2021-03-16 00:15:25 +01:00
|
|
|
/*++
|
|
|
|
Copyright (c) Microsoft Corporation
|
|
|
|
Licensed under the MIT license.
|
|
|
|
|
|
|
|
Module Name:
|
|
|
|
- TerminalSettings.h
|
|
|
|
|
|
|
|
Abstract:
|
|
|
|
- The implementation of the TerminalSettings winrt class. Provides both
|
|
|
|
terminal control settings and terminal core settings.
|
|
|
|
Author(s):
|
|
|
|
- Mike Griese - March 2019
|
|
|
|
|
|
|
|
--*/
|
|
|
|
#pragma once
|
|
|
|
|
|
|
|
#include "TerminalSettings.g.h"
|
2021-04-09 00:46:16 +02:00
|
|
|
#include "TerminalSettingsCreateResult.g.h"
|
2021-03-16 00:15:25 +01:00
|
|
|
#include "IInheritable.h"
|
|
|
|
#include "../inc/cppwinrt_utils.h"
|
|
|
|
#include <DefaultSettings.h>
|
|
|
|
#include <conattrs.hpp>
|
|
|
|
|
2021-07-23 01:15:44 +02:00
|
|
|
using IFontAxesMap = winrt::Windows::Foundation::Collections::IMap<winrt::hstring, float>;
|
|
|
|
using IFontFeatureMap = winrt::Windows::Foundation::Collections::IMap<winrt::hstring, uint32_t>;
|
|
|
|
|
2021-03-16 00:15:25 +01:00
|
|
|
// fwdecl unittest classes
|
|
|
|
namespace SettingsModelLocalTests
|
|
|
|
{
|
|
|
|
class TerminalSettingsTests;
|
|
|
|
}
|
|
|
|
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Model::implementation
|
|
|
|
{
|
2021-04-09 00:46:16 +02:00
|
|
|
struct TerminalSettingsCreateResult :
|
|
|
|
public TerminalSettingsCreateResultT<TerminalSettingsCreateResult>
|
|
|
|
{
|
|
|
|
public:
|
|
|
|
TerminalSettingsCreateResult(Model::TerminalSettings defaultSettings, Model::TerminalSettings unfocusedSettings) :
|
|
|
|
_defaultSettings(defaultSettings),
|
|
|
|
_unfocusedSettings(unfocusedSettings) {}
|
|
|
|
|
2021-04-21 22:35:06 +02:00
|
|
|
TerminalSettingsCreateResult(Model::TerminalSettings defaultSettings) :
|
|
|
|
_defaultSettings(defaultSettings),
|
|
|
|
_unfocusedSettings(nullptr) {}
|
|
|
|
|
2021-04-09 00:46:16 +02:00
|
|
|
Model::TerminalSettings DefaultSettings() { return _defaultSettings; };
|
|
|
|
Model::TerminalSettings UnfocusedSettings() { return _unfocusedSettings; };
|
|
|
|
|
|
|
|
private:
|
|
|
|
Model::TerminalSettings _defaultSettings;
|
|
|
|
Model::TerminalSettings _unfocusedSettings;
|
|
|
|
};
|
|
|
|
|
2021-03-16 00:15:25 +01:00
|
|
|
struct TerminalSettings : TerminalSettingsT<TerminalSettings>, IInheritable<TerminalSettings>
|
|
|
|
{
|
|
|
|
TerminalSettings() = default;
|
|
|
|
|
Reintroduce the Defaults page and the Reset buttons (#10588)
This pull request brings back the "Base Layer" page, now renamed to
"Defaults", and the "Reset to inherited value" buttons. The scope of
inheritance for which buttons will display has been widened.
The button will be visible in the following cases:
The user has set a setting for the current profile, and it overrides...
1. ... something in profiles.defaults.
2. ... something in a Fragment Extension profile.
3. ... something from a Dynamic Profile Generator.
4. ... something from the compiled-in defaults.
Compared to the original implementation of reset arrows, cases (1), (3)
and (4) are new. Rationale:
(1) The user can see a setting on the Defaults page, and they need a way
to reset back to it.
(3) Dynamic profiles are not meaningfully different from fragments, and
users may need a way to reset back to the default value generated
for WSL or PowerShell.
