an "Automatic" split should
|
|
|
|
// happen in for the active pane of this tab, but without using the ActualWidth() and
|
|
|
|
// ActualHeight() methods.
|
|
|
|
// - See Pane::PreCalculateAutoSplit
|
|
|
|
// Arguments:
|
|
|
|
// - availableSpace: The theoretical space that's available for this Tab's content
|
|
|
|
// Return Value:
|
|
|
|
// - The SplitState that we should use for an `Automatic` split given
|
|
|
|
// `availableSpace`
|
|
|
|
SplitState Tab::PreCalculateAutoSplit(winrt::Windows::Foundation::Size availableSpace) const
|
|
|
|
{
|
|
|
|
return _rootPane->PreCalculateAutoSplit(_activePane, availableSpace).value_or(SplitState::Vertical);
|
|
|
|
}
|
|
|
|
|
Add support for running a `wt` commandline in the curent window WITH A KEYBINDING (#6537)
## Summary of the Pull Request
Adds a execute commandline action (`wt`), which lets a user bind a key to a specific `wt` commandline. This commandline will get parsed and run _in the current window_.
## References
* Related to #4472
* Related to #5400 - I need this for the commandline mode of the Command Palette
* Related to #5970
## PR Checklist
* [x] Closes oh, there's not actually an issue for this.
* [x] I work here
* [x] Tests added/passed
* [ ] Requires documentation to be updated - yes it does
## Detailed Description of the Pull Request / Additional comments
One important part of this change concerns how panes are initialized at runtime. We've had some persistent trouble with initializing multiple panes, because they rely on knowing how big they'll actually be, to be able to determine if they can split again.
We previously worked around this by ignoring the size check when we were in "startup", processing an initial commandline. This PR however requires us to be able to know the initial size of a pane at runtime, but before the parents have necessarily been added to the tree, or had their renderer's set up.
This led to the development of `Pane::PreCalculateCanSplit`, which is very highly similar to `Pane::PreCalculateAutoSplit`. This method attempts to figure out how big a pane _will_ take, before the parent has necessarily laid out.
This also involves a small change to `TermControl`, because if its renderer hasn't been set up yet, it'll always think the font is `{0, fontHeight}`, which will let the Terminal keep splitting in the x direction. This change also makes the TermControl set up a renderer to get the real font size when it hasn't yet been initialized.
## Validation Steps Performed
This was what the json blob I was using for testing evolved into
```json
{
"command": {
"action":"wt",
"commandline": "new-tab cmd.exe /k #work 15 ; split-pane cmd.exe /k #work 15 ; split-pane cmd.exe /k media-commandline ; new-tab powershell dev\\symbols.ps1 ; new-tab -p \"Ubuntu\" ; new-tab -p \"haunter.gif\" ; focus-tab -t 0",
},
"keys": ["ctrl+shift+n"]
}
```
I also added some tests.
# TODO
* [x] Creating a `{ "command": "wt" }` action without a commandline will spawn a new `wt.exe` process?
- Probably should just do nothing for the empty string
2020-07-17 23:05:29 +02:00
|
|
|
bool Tab::PreCalculateCanSplit(SplitState splitType, winrt::Windows::Foundation::Size availableSpace) const
|
|
|
|
{
|
|
|
|
return _rootPane->PreCalculateCanSplit(_activePane, splitType, availableSpace).value_or(false);
|
|
|
|
}
|
2020-02-04 22:51:11 +01:00
|
|
|
DEFINE_EVENT(Tab, ActivePaneChanged, _ActivePaneChangedHandlers, winrt::delegate<>);
|
2020-05-04 22:57:12 +02:00
|
|
|
DEFINE_EVENT(Tab, ColorSelected, _colorSelected, winrt::delegate<winrt::Windows::UI::Color>);
|
|
|
|
DEFINE_EVENT(Tab, ColorCleared, _colorCleared, winrt::delegate<>);
|
2019-07-19 02:23:40 +02:00
|
|
|
}
|