(4) The user can see a setting on the Defaults page, **BUT** they are
not the one who created it. They *still* need a way to get back to
it.
To support this, I've introduced another origin tag, "User", and renamed
"Custom" to "None". Due to the way origin/override detection works¹, we
cannot otherwise disambiguate between settings that came from the user
and settings that came from the compiled-in defaults.
Changes were required in TerminalSettings such that we could construct a
settings object with a profile that does not have a GUID. In making this
change, I fixed a bit of silliness where we took a profile, extracted
its guid, and used that guid to look up the same profile object. Oops.
I also fixed the PropertyChanged notifier to include the
XxxOverrideSource property.
The presence of the page and the reset arrows is restricted to
Preview- or Dev-branded builds. Stable builds will retain their current
behavior.
¹ `XxxOverrideSource` returns the profile *above* the current profile
that holds a value for setting `Xxx`. When the value is the
compiled-in value, `XxxOverrideSource` will be `null`. Since it's
supposed to be the profile above the current profile, it will also be
`null` if the profile contains a setting at this layer.
In short, `null` means "user specified" *or* "compiled in". Oops.
Fixes #10430
Validation
----------
* [x] Tested Release build to make sure it's mostly arrow-free (apart from fragments)
2021-07-10 00:03:41 +02:00
|
|
|
static Model::TerminalSettingsCreateResult CreateWithProfile(const Model::CascadiaSettings& appSettings,
|
|
|
|
const Model::Profile& profile,
|
|
|
|
const Control::IKeyBindings& keybindings);
|
|
|
|
|
2021-04-09 00:46:16 +02:00
|
|
|
static Model::TerminalSettingsCreateResult CreateWithNewTerminalArgs(const Model::CascadiaSettings& appSettings,
|
|
|
|
const Model::NewTerminalArgs& newTerminalArgs,
|
|
|
|
const Control::IKeyBindings& keybindings);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
2021-04-09 00:46:16 +02:00
|
|
|
static Model::TerminalSettingsCreateResult CreateWithParent(const Model::TerminalSettingsCreateResult& parent);
|
2021-04-21 22:35:06 +02:00
|
|
|
|
|
|
|
Model::TerminalSettings GetParent();
|
|
|
|
|
2021-03-16 00:15:25 +01:00
|
|
|
void SetParent(const Model::TerminalSettings& parent);
|
|
|
|
|
|
|
|
void ApplyColorScheme(const Model::ColorScheme& scheme);
|
|
|
|
|
|
|
|
// --------------------------- Core Settings ---------------------------
|
|
|
|
// All of these settings are defined in ICoreSettings.
|
|
|
|
|
|
|
|
// GetColorTableEntry needs to be implemented manually, to get a
|
|
|
|
// particular value from the array.
|
Introduce MS.Term.Core.Color to replace W.U.Color for Core/Control/TSM (#9658)
This pull request introduces Microsoft.Terminal.Core.Color as an
alternative to both Windows.UI.Color and uint32_t/COLORREF in the
TerminalCore, ...Control, ...SettingsModel and ...SettingsEditor layers.
M.T.C.Color is trivially convertible to/from til::color and therefore
to/from COLORREF, W.U.Color, and any other color representation we might
need².
I've replaced almost every use of W.U.Color and uint32_t-as-color in the
above layers, with minor exception¹.
The need for this work is twofold.
First: We cannot bear a dependency from TerminalCore (which should,
on paper, be Windows 7 compatible) on Windows.UI or any other WinRT
namespace.
This work removes one big dependency on Windows.UI, but it does not go
all the way.
Second: TerminalCore chose to communicate mostly in packed uint32s
(COLORREF), which was inherently lossy and dangerous.
¹ The UI layers (TerminalControl, TerminalApp) still use
Windows.UI.Color as they are intimately connected to the UWP XAML UI.
² In the future, we might even be able to *use* the alpha channel...
## PR Checklist
* [x] I ran into the need for this when I introduced cursor inversion
* [X] Fixes a longstanding itch
## Validation Steps Performed
Built and ran all tests for the impacted layers, even the local ones!
2021-03-30 22:15:49 +02:00
|
|
|
Microsoft::Terminal::Core::Color GetColorTableEntry(int32_t index) noexcept;
|
|
|
|
void ColorTable(std::array<Microsoft::Terminal::Core::Color, 16> colors);
|
|
|
|
std::array<Microsoft::Terminal::Core::Color, 16> ColorTable();
|
2021-03-16 00:15:25 +01:00
|
|
|
|
Introduce MS.Term.Core.Color to replace W.U.Color for Core/Control/TSM (#9658)
This pull request introduces Microsoft.Terminal.Core.Color as an
alternative to both Windows.UI.Color and uint32_t/COLORREF in the
TerminalCore, ...Control, ...SettingsModel and ...SettingsEditor layers.
M.T.C.Color is trivially convertible to/from til::color and therefore
to/from COLORREF, W.U.Color, and any other color representation we might
need².
I've replaced almost every use of W.U.Color and uint32_t-as-color in the
above layers, with minor exception¹.
The need for this work is twofold.
First: We cannot bear a dependency from TerminalCore (which should,
on paper, be Windows 7 compatible) on Windows.UI or any other WinRT
namespace.
This work removes one big dependency on Windows.UI, but it does not go
all the way.
Second: TerminalCore chose to communicate mostly in packed uint32s
(COLORREF), which was inherently lossy and dangerous.
¹ The UI layers (TerminalControl, TerminalApp) still use
Windows.UI.Color as they are intimately connected to the UWP XAML UI.
² In the future, we might even be able to *use* the alpha channel...
## PR Checklist
* [x] I ran into the need for this when I introduced cursor inversion
* [X] Fixes a longstanding itch
## Validation Steps Performed
Built and ran all tests for the impacted layers, even the local ones!
2021-03-30 22:15:49 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, til::color, DefaultForeground, DEFAULT_FOREGROUND);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, til::color, DefaultBackground, DEFAULT_BACKGROUND);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, til::color, SelectionBackground, DEFAULT_FOREGROUND);
|
2021-03-16 00:15:25 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, int32_t, HistorySize, DEFAULT_HISTORY_SIZE);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, int32_t, InitialRows, 30);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, int32_t, InitialCols, 80);
|
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, SnapOnInput, true);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, AltGrAliasing, true);
|
Introduce MS.Term.Core.Color to replace W.U.Color for Core/Control/TSM (#9658)
This pull request introduces Microsoft.Terminal.Core.Color as an
alternative to both Windows.UI.Color and uint32_t/COLORREF in the
TerminalCore, ...Control, ...SettingsModel and ...SettingsEditor layers.
M.T.C.Color is trivially convertible to/from til::color and therefore
to/from COLORREF, W.U.Color, and any other color representation we might
need².
I've replaced almost every use of W.U.Color and uint32_t-as-color in the
above layers, with minor exception¹.
The need for this work is twofold.
First: We cannot bear a dependency from TerminalCore (which should,
on paper, be Windows 7 compatible) on Windows.UI or any other WinRT
namespace.
This work removes one big dependency on Windows.UI, but it does not go
all the way.
Second: TerminalCore chose to communicate mostly in packed uint32s
(COLORREF), which was inherently lossy and dangerous.
¹ The UI layers (TerminalControl, TerminalApp) still use
Windows.UI.Color as they are intimately connected to the UWP XAML UI.
² In the future, we might even be able to *use* the alpha channel...
## PR Checklist
* [x] I ran into the need for this when I introduced cursor inversion
* [X] Fixes a longstanding itch
## Validation Steps Performed
Built and ran all tests for the impacted layers, even the local ones!
2021-03-30 22:15:49 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, til::color, CursorColor, DEFAULT_CURSOR_COLOR);
|
2021-03-17 21:47:24 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Microsoft::Terminal::Core::CursorStyle, CursorShape, Core::CursorStyle::Vintage);
|
2021-03-16 00:15:25 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, uint32_t, CursorHeight, DEFAULT_CURSOR_HEIGHT);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, WordDelimiters, DEFAULT_WORD_DELIMITERS);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, CopyOnSelect, false);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, InputServiceWarning, true);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, FocusFollowMouse, false);
|
2021-04-24 00:36:51 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, TrimBlockSelection, false);
|
2021-05-17 06:20:09 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, DetectURLs, true);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
Introduce MS.Term.Core.Color to replace W.U.Color for Core/Control/TSM (#9658)
This pull request introduces Microsoft.Terminal.Core.Color as an
alternative to both Windows.UI.Color and uint32_t/COLORREF in the
TerminalCore, ...Control, ...SettingsModel and ...SettingsEditor layers.
M.T.C.Color is trivially convertible to/from til::color and therefore
to/from COLORREF, W.U.Color, and any other color representation we might
need².
I've replaced almost every use of W.U.Color and uint32_t-as-color in the
above layers, with minor exception¹.
The need for this work is twofold.
First: We cannot bear a dependency from TerminalCore (which should,
on paper, be Windows 7 compatible) on Windows.UI or any other WinRT
namespace.
This work removes one big dependency on Windows.UI, but it does not go
all the way.
Second: TerminalCore chose to communicate mostly in packed uint32s
(COLORREF), which was inherently lossy and dangerous.
¹ The UI layers (TerminalControl, TerminalApp) still use
Windows.UI.Color as they are intimately connected to the UWP XAML UI.
² In the future, we might even be able to *use* the alpha channel...
## PR Checklist
* [x] I ran into the need for this when I introduced cursor inversion
* [X] Fixes a longstanding itch
## Validation Steps Performed
Built and ran all tests for the impacted layers, even the local ones!
2021-03-30 22:15:49 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Windows::Foundation::IReference<Microsoft::Terminal::Core::Color>, TabColor, nullptr);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
|
|
|
// When set, StartingTabColor allows to create a terminal with a "sticky" tab color.
|
|
|
|
// This color is prioritized above the TabColor (that is usually initialized based on profile settings).
|
|
|
|
// Due to this prioritization, the tab color will be preserved upon settings reload
|
|
|
|
// (even if the profile's tab color gets altered or removed).
|
|
|
|
// This property is expected to be passed only once upon terminal creation.
|
|
|
|
// TODO: to ensure that this property is not populated during settings reload,
|
|
|
|
// we should consider moving this property to a separate interface,
|
|
|
|
// passed to the terminal only upon creation.
|
Introduce MS.Term.Core.Color to replace W.U.Color for Core/Control/TSM (#9658)
This pull request introduces Microsoft.Terminal.Core.Color as an
alternative to both Windows.UI.Color and uint32_t/COLORREF in the
TerminalCore, ...Control, ...SettingsModel and ...SettingsEditor layers.
M.T.C.Color is trivially convertible to/from til::color and therefore
to/from COLORREF, W.U.Color, and any other color representation we might
need².
I've replaced almost every use of W.U.Color and uint32_t-as-color in the
above layers, with minor exception¹.
The need for this work is twofold.
First: We cannot bear a dependency from TerminalCore (which should,
on paper, be Windows 7 compatible) on Windows.UI or any other WinRT
namespace.
This work removes one big dependency on Windows.UI, but it does not go
all the way.
Second: TerminalCore chose to communicate mostly in packed uint32s
(COLORREF), which was inherently lossy and dangerous.
¹ The UI layers (TerminalControl, TerminalApp) still use
Windows.UI.Color as they are intimately connected to the UWP XAML UI.
² In the future, we might even be able to *use* the alpha channel...
## PR Checklist
* [x] I ran into the need for this when I introduced cursor inversion
* [X] Fixes a longstanding itch
## Validation Steps Performed
Built and ran all tests for the impacted layers, even the local ones!
2021-03-30 22:15:49 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Windows::Foundation::IReference<Microsoft::Terminal::Core::Color>, StartingTabColor, nullptr);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
Add an ENUM setting for disabling rendering "intense" text as bold (#10759)
## Summary of the Pull Request
This adds a new setting `intenseTextStyle`. It's a per-appearance, control setting, defaulting to `"all"`.
* When set to `"all"` or `["bold", "bright"]`, then we'll render text as both **bold** and bright (1.10 behavior)
* When set to `"bold"`, `["bold"]`, we'll render text formatted with `^[[1m` as **bold**, but not bright
* When set to `"bright"`, `["bright"]`, we'll render text formatted with `^[[1m` as bright, but not bold. This is the pre 1.10 behavior
* When set to `"none"`, we won't do anything special for it at all.
## references
* I last did this in #10648. This time it's an enum, so we can add bright in the future. It's got positive wording this time.
* ~We will want to add `"bright"` as a value in the future, to disable the auto intense->bright conversion.~ I just did that now.
* #5682 is related
## PR Checklist
* [x] Closes #10576
* [x] I seriously don't think we have an issue for "disable intense is bright", but I'm not crazy, people wanted that, right? https://github.com/microsoft/terminal/issues/2916#issuecomment-544880423 was the closest
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/381
## Validation Steps Performed
<!-- 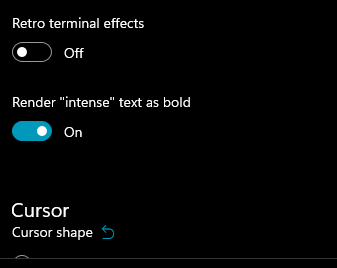 -->
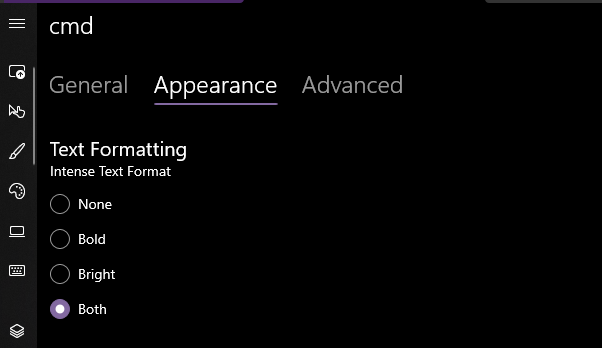
Yea that works. Printed some bold text, toggled it on, the text was no longer bold. hooray.
### EDIT, 10 Aug
```json
"intenseTextStyle": "none",
"intenseTextStyle": "bold",
"intenseTextStyle": "bright",
"intenseTextStyle": "all",
"intenseTextStyle": ["bold", "bright"],
```
all work now. Repro script:
```sh
printf "\e[1m[bold]\e[m[normal]\e[34m[blue]\e[1m[bold blue]\e[m\n"
```
2021-08-16 15:45:56 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, IntenseIsBright);
|
|
|
|
|
2021-10-08 00:43:17 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, AdjustIndistinguishableColors);
|
|
|
|
|
2021-03-16 00:15:25 +01:00
|
|
|
// ------------------------ End of Core Settings -----------------------
|
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, ProfileName);
|
2021-11-10 22:19:52 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, ProfileSource);
|
|
|
|
|
2021-03-16 00:15:25 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, UseAcrylic, false);
|
2021-09-20 19:08:13 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, double, Opacity, UseAcrylic() ? 0.5 : 1.0);
|
2021-03-16 00:15:25 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, Padding, DEFAULT_PADDING);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, FontFace, DEFAULT_FONT_FACE);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, int32_t, FontSize, DEFAULT_FONT_SIZE);
|
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, winrt::Windows::UI::Text::FontWeight, FontWeight);
|
2021-07-23 01:15:44 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, IFontAxesMap, FontAxes);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, IFontFeatureMap, FontFeatures);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
Persist window layout on window close (#10972)
This commit adds initial support for saving window layout on application
close.
Done:
- Add user setting for if tabs should be maintained.
- Added events to track the number of open windows for the monarch, and
then save if you are the last window closing.
- Saves layout when the user explicitly hits the "Close Window" button.
- If the user manually closed all of their tabs (through the tab x
button or through closing all panes on the tab) then remove any saved
state.
- Saves in the ApplicationState file a list of actions the terminal can
perform to restore its layout and the window size/position
information.
- This saves an action to focus the correct pane, but this won't
actually work without #10978. Note that if you have a pane zoomed, it
does still zoom the correct pane, but when you unzoom it will have a
different pane selected.
Todo:
- multiple windows? Right now it can only handle loading/saving one
window.
- PR #11083 will save multiple windows.
- This also sometimes runs into the existing bug where multiple tabs
appear to be focused on opening.
Next Steps:
- The business logic of when the save is triggered can be adjusted as
necessary.
- Right now I am taking the pragmatic approach and just saving the state
as an array of objects, but only ever populate it with 1, that way
saving multiple windows in the future could be added without breaking
schema compatibility. Selfishly I'm hoping that handling multiple
windows could be spun off into another pr/feature for now.
- One possible thing that can maybe be done is that the commandline can
be augmented with a "--saved ##" attribute that would load from the
nth saved state if it exists. e.g. if there are 3 saved windows, on
first load it can spawn three wt --saved {0,1,2} that would reopen the
windows? This way there also exists a way to load a copy of a previous
window (if it is in the saved state).
- Is the application state something that is planned to be public/user
editable? In theory the user could since it is just json, but I don't
know what it buys them over just modifying their settings and
startupActions.
Validation Steps Performed:
- The happy path: open terminal -> set setting to true -> close terminal
-> reopen and see tabs. Tested with powershell/cmd/wsl windows.
- That closing all panes/tabs on their own will remove the saved
session.
- Open multiple windows, close windows and confirm that the last window
closed saves its state.
The generated file stores a sequence of actions that will be executed to
restore the terminal to its saved form.
References #8324
This is also one of the items on microsoft/terminal#5000
Closes #766
2021-09-09 00:44:53 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Model::ColorScheme, AppliedColorScheme);
|
2021-03-16 00:15:25 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, BackgroundImage);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, double, BackgroundImageOpacity, 1.0);
|
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, winrt::Windows::UI::Xaml::Media::Stretch, BackgroundImageStretchMode, winrt::Windows::UI::Xaml::Media::Stretch::UniformToFill);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, winrt::Windows::UI::Xaml::HorizontalAlignment, BackgroundImageHorizontalAlignment, winrt::Windows::UI::Xaml::HorizontalAlignment::Center);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, winrt::Windows::UI::Xaml::VerticalAlignment, BackgroundImageVerticalAlignment, winrt::Windows::UI::Xaml::VerticalAlignment::Center);
|
|
|
|
|
2021-03-17 21:47:24 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Microsoft::Terminal::Control::IKeyBindings, KeyBindings, nullptr);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, Commandline);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, StartingDirectory);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, StartingTitle);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, SuppressApplicationTitle);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, EnvironmentVariables);
|
|
|
|
|
2021-03-17 21:47:24 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Microsoft::Terminal::Control::ScrollbarState, ScrollState, Microsoft::Terminal::Control::ScrollbarState::Visible);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
2021-03-17 21:47:24 +01:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, Microsoft::Terminal::Control::TextAntialiasingMode, AntialiasingMode, Microsoft::Terminal::Control::TextAntialiasingMode::Grayscale);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, RetroTerminalEffect, false);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, ForceFullRepaintRendering, false);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, SoftwareRendering, false);
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, ForceVTInput, false);
|
|
|
|
|
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, hstring, PixelShaderPath);
|
Add an ENUM setting for disabling rendering "intense" text as bold (#10759)
## Summary of the Pull Request
This adds a new setting `intenseTextStyle`. It's a per-appearance, control setting, defaulting to `"all"`.
* When set to `"all"` or `["bold", "bright"]`, then we'll render text as both **bold** and bright (1.10 behavior)
* When set to `"bold"`, `["bold"]`, we'll render text formatted with `^[[1m` as **bold**, but not bright
* When set to `"bright"`, `["bright"]`, we'll render text formatted with `^[[1m` as bright, but not bold. This is the pre 1.10 behavior
* When set to `"none"`, we won't do anything special for it at all.
## references
* I last did this in #10648. This time it's an enum, so we can add bright in the future. It's got positive wording this time.
* ~We will want to add `"bright"` as a value in the future, to disable the auto intense->bright conversion.~ I just did that now.
* #5682 is related
## PR Checklist
* [x] Closes #10576
* [x] I seriously don't think we have an issue for "disable intense is bright", but I'm not crazy, people wanted that, right? https://github.com/microsoft/terminal/issues/2916#issuecomment-544880423 was the closest
* [x] I work here
* [x] Tests added/passed
* [x] https://github.com/MicrosoftDocs/terminal/pull/381
## Validation Steps Performed
<!-- 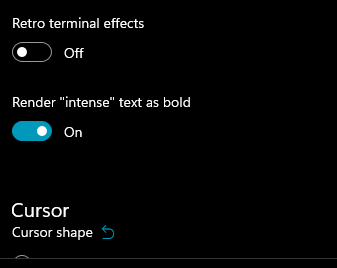 -->
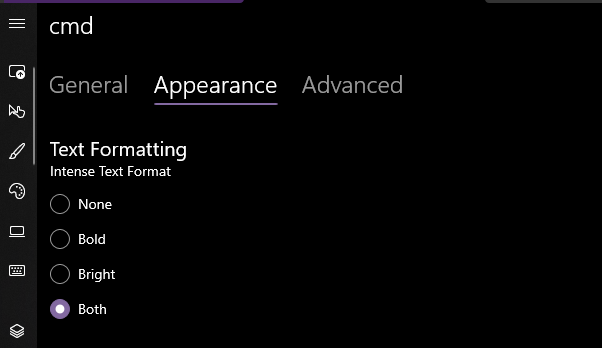
Yea that works. Printed some bold text, toggled it on, the text was no longer bold. hooray.
### EDIT, 10 Aug
```json
"intenseTextStyle": "none",
"intenseTextStyle": "bold",
"intenseTextStyle": "bright",
"intenseTextStyle": "all",
"intenseTextStyle": ["bold", "bright"],
```
all work now. Repro script:
```sh
printf "\e[1m[bold]\e[m[normal]\e[34m[blue]\e[1m[bold blue]\e[m\n"
```
2021-08-16 15:45:56 +02:00
|
|
|
INHERITABLE_SETTING(Model::TerminalSettings, bool, IntenseIsBold);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
|
|
|
private:
|
Introduce MS.Term.Core.Color to replace W.U.Color for Core/Control/TSM (#9658)
This pull request introduces Microsoft.Terminal.Core.Color as an
alternative to both Windows.UI.Color and uint32_t/COLORREF in the
TerminalCore, ...Control, ...SettingsModel and ...SettingsEditor layers.
M.T.C.Color is trivially convertible to/from til::color and therefore
to/from COLORREF, W.U.Color, and any other color representation we might
need².
I've replaced almost every use of W.U.Color and uint32_t-as-color in the
above layers, with minor exception¹.
The need for this work is twofold.
First: We cannot bear a dependency from TerminalCore (which should,
on paper, be Windows 7 compatible) on Windows.UI or any other WinRT
namespace.
This work removes one big dependency on Windows.UI, but it does not go
all the way.
Second: TerminalCore chose to communicate mostly in packed uint32s
(COLORREF), which was inherently lossy and dangerous.
¹ The UI layers (TerminalControl, TerminalApp) still use
Windows.UI.Color as they are intimately connected to the UWP XAML UI.
² In the future, we might even be able to *use* the alpha channel...
## PR Checklist
* [x] I ran into the need for this when I introduced cursor inversion
* [X] Fixes a longstanding itch
## Validation Steps Performed
Built and ran all tests for the impacted layers, even the local ones!
2021-03-30 22:15:49 +02:00
|
|
|
std::optional<std::array<Microsoft::Terminal::Core::Color, COLOR_TABLE_SIZE>> _ColorTable;
|
|
|
|
gsl::span<Microsoft::Terminal::Core::Color> _getColorTableImpl();
|
2021-04-09 00:46:16 +02:00
|
|
|
void _ApplyProfileSettings(const Model::Profile& profile);
|
|
|
|
|
2021-03-16 00:15:25 +01:00
|
|
|
void _ApplyGlobalSettings(const Model::GlobalAppSettings& globalSettings) noexcept;
|
2021-04-09 00:46:16 +02:00
|
|
|
void _ApplyAppearanceSettings(const Microsoft::Terminal::Settings::Model::IAppearanceConfig& appearance,
|
|
|
|
const Windows::Foundation::Collections::IMapView<hstring, Microsoft::Terminal::Settings::Model::ColorScheme>& schemes);
|
2021-03-16 00:15:25 +01:00
|
|
|
|
|
|
|
friend class SettingsModelLocalTests::TerminalSettingsTests;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
|
|
|
namespace winrt::Microsoft::Terminal::Settings::Model::factory_implementation
|
|
|
|
{
|
2021-04-21 22:35:06 +02:00
|
|
|
BASIC_FACTORY(TerminalSettingsCreateResult);
|
2021-03-16 00:15:25 +01:00
|
|
|
BASIC_FACTORY(TerminalSettings);
|
|
|
|
}
